// 定义描述子类型及相关变量 typedef pcl::SHOT352 Descriptor; typedef pcl::PointCloud<Descriptor> DescriptorCloud; DescriptorCloud::Ptr descriptors_src(new DescriptorCloud); DescriptorCloud::Ptr descriptors_tgt(new DescriptorCloud); // 计算匹配点对及其汉明距离 pcl::Correspondences all_correspondences; pcl::registration::CorrespondenceEstimation<Descriptor, Descriptor> est; est.setInputSource(descriptors_src); est.setInputTarget(descriptors_tgt); est.determineCorrespondences(all_correspondences); // 将汉明距离按照从小到大的顺序排序 std::sort(all_correspondences.begin(), all_correspondences.end(), [](const pcl::Correspondence& a, const pcl::Correspondence& b) { return a.distance < b.distance; }); // 设置误差阈值,将小于阈值的匹配点对作为正确匹配点 const float kErrorThreshold = 20.0f; pcl::Correspondences correspondences; for (const auto& correspondence : all_correspondences) { if (correspondence.distance > kErrorThreshold) { correspondences.push_back(correspondence); } } // 逐步滤除误匹配点 while (true) { if (correspondences.empty()) { break; } const auto& correspondence = correspondences.front(); correspondences.erase(correspondences.begin()); // 检查该匹配点对是否已经被删除 if (correspondence.index_query >= static_cast<int>(cloud_src->size()) || correspondence.index_match >= static_cast<int>(cloud_tgt->size())) { continue; } // 将正确匹配的点对从点云中删除 cloud_src->erase(cloud_src->begin() + correspondence.index_query); cloud_tgt->erase(cloud_tgt->begin() + correspondence.index_match); // 重新计算匹配点对 est.setInputSource(descriptors_src); est.setInputTarget(descriptors_tgt); correspondences.clear(); est.determineCorrespondences(correspondences); }
时间: 2024-02-23 17:56:07 浏览: 50
这段代码是用于点云配准的过程中,通过计算描述子之间的汉明距离来筛选出正确的匹配点对,然后逐步滤除误匹配点的过程。
首先,通过定义描述子类型及相关变量,计算匹配点对及其汉明距离,将汉明距离按照从小到大的顺序排序,然后设置一个误差阈值,将小于阈值的匹配点对作为正确匹配点。接着,通过一个循环来逐步滤除误匹配点,每次循环中,都从正确匹配点对中取出一个点对,检查该点对是否已经被删除,如果没有被删除,则将其从点云中删除,然后重新计算匹配点对,直到没有误匹配点为止。
相关问题
pcl::PointCloud<pcl::SHOT352>::Ptr model_descriptors如果model_descriptors是布尔型描述子,要怎么改
如果 `model_descriptors` 变量是布尔型描述子,则需要将 `pcl::SHOT352` 类型的指针改为 `pcl::PointCloud<pcl::BINARY>::Ptr` 类型的指针,如下所示:
```
pcl::PointCloud<pcl::BINARY>::Ptr model_descriptors(new pcl::PointCloud<pcl::BINARY>());
```
然后,你需要在代码中将 `SHOT352` 相关的部分修改为 `BINARY` 相关的部分。例如,将 `pcl::SHOT352::Ptr` 修改为 `pcl::BINARY::Ptr`,将 `pcl::KdTreeFLANN<pcl::SHOT352>` 修改为 `pcl::KdTreeFLANN<pcl::BINARY>`,将 `pcl::CorrespondencesPtr` 修改为 `pcl::CorrespondencesPtr`,等等。最后,你需要根据实际数据特征来设置汉明距离的阈值,以便进行正确的匹配。
pcl::PointCloud<pcl::Histogram<64, pcl::po::common::IntensityAndXY> >::Ptr是对二维的布尔型描述子使用的吗
不完全正确。`pcl::PointCloud<pcl::Histogram<64, pcl::po::common::IntensityAndXY> >::Ptr`是用来表示64维的直方图描述子的PointCloud指针类型。其中,`pcl::Histogram<64, pcl::po::common::IntensityAndXY>`表示一个64维的直方图,其中每个直方图都是由`pcl::po::common::IntensityAndXY`类型的点云属性计算得出的。这里的`IntensityAndXY`表示点的强度和二维坐标,因此这个直方图的计算是基于点的位置和强度信息的。这种直方图描述子和布尔型描述子不同。因此,如果要对二维的布尔型描述子进行匹配,需要使用另外一种PointCloud类型来表示。
阅读全文
相关推荐
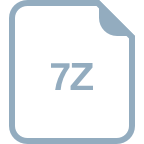
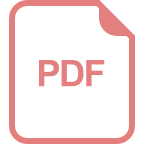
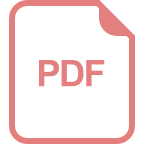













