TVM的schedule能否同时计算四个矩阵乘法
时间: 2024-03-05 14:48:42 浏览: 138
可以的。在TVM中,您可以使用schedule对多个算子进行优化,包括同时计算多个矩阵乘法。具体来说,您可以使用TVM的API创建多个矩阵乘法算子,并将它们组合成一个复合算子。然后,您可以使用TVM的schedule API对这些算子进行调度,以实现并行计算。
以下是一个示例代码,其中包含四个矩阵相乘的算子,它们被组合成一个复合算子,并使用TVM的schedule API对其进行并行化:
```python
import tvm
import numpy as np
# Define the shape of the matrices
n, m, l, p = 10, 20, 30, 40
# Define the input placeholders
A = tvm.te.placeholder((n, m), name='A')
B = tvm.te.placeholder((m, l), name='B')
C = tvm.te.placeholder((l, p), name='C')
D = tvm.te.placeholder((p, n), name='D')
# Define the intermediate tensors
k = tvm.te.reduce_axis((0, m), name='k')
E = tvm.te.compute((n, l), lambda i, j: tvm.te.sum(A[i, k] * B[k, j], axis=k), name='E')
l = tvm.te.reduce_axis((0, l), name='l')
F = tvm.te.compute((n, p), lambda i, j: tvm.te.sum(E[i, l] * C[l, j], axis=l), name='F')
o = tvm.te.reduce_axis((0, p), name='o')
G = tvm.te.compute((m, n), lambda i, j: tvm.te.sum(D[o, i] * F[j, o], axis=o), name='G')
# Compile the computation into a function
s = tvm.te.create_schedule(G.op)
xo, xi = s[G].split(G.op.axis[1], factor=32)
s[G].reorder(G.op.reduce_axis[0], xo, xi, G.op.axis[0])
s[G].parallel(xo)
s[F].parallel(F.op.axis[0])
s[E].parallel(E.op.axis[0])
s[C].parallel(C.op.axis[1])
s[B].parallel(B.op.axis[1])
s[A].parallel(A.op.axis[0])
func = tvm.build(s, [A, B, C, D, G])
# Generate some random input data
a = np.random.rand(n, m).astype(np.float32)
b = np.random.rand(m, l).astype(np.float32)
c = np.random.rand(l, p).astype(np.float32)
d = np.random.rand(p, n).astype(np.float32)
# Execute the function with the input data
g = np.empty((m, n), dtype=np.float32)
func(tvm.nd.array(a), tvm.nd.array(b), tvm.nd.array(c), tvm.nd.array(d), tvm.nd.array(g))
# Verify the result
np.testing.assert_allclose(g, np.dot(d, np.dot(c, np.dot(a, b))))
```
在上面的代码中,我们使用schedule API对四个矩阵相乘的算子进行了调度。我们使用了TVM的API定义了四个矩阵相乘的算子,并将它们组合成一个复合算子。然后,我们使用TVM的schedule API对这些算子进行并行化。具体来说,我们使用了split、reorder和parallel等API对这些算子进行调度,以实现并行计算。最后,我们使用TVM的API将这个复合算子编译成一个可执行函数,并将输入数据传递给它。最后,我们验证了输出结果是否正确。
阅读全文
相关推荐
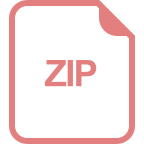















