写一个Python五子棋代码
时间: 2023-09-26 10:07:04 浏览: 123
### 回答1:
我的Python五子棋代码如下:board = [[0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 1, 2, 0, 0, 0],
[0, 0, 0, 2, 1, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0]]def turn_valid(board, stone, x, y):
if board[x][y] != 0:
return False board[x][y] = stone #set the stone on board
for dx in range(-1, 2):
for dy in range(-1, 2):
if dx == 0 and dy == 0:
continue
if turn_around(board, stone, x, y, dx, dy):
board[x][y] = 0
return True
board[x][y] = 0
return Falsedef turn_around(board, stone, x, y, dx, dy):
count = 0
for i in range(1, 8):
nx = x + dx * i
ny = y + dy * i
if nx < 0 or ny < 0 or nx >= 8 or ny >= 8:
break
if board[nx][ny] == stone:
break
if board[nx][ny] == 0:
break
count += 1
if count == 0:
return False
for i in range(1, count+1):
nx = x + dx * i
ny = y + dy * i
board[nx][ny] = stone
return True
### 回答2:
下面是一个简单的Python五子棋代码实现:
```python
# 定义棋盘的大小
board_size = 15
# 定义两个玩家,1代表黑子,2代表白子
player1, player2 = 1, 2
# 初始化棋盘
board = [[0] * board_size for _ in range(board_size)]
# 判断当前位置是否可下棋
def is_valid_move(row, col):
return 0 <= row < board_size and 0 <= col < board_size and board[row][col] == 0
# 判断当前位置是否胜利
def is_win(row, col, player):
# 判断水平方向
count = 1
i = col - 1
while i >= 0 and board[row][i] == player:
count += 1
i -= 1
i = col + 1
while i < board_size and board[row][i] == player:
count += 1
i += 1
if count >= 5:
return True
# 判断垂直方向
count = 1
i = row - 1
while i >= 0 and board[i][col] == player:
count += 1
i -= 1
i = row + 1
while i < board_size and board[i][col] == player:
count += 1
i += 1
if count >= 5:
return True
# 判断正斜方向
count = 1
i, j = row - 1, col - 1
while i >= 0 and j >= 0 and board[i][j] == player:
count += 1
i -= 1
j -= 1
i, j = row + 1, col + 1
while i < board_size and j < board_size and board[i][j] == player:
count += 1
i += 1
j += 1
if count >= 5:
return True
# 判断反斜方向
count = 1
i, j = row - 1, col + 1
while i >= 0 and j < board_size and board[i][j] == player:
count += 1
i -= 1
j += 1
i, j = row + 1, col - 1
while i < board_size and j >= 0 and board[i][j] == player:
count += 1
i += 1
j -= 1
if count >= 5:
return True
return False
# 主游戏循环
current_player = player1
while True:
# 打印当前棋盘状态
for row in board:
print(row)
print("当前玩家:{}".format(current_player))
# 获取用户输入
row = int(input("请输入行号:"))
col = int(input("请输入列号:"))
# 判断输入是否合法
if is_valid_move(row, col):
# 下子
board[row][col] = current_player
# 判断是否胜利
if is_win(row, col, current_player):
print("玩家{}获胜!".format(current_player))
break
# 切换玩家
current_player = player2 if current_player == player1 else player1
else:
print("无效的输入,请重新输入。")
```
这个代码实现了一个简易的五子棋游戏,通过不断获取用户输入行号和列号来下棋,判断是否获胜,如果存在获胜的玩家,则游戏结束并打印胜利玩家的信息。
### 回答3:
五子棋是一种非常经典的棋类游戏,下面是一个简单的Python代码示例。
```python
# 五子棋游戏
# 初始化棋盘大小
board_size = 15
def create_board():
"""
创建棋盘
"""
board = [[" " for _ in range(board_size)] for _ in range(board_size)]
return board
def print_board(board):
"""
打印棋盘
"""
for row in board:
print("|".join(row))
def is_win(board, player, row, col):
"""
判断是否获胜
"""
directions = [(0, 1), (1, 0), (1, 1), (-1, 1)] # 四个方向:水平、垂直、正斜线、反斜线
for direction in directions:
dx, dy = direction
count = 0
# 统计在当前方向上相同棋子的个数
for i in range(-4, 5):
nx, ny = row + i * dx, col + i * dy
if 0 <= nx < board_size and 0 <= ny < board_size and board[nx][ny] == player:
count += 1
if count == 5: # 如果相同棋子个数达到5个,则获胜
return True
else:
count = 0
return False
def play():
"""
游戏主循环
"""
board = create_board()
player = "X"
while True:
print_board(board)
print("当前玩家:", player)
row = int(input("请输入行号(0-14):"))
col = int(input("请输入列号(0-14):"))
if board[row][col] != " ":
print("该位置已被占据,请重新输入")
continue
board[row][col] = player
if is_win(board, player, row, col):
print("玩家", player, "获胜!")
break
player = "O" if player == "X" else "X"
play()
```
这段代码实现了一个简单的五子棋游戏。玩家可以通过输入行号和列号来落子,判断胜利的依据是任意一方在水平、垂直、正斜线、反斜线方向上连续五个相同棋子。游戏通过打印棋盘的方式展示当前局面,当玩家获胜时,程序会给出相关提示并结束游戏。
这只是一个简单的示例,实际的五子棋游戏还可以加入更多的功能,如悔棋、多人对战等,可以根据需求进行相应的扩展。
阅读全文
相关推荐






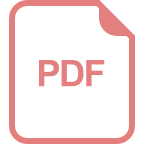
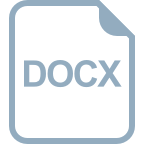
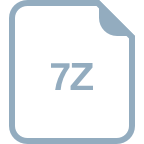