用Python写一个贪吃蛇游戏
时间: 2024-03-06 19:03:03 浏览: 33
好的,以下是用Python实现贪吃蛇游戏的代码:
```python
import pygame, random
# 初始化Pygame
pygame.init()
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义屏幕大小
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("贪吃蛇游戏")
# 定义蛇的初始位置
snake_pos = [100, 50]
snake_body = [[100, 50], [90, 50], [80, 50]]
# 定义食物的初始位置
food_pos = [random.randrange(1, (SCREEN_WIDTH//10)) * 10, random.randrange(1, (SCREEN_HEIGHT//10)) * 10]
food_spawned = True
# 定义蛇移动的初始方向
direction = "RIGHT"
change_to = direction
# 定义游戏结束的标志变量
game_over = False
# 定义游戏时钟
clock = pygame.time.Clock()
# 定义字体
font_style = pygame.font.SysFont(None, 50)
# 定义游戏结束的函数
def game_over_screen():
game_over_msg = font_style.render("游戏结束,按Q退出,按C重新开始", True, RED)
game_over_rect = game_over_msg.get_rect()
game_over_rect.center = (SCREEN_WIDTH//2, SCREEN_HEIGHT//2)
screen.blit(game_over_msg, game_over_rect)
pygame.display.update()
pygame.time.wait(500)
while True:
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
pygame.quit()
quit()
elif event.key == pygame.K_c:
game_loop()
# 定义游戏循环函数
def game_loop():
# 使用全局变量
global direction, change_to, snake_pos, snake_body, food_pos, food_spawned, game_over
# 循环标志变量
game_exit = False
while not game_exit:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_exit = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT and direction != "RIGHT":
change_to = "LEFT"
elif event.key == pygame.K_RIGHT and direction != "LEFT":
change_to = "RIGHT"
elif event.key == pygame.K_UP and direction != "DOWN":
change_to = "UP"
elif event.key == pygame.K_DOWN and direction != "UP":
change_to = "DOWN"
elif event.key == pygame.K_ESCAPE:
pygame.quit()
quit()
# 检查方向是否相反
if change_to == "LEFT" and direction != "RIGHT":
direction = "LEFT"
elif change_to == "RIGHT" and direction != "LEFT":
direction = "RIGHT"
elif change_to == "UP" and direction != "DOWN":
direction = "UP"
elif change_to == "DOWN" and direction != "UP":
direction = "DOWN"
# 根据方向移动蛇的位置
if direction == "LEFT":
snake_pos[0] -= 10
elif direction == "RIGHT":
snake_pos[0] += 10
elif direction == "UP":
snake_pos[1] -= 10
elif direction == "DOWN":
snake_pos[1] += 10
# 更新蛇身体的位置
snake_body.insert(0, list(snake_pos))
if snake_pos == food_pos:
food_spawned = False
else:
snake_body.pop()
# 生成新的食物
if not food_spawned:
food_pos = [random.randrange(1, (SCREEN_WIDTH//10)) * 10, random.randrange(1, (SCREEN_HEIGHT//10)) * 10]
food_spawned = True
# 绘制游戏窗口
screen.fill(BLACK)
for pos in snake_body:
pygame.draw.rect(screen, GREEN, pygame.Rect(pos[0], pos[1], 10, 10))
pygame.draw.rect(screen, WHITE, pygame.Rect(food_pos[0], food_pos[1], 10, 10))
# 检查是否碰到边界
if snake_pos[0] < 0 or snake_pos[0] > SCREEN_WIDTH-10 or snake_pos[1] < 0 or snake_pos[1] > SCREEN_HEIGHT-10:
game_over = True
game_over_screen()
# 检查是否碰到自己
for block in snake_body[1:]:
if snake_pos == block:
game_over = True
game_over_screen()
# 更新游戏窗口
pygame.display.update()
# 设置游戏时钟
clock.tick(15)
# 退出Pygame
pygame.quit()
# 开始游戏循环
game_loop()
```
以上代码实现了一个简单的贪吃蛇游戏,你可以在命令行中运行这个Python文件,即可开始游戏。注意,这个游戏需要Pygame库的支持,如果你没有安装这个库,可以使用pip安装,命令如下:
```
pip install pygame
```
相关推荐
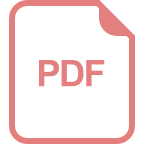
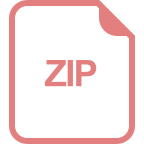
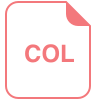
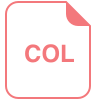
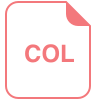
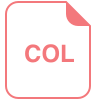
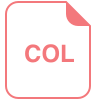



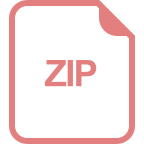
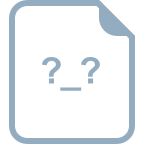
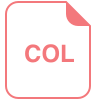
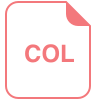
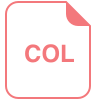
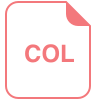