如何用AutoTVM进行矩阵乘法运算
时间: 2024-06-07 16:11:45 浏览: 12
AutoTVM 是一个自动调优的深度学习加速框架,它可以根据硬件配置和算法特性自动选择最佳的计算图和调度策略,进而优化深度学习模型的性能。下面是如何用 AutoTVM 进行矩阵乘法运算的简单示例:
首先,我们需要安装 AutoTVM 和 tvm 等相关依赖:
```
pip install --user tvm
pip install --user psutil xgboost tornado cloudpickle
```
然后,我们定义一个简单的矩阵乘法函数,用于测试:
```python
import tvm
from tvm import te
def matmul(n):
A = te.placeholder((n, n), name='A')
B = te.placeholder((n, n), name='B')
k = te.reduce_axis((0, n), name='k')
C = te.compute((n, n), lambda i, j: te.sum(A[i, k] * B[k, j], axis=k), name='C')
s = te.create_schedule(C.op)
return s, [A, B, C]
```
接着,我们使用 AutoTVM 进行自动调优:
```python
import autotvm
target = "llvm"
n = 1024
log_file = "matmul.log"
# define a tuning task
task = autotvm.task.create("matmul", args=(n,), target=target)
# define search space
search_space = autotvm.get_config("matmul.tune")
# run tuning
tuner = autotvm.tuner.XGBTuner(task)
tuner.tune(n_trial=1000, early_stopping=200, measure_option=autotvm.measure_option(builder='local', runner=autotvm.LocalRunner(timeout=10)), callbacks=[autotvm.callback.log_to_file(log_file)])
```
最后,我们可以使用调优结果来运行矩阵乘法函数:
```python
# load tuning results
with autotvm.apply_history_best(log_file):
with tvm.target.create(target):
s, args = matmul(n)
func = tvm.build(s, args)
# run the function
ctx = tvm.cpu()
a_np = np.random.rand(n, n).astype(np.float32)
b_np = np.random.rand(n, n).astype(np.float32)
c_np = np.zeros((n, n)).astype(np.float32)
a_tvm = tvm.nd.array(a_np, ctx)
b_tvm = tvm.nd.array(b_np, ctx)
c_tvm = tvm.nd.array(c_np, ctx)
func(a_tvm, b_tvm, c_tvm)
tvm.testing.assert_allclose(c_np, c_tvm.asnumpy(), rtol=1e-4)
```
相关推荐
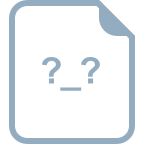














