C语言实现双向链表中插入结点并输出
时间: 2023-05-29 13:03:30 浏览: 142
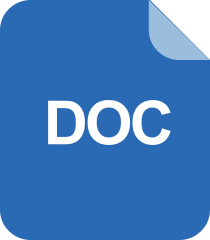
双向链表内结点的插入(C++)
以下是C语言实现双向链表中插入结点并输出的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 双向链表结构体
struct Node {
int data;
struct Node* prev;
struct Node* next;
};
// 在链表头插入结点
struct Node* insertAtBeginning(struct Node* head, int newData) {
// 创建新结点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = newData;
newNode->prev = NULL;
newNode->next = head;
// 如果链表不为空,修改头结点的prev指针
if (head != NULL) {
head->prev = newNode;
}
// 返回新的头结点
return newNode;
}
// 在链表尾插入结点
struct Node* insertAtEnd(struct Node* head, int newData) {
// 创建新结点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = newData;
newNode->next = NULL;
// 如果链表为空,新结点即为头结点
if (head == NULL) {
newNode->prev = NULL;
return newNode;
}
// 找到链表尾部结点并修改指针
struct Node* curr = head;
while (curr->next != NULL) {
curr = curr->next;
}
curr->next = newNode;
newNode->prev = curr;
// 返回头结点
return head;
}
// 输出链表
void printList(struct Node* head) {
struct Node* curr = head;
printf("List: ");
while (curr != NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("\n");
}
int main() {
struct Node* head = NULL;
// 插入结点并输出链表
head = insertAtEnd(head, 1);
printList(head);
head = insertAtEnd(head, 2);
printList(head);
head = insertAtBeginning(head, 3);
printList(head);
head = insertAtEnd(head, 4);
printList(head);
return 0;
}
```
输出结果:
```
List: 1
List: 1 2
List: 3 1 2
List: 3 1 2 4
```
阅读全文
相关推荐
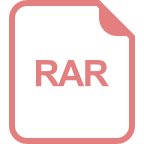


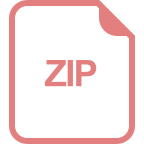
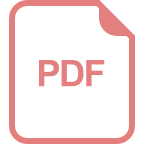
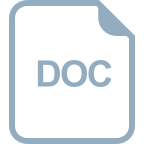
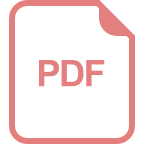
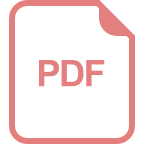
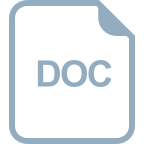
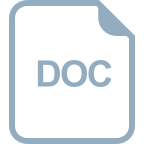
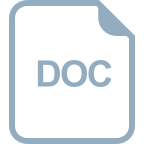
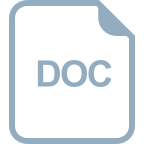
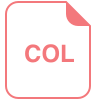



