spring boot+vue断点续传 代码实现
时间: 2023-07-31 21:08:31 浏览: 52
以下是一个简单的 Spring Boot + Vue 的文件上传和断点续传的示例代码,供参考:
前端代码:
```vue
<template>
<div>
<!-- 选择文件按钮 -->
<input type="file" ref="fileInput" @change="selectFile">
<!-- 上传按钮 -->
<button @click="upload">上传</button>
<!-- 进度条 -->
<div>
<div :style="{ width: progress + '%' }"></div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
file: null, // 选择的文件
progress: 0, // 上传进度
uploadedChunks: [], // 已上传的分块
chunkSize: 1024 * 1024, // 分块大小
};
},
methods: {
// 选择文件
selectFile() {
this.file = this.$refs.fileInput.files[0];
},
// 上传文件
async upload() {
const totalChunks = Math.ceil(this.file.size / this.chunkSize);
for (let i = 0; i < totalChunks; i++) {
const start = i * this.chunkSize;
const end = Math.min(start + this.chunkSize, this.file.size);
const chunk = this.file.slice(start, end);
// 如果该分块已上传,则跳过
if (this.uploadedChunks.includes(i)) {
continue;
}
// 构造 FormData 对象
const formData = new FormData();
formData.append('file', chunk);
formData.append('chunk', i);
formData.append('totalChunks', totalChunks);
// 发送分块上传请求
const res = await this.$http.post('/upload', formData, {
headers: { 'Content-Type': 'multipart/form-data' },
onUploadProgress: (progressEvent) => {
// 更新上传进度
this.progress = Math.round((start + progressEvent.loaded) / this.file.size * 100);
},
});
// 如果上传成功,则记录已上传的分块
if (res.data) {
this.uploadedChunks.push(i);
}
}
// 如果所有分块都上传成功,则发送合并请求
if (this.uploadedChunks.length === totalChunks) {
await this.$http.post('/merge', { filename: this.file.name });
}
},
},
};
</script>
```
后端代码:
```java
@RestController
public class FileController {
private final String uploadDir = "/tmp/upload/"; // 上传文件存储路径
private final String mergeDir = "/tmp/merge/"; // 合并文件存储路径
private final int chunkSize = 1024 * 1024; // 分块大小
// 分块上传接口
@PostMapping("/upload")
public boolean upload(@RequestParam("file") MultipartFile file,
@RequestParam("chunk") int chunk,
@RequestParam("totalChunks") int totalChunks) throws IOException {
// 构造上传文件的路径和文件名
String filename = file.getOriginalFilename();
String filepath = uploadDir + filename + "_" + chunk;
// 如果该分块已上传,则直接返回上传成功
if (new File(filepath).exists()) {
return true;
}
// 将分块保存到临时文件中
file.transferTo(new File(filepath));
// 如果所有分块都已上传,则返回上传成功
if (new File(uploadDir).list().length == totalChunks) {
return true;
}
return false;
}
// 合并文件接口
@PostMapping("/merge")
public void merge(@RequestParam("filename") String filename) throws IOException {
// 构造合并后的文件路径和文件名
String filepath = mergeDir + filename;
File mergedFile = new File(filepath);
// 如果合并后的文件已存在,则删除原文件
if (mergedFile.exists()) {
mergedFile.delete();
}
// 将所有分块合并成一个文件
for (int i = 0; i < Integer.MAX_VALUE; i++) {
String chunkPath = uploadDir + filename + "_" + i;
File chunkFile = new File(chunkPath);
// 如果该分块不存在,则说明所有分块都已合并完成
if (!chunkFile.exists()) {
break;
}
// 将该分块添加到合并文件中
Files.write(mergedFile.toPath(), Files.readAllBytes(chunkFile.toPath()), StandardOpenOption.APPEND);
}
// 删除上传文件和分块文件
FileUtils.deleteDirectory(new File(uploadDir));
FileUtils.deleteDirectory(new File(mergeDir));
}
}
```
以上代码实现了一个简单的文件上传和断点续传的示例,其中前端使用 Vue 实现了文件选择和上传操作,后端使用 Spring Boot 实现了分块上传和合并操作。需要注意的是,这只是一个简单的示例,实际应用中需要根据具体的需求和问题来选择不同的技术和组件,并合理地设置参数和配置。
相关推荐
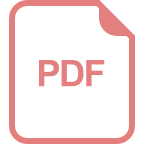














