C语言设计带头结点静态双向循环链表
时间: 2024-01-25 10:43:24 浏览: 96
的插入操作时,应该如何实现?
对于带头结点的静态双向循环链表,插入操作分为两种情况:
1. 在表头插入:新节点的后继指针指向原头节点,原头节点的前驱指针指向新节点,新节点的前驱指针指向头结点,头结点的后继指针指向新结点。
2. 在表尾插入:新节点的前驱指针指向原尾节点,原尾节点的后继指针指向新节点,新节点的后继指针指向头结点,头结点的前驱指针指向新节点。
下面是示例代码:
```
typedef struct Node{
int data;
int prev;
int next;
} Node;
Node nodeList[MAX_SIZE];
int head, tail; //头尾指针
//初始化双向循环链表
void initList(){
nodeList[head].prev = tail;
nodeList[head].next = tail;
nodeList[tail].prev = head;
nodeList[tail].next = head;
}
//在表头插入节点
void insertNodeAtHead(int data){
int newNodeIndex = tail + 1;
nodeList[newNodeIndex].data = data;
nodeList[newNodeIndex].prev = head;
nodeList[newNodeIndex].next = nodeList[head].next;
nodeList[nodeList[head].next].prev = newNodeIndex;
nodeList[head].next = newNodeIndex;
}
//在表尾插入节点
void insertNodeAtTail(int data){
int newNodeIndex = tail + 1;
nodeList[newNodeIndex].data = data;
nodeList[newNodeIndex].prev = nodeList[tail].prev;
nodeList[newNodeIndex].next = head;
nodeList[nodeList[tail].prev].next = newNodeIndex;
nodeList[head].prev = newNodeIndex;
}
```
阅读全文
相关推荐
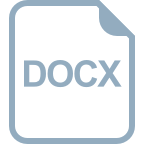

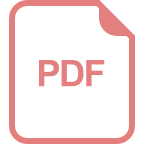
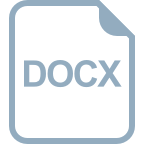
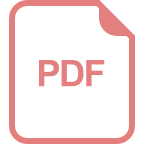
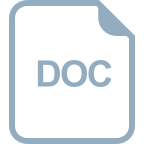
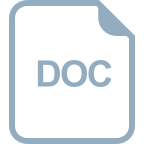
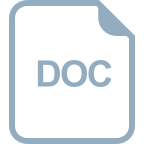
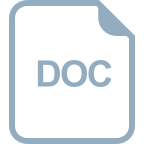






