ef catmap(frame, n): s = frame.shape pic = np.zeros(s, np.uint8) A = np.array([[2, 1], [1, 1]]) ind = A @ np.indices(s).reshape(2, -1) % (np.array(s)[:, None]) pic[ind[0], ind[1]] = frame.reshape(-1) return pic
时间: 2024-04-03 15:35:29 浏览: 109
这段代码实现了一个Cat映射(Cat Map)的函数,它将输入的二维数组(图像)做一次Cat映射,并返回映射后的结果。
具体来说,这个Cat映射是由矩阵A = [[2, 1], [1, 1]] 定义的,而映射的过程则是通过将输入的二维数组看作一个向量,然后将其通过矩阵A进行线性变换,再对变换后的坐标取模得到的。最后,将变换后的坐标映射回原图像坐标系中,并将原图像中的像素按照新的坐标位置赋值到新的图像中。
这个函数的输入参数frame是一个二维数组,表示输入的图像,而n则是一个整数,表示Cat映射的次数。由于Cat映射是一个可逆的映射,因此多次映射可以得到更加复杂的变换效果。
相关问题
Map<String,VideoSplitDataFiles> catMap = videoSplitDataFilesListAll.stream().collect(Collectors.toMap(VideoSplitDataFiles::getUUID, Function.identity()));
根据你提供的代码,你正在使用Java 8的Stream API将`videoSplitDataFilesListAll`列表转换为一个映射(Map),其中键是`VideoSplitDataFiles`对象的UUID,值是对应的`VideoSplitDataFiles`对象本身。
这段代码可能会抛出`java.lang.IllegalStateException: Duplicate key`异常,如果`videoSplitDataFilesListAll`列表中存在具有相同UUID的两个或多个对象。这意味着在转换为映射时会出现重复的键。
要解决这个问题,你可以在使用`toMap()`方法之前确保列表中没有重复的UUID。一种简单的方法是使用`distinct()`方法来去除重复的对象:
```java
Map<String, VideoSplitDataFiles> catMap = videoSplitDataFilesListAll.stream()
.distinct()
.collect(Collectors.toMap(VideoSplitDataFiles::getUUID, Function.identity()));
```
如果你希望处理重复键而不是抛出异常,你可以使用第三个参数来指定一个合并函数,它将决定如何处理重复键。例如,你可以选择保留第一个键对应的值:
```java
Map<String, VideoSplitDataFiles> catMap = videoSplitDataFilesListAll.stream()
.collect(Collectors.toMap(VideoSplitDataFiles::getUUID, Function.identity(), (existing, duplicate) -> existing));
```
这样,当遇到重复键时,将保留原始值并丢弃重复值。
请确保在使用这段代码之前,`videoSplitDataFilesListAll`列表中的对象具有正确的UUID,并且你已经实现了适当的`equals()`和`hashCode()`方法,以便在比较和识别重复对象时能够正常工作。
def catmap(img, rounds): # 将图像转换为numpy数组 img_np = np.array(img) # 获取图像的宽度和高度 width, height = img.size # 设置Arnold置乱的参数 a = 3 b = 5 # 迭代进行Arnold置乱 for i in range(rounds): new_img = np.zeros_like(img_np) for x in range(width): for y in range(height): new_x = (a * x + b * y) % width new_y = (b * x + (a * b + 1) * y) % height new_img[new_x, new_y] = img_np[x, y] img_np = new_img return Image.fromarray(img_np) scrambled_image = catmap(image, 100) si = scrambled_image si = 255.0 * (si - si.min()) / (si.max() - si.min()) si = np.array(si, np.int) cv2.imwrite('scrambled_image.png', si) plt.imshow(scrambled_image, cmap='gray')、
这段代码中,catmap函数是将输入的图像进行Arnold置乱操作,并返回置乱后的图像。然后,通过调用catmap函数,将输入的image图像进行100次Arnold置乱,得到置乱后的图像scrambled_image。接下来,通过将每个像素点的值进行归一化和类型转换,将scrambled_image保存为png格式的图像文件。最后,使用imshow函数显示置乱后的图像。
这段代码中,有一些需要注意的地方。首先,在catmap函数中,return语句的位置应该在for循环的外面,否则每次迭代只会执行一次就直接返回结果了,无法达到指定的迭代次数。其次,在保存图像文件时,需要设置像素值的类型为整型,否则保存的图像会出现错误。最后,在显示图像时,使用imshow函数需要指定图像的颜色映射cmap为gray,否则会显示为彩色图像。
下面是修改后的代码:
```python
def catmap(img, rounds):
# 将图像转换为numpy数组
img_np = np.array(img)
# 获取图像的宽度和高度
width, height = img.size
# 设置Arnold置乱的参数
a = 3
b = 5
# 迭代进行Arnold置乱
for i in range(rounds):
new_img = np.zeros_like(img_np)
for x in range(width):
for y in range(height):
new_x = (a * x + b * y) % width
new_y = (b * x + (a * b + 1) * y) % height
new_img[new_x, new_y] = img_np[x, y]
img_np = new_img
return Image.fromarray(img_np)
# 对图像进行100次Arnold置乱
scrambled_image = catmap(image, 100)
# 将像素值归一化并转换为整型
si = 255.0 * (scrambled_image - scrambled_image.min()) / (scrambled_image.max() - scrambled_image.min())
si = np.array(si, np.int)
# 保存置乱后的图像为png格式
cv2.imwrite('scrambled_image.png', si)
# 显示置乱后的图像
plt.imshow(scrambled_image, cmap='gray')
```
阅读全文
相关推荐
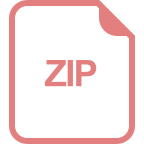
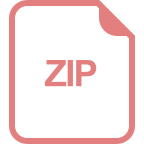
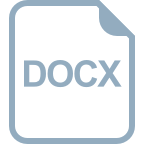



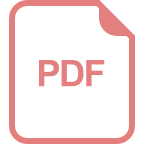
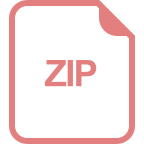
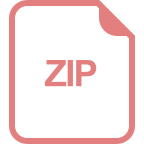
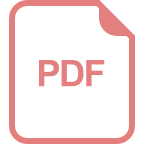