用C语言写条件为. 双向链表中插入结点并输出 【问题描述】 仿照有头结点单链表的存储和基本操作,设计双向链表-非循环链表(初始化、插入、输出)。 对带头结点的双向链表,实现数据插入。 【输入形式】 第一行输入一个N(N大于等于1,小于1000); 第二行输入N个整数,以空格作为分隔,创建长度为N的双向链表,为双向输出方便,需保留头尾指针; 第三行输入pos和e,以空格分隔,分别表示插入位置和插入元素的值。 【输出形式】 若插入位置合法,插入成功,则输出: 第一行从头开始顺向输出双向链表中元素,用空格分隔; 第二行从尾结点开始逆向输出双向链表中元素,用空格分隔。 若插入位置不合法,例如插入位置<1或超出链表范围,则输出error。
时间: 2023-05-29 11:05:50 浏览: 45
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *prev;
struct Node *next;
} Node;
void initList(Node **head, Node **tail) {
*head = (Node *)malloc(sizeof(Node));
*tail = (Node *)malloc(sizeof(Node));
(*head)->prev = NULL;
(*head)->next = *tail;
(*tail)->prev = *head;
(*tail)->next = NULL;
}
void insert(Node *head, int pos, int e) {
Node *p = head;
int i;
for (i = 0; i < pos - 1 && p->next != NULL; i++) {
p = p->next;
}
if (i != pos - 1) {
printf("error\n");
return;
}
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = e;
newNode->prev = p;
newNode->next = p->next;
p->next->prev = newNode;
p->next = newNode;
}
void printList(Node *head, Node *tail) {
Node *p = head->next;
while (p != tail) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
p = tail->prev;
while (p != head) {
printf("%d ", p->data);
p = p->prev;
}
printf("\n");
}
int main() {
int n, pos, e;
Node *head, *tail;
scanf("%d", &n);
initList(&head, &tail);
for (int i = 0; i < n; i++) {
int data;
scanf("%d", &data);
insert(tail->prev, 1, data);
}
scanf("%d%d", &pos, &e);
insert(head, pos, e);
printList(head, tail);
return 0;
}
```
相关推荐
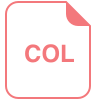
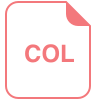
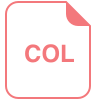
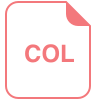
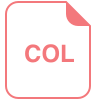









