使用java定义一个表示颜色的枚举类型 Color,该枚举类型有3个常量red,yellow,blue。 声明两个Color枚举变量x和y,为x和y变量赋值任一枚举常量,输出x和y的值。
时间: 2023-04-01 12:00:57 浏览: 121
Color 枚举类型的定义如下:
enum Color {
red, yellow, blue
}
声明两个 Color 枚举变量 x 和 y,并为它们赋值任意枚举常量,可以使用以下代码:
Color x = Color.red;
Color y = Color.yellow;
输出 x 和 y 的值,可以使用以下代码:
System.out.println("x 的值为:" + x);
System.out.println("y 的值为:" + y);
输出结果为:
x 的值为:red
y 的值为:yellow
相关问题
我有多个枚举类型,但是我如何通过一种方式获取枚举类型的最大值
如果你有多个枚举类型,可以使用一个通用的函数来获取它们的最大值。这个通用的函数可以接受任何一个枚举类型,并返回它的最大值。
以下是一个示例代码:
```c++
template<typename T>
int getMaxEnumValue() {
int max_value = 0;
for (int i = 0; i <= static_cast<int>(T::MAX_ENUM_VALUE); i++) {
if (i > max_value) {
max_value = i;
}
}
return max_value;
}
enum class Color1 {
RED,
GREEN,
BLUE,
MAX_ENUM_VALUE = 255
};
enum class Color2 {
CYAN,
MAGENTA,
YELLOW,
BLACK,
MAX_ENUM_VALUE = 1023
};
int main() {
int max_color1_value = getMaxEnumValue<Color1>();
int max_color2_value = getMaxEnumValue<Color2>();
std::cout << "Max value of Color1: " << max_color1_value << std::endl;
std::cout << "Max value of Color2: " << max_color2_value << std::endl;
return 0;
}
```
在上面的示例中,我们定义了一个模板函数`getMaxEnumValue()`,它可以接受任何一个枚举类型,并返回它的最大值。在函数中,我们使用了`static_cast<int>(T::MAX_ENUM_VALUE)`来获取枚举类型的最大值,其中`T`是模板参数,`MAX_ENUM_VALUE`是一个特殊的枚举常量,它用于标记枚举类型的最大值。
在`main()`函数中,我们调用`getMaxEnumValue()`函数来获取两个枚举类型`Color1`和`Color2`的最大值,并将结果输出到控制台。
color_blobs = img.find_blobs([red,blue, yellow])
color_blobs = img.find_blobs([red, blue, yellow]) 是一个用于在图像中找到一组颜色块的函数调用。接受一个包含待检测颜色的列表作为参数,并返回一个包含检测到的颜色块的列表。
在这个例子中,`red`、`blue`和`yellow`是颜色对象,可能是预定义的颜色常量或者是用于定义颜色范围的参数。`img`代表输入图像。
调用`find_blobs`函数后,返回的`color_blobs`列表包含了找到的颜色块的相关信息,比如位置、大小和像素值等。
请注意,这只是一个示例,实际使用时需要根据具体情况定义和处理颜色对象和图像对象。
相关推荐
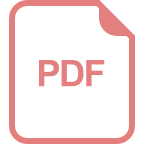
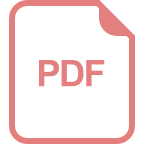
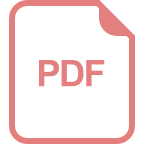












