用c语言代码写九九乘法表
时间: 2023-05-20 15:04:58 浏览: 118
Sure, here's a C program to print the multiplication table of 1 to 9:
```
#include <stdio.h>
int main() {
int i, j;
for (i = 1; i <= 9; i++) {
for (j = 1; j <= i; j++) {
printf("%d*%d=%d\t", j, i, i*j);
}
printf("\n");
}
return 0;
}
```
This program uses nested loops to print the multiplication table. The outer loop iterates from 1 to 9, and the inner loop iterates from 1 to the current value of the outer loop variable. Inside the inner loop, we print the product of the two loop variables, along with some formatting characters to make the output look nice.
相关问题
用C语言写:九九乘法表
好的,以下是在C语言中打印九九乘法表的代码:
#include <stdio.h>
int main()
{
int i, j;
for(i=1; i<=9; i++)
{
for(j=1; j<=i; j++)
{
printf("%d*%d=%d ", j, i, i*j);
}
printf("\n");
}
return 0;
}
运行该程序,将会得到如下输出的九九乘法表:
1*1=1
1*2=2 2*2=4
1*3=3 2*3=6 3*3=9
1*4=4 2*4=8 3*4=12 4*4=16
1*5=5 2*5=10 3*5=15 4*5=20 5*5=25
1*6=6 2*6=12 3*6=18 4*6=24 5*6=30 6*6=36
1*7=7 2*7=14 3*7=21 4*7=28 5*7=35 6*7=42 7*7=49
1*8=8 2*8=16 3*8=24 4*8=32 5*8=40 6*8=48 7*8=56 8*8=64
1*9=9 2*9=18 3*9=27 4*9=36 5*9=45 6*9=54 7*9=63 8*9=72 9*9=81
用c语言写个九九乘法表
九九乘法表是由2个数字相乘得来的,而且每一列的第一个数字都是一样的,每一行的第2个数字都是一样的。我们可以用两个for循环来控制每一行每一列的内容,每一行的内容我们可以用for循环来打印。以下是用C语言写九九乘法表的代码:
```
#include <stdio.h>
int main() {
int i, j;
for (i = 1; i <= 9; i++) {
for (j = 1; j <= i; j++) {
printf("%d*%d=%d\t", j, i, i*j);
}
printf("\n");
}
return 0;
}
```
这段代码中,外层循环控制行数,内层循环控制列数,每次输出一个乘法表达式并用制表符隔开,最后换行。运行代码后,即可输出九九乘法表。
阅读全文
相关推荐
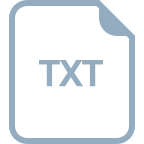
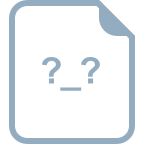
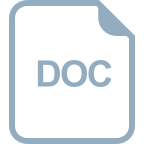










