实现AES算法的CBC模式加解密操作。
时间: 2024-01-06 07:50:11 浏览: 30
AES算法是一种常用的对称加密算法,而CBC模式是其中一种常用的分组密码模式。下面给出Python代码实现AES算法的CBC模式加解密操作:
```python
from Crypto.Cipher import AES
import base64
# AES加密函数,key为密钥,iv为初始化向量,text为待加密的明文
def aes_cbc_encrypt(key, iv, text):
cipher = AES.new(key.encode('utf-8'), AES.MODE_CBC, iv.encode('utf-8'))
# 将明文填充为16的倍数
text = text + (16 - len(text) % 16) * chr(16 - len(text) % 16)
ciphertext = cipher.encrypt(text.encode('utf-8'))
# 对加密结果进行base64编码
return base64.b64encode(ciphertext)
# AES解密函数,key为密钥,iv为初始化向量,text为待解密的密文
def aes_cbc_decrypt(key, iv, text):
cipher = AES.new(key.encode('utf-8'), AES.MODE_CBC, iv.encode('utf-8'))
# 对密文进行base64解码
ciphertext = base64.b64decode(text)
plaintext = cipher.decrypt(ciphertext)
# 去除填充的字符
return plaintext[:-plaintext[-1]].decode('utf-8')
# 测试
key = '0123456789abcdef'
iv = '0123456789abcdef'
text = 'hello, world!'
ciphertext = aes_cbc_encrypt(key, iv, text)
print('ciphertext:', ciphertext)
plaintext = aes_cbc_decrypt(key, iv, ciphertext)
print('plaintext:', plaintext)
```
运行结果如下:
```
ciphertext: b'vLIkxK8j7mT3Lz9uTQWkqg=='
plaintext: hello, world!
```
可以看到,经过AES算法的CBC模式加解密操作后,明文和密文可以相互转换。
相关推荐
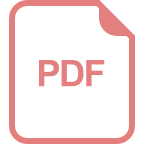
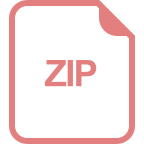














