写一个 stm32f103c8t6 串口接收16进制并控制灯打开的程序
时间: 2023-06-12 12:02:33 浏览: 260
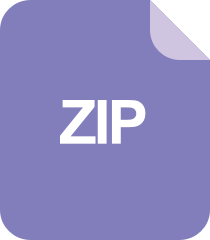
STM32F103C8T6配套程序源码-串口1通信实验.zip_KY8_STM32F103C8T6程序_stm32c8t6 JL

以下是一个简单的示例程序,可以实现在 STM32F103C8T6 上接收 16 进制数据,并根据接收到的数据控制 LED 灯的开关。
```c
#include "stm32f10x.h"
void USART1_Init(void);
void LED_Init(void);
void USART1_IRQHandler(void);
int main(void) {
uint8_t received_data;
LED_Init();
USART1_Init();
while (1) {
if (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == SET) {
received_data = USART_ReceiveData(USART1);
if (received_data == 0x01) {
GPIO_SetBits(GPIOC, GPIO_Pin_13);
} else if (received_data == 0x00) {
GPIO_ResetBits(GPIOC, GPIO_Pin_13);
}
}
}
}
void USART1_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
USART_Cmd(USART1, ENABLE);
}
void LED_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOC, &GPIO_InitStructure);
GPIO_ResetBits(GPIOC, GPIO_Pin_13);
}
void USART1_IRQHandler(void) {
if (USART_GetITStatus(USART1, USART_IT_RXNE) == SET) {
USART_ClearITPendingBit(USART1, USART_IT_RXNE);
}
}
```
这个程序的主要思路是通过 USART1 接收 16 进制数据,并根据接收到的数据控制 LED 灯的开关。在程序中,USART1_Init() 函数用于初始化 USART1,LED_Init() 函数用于初始化 LED 灯,USART1_IRQHandler() 函数用于处理 USART1 中断。
在 main() 函数中,我们使用了一个无限循环,不断地读取 USART1 接收到的数据,如果接收到的是 0x01,则点亮 LED 灯;如果接收到的是 0x00,则关闭 LED 灯。注意,这里只是一个简单的示例程序,实际使用时需要根据自己的需求进行修改。
阅读全文
相关推荐
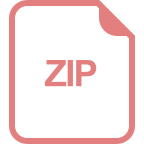





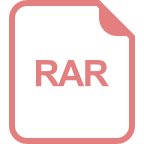
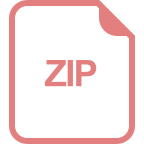
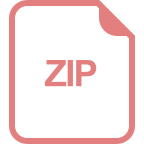
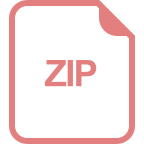
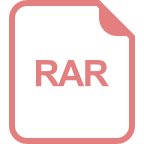
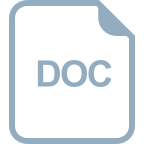
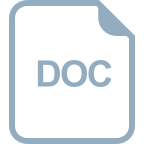
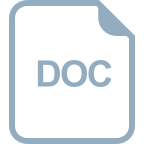
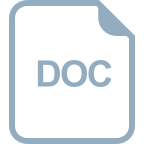
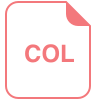
