springboot点赞功能
时间: 2023-05-08 11:58:54 浏览: 121
SpringBoot是一个非常流行的Java框架,其凭借其快速开发和简化配置的优势,使得开发Web应用变得更为轻松。在业务应用中,经常需要使用点赞功能,本文将介绍如何使用SpringBoot实现点赞功能。
首先,我们需要定义点赞的实体类,包含点赞的用户ID和点赞的文章ID。可以使用JPA的@Entity注解来定义实体类,并使用@Id注解来指定主键ID。
其次,需要定义点赞的操作服务类,可以使用@Service注解来标识,其中包含点赞和取消点赞的方法。在点赞方法中,我们需要使用JPA的save方法将点赞信息保存到数据库中,并使用Spring Cache来缓存点赞信息。在取消点赞方法中,我们需要删除数据库中的点赞信息,并清除缓存。
最后,在Spring Boot中,我们可以使用@RestController注解来定义RESTful API,提供对点赞操作服务的访问,其中包括添加和取消点赞的接口。在添加点赞和取消点赞接口中,我们需要获取用户ID和文章ID,并调用点赞服务类中的点赞和取消点赞方法。
综上所述,使用SpringBoot实现点赞功能,需要定义实体类,点赞操作服务类,以及RESTful API,其中包括添加和取消点赞接口。通过以上的步骤,就可以实现点赞功能,让用户可以轻松地点赞和取消点赞。
相关问题
springboot点赞功能实现
要实现Spring Boot的点赞功能,可以参考以下步骤:
1. 首先,设计数据库表来存储点赞信息。可以创建一个名为"likes"的表,包含字段如下:id(点赞记录的唯一标识)、user_id(点赞用户的ID)、article_id(被点赞的文章ID)等。
2. 在Spring Boot项目中,创建一个名为"LikeService"的服务类,用于处理点赞相关的
springboot实现点赞功能
很高兴回答您的问题。对于实现点赞功能,可以使用Spring Data Redis来记录用户的点赞信息,利用Redis的数据结构set或者hash来存储。在用户点击点赞时,向Redis中添加一条记录,表示该用户点赞了该条数据。在取消点赞时,从Redis中移除该记录即可。同时,可以使用Redis的计数器来记录点赞总数,以及每个数据的点赞数量。希望能对您有所帮助。
相关推荐
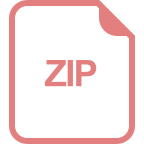
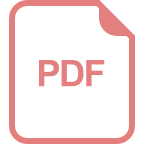
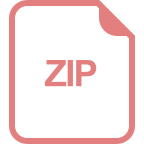
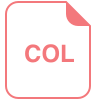
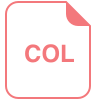










