class MobileInvertedResidualBlock(BasicUnit): def __init__(self, mobile_inverted_conv, shortcut): super(MobileInvertedResidualBlock, self).__init__() self.mobile_inverted_conv = mobile_inverted_conv self.shortcut = shortcut def forward(self, x): if self.mobile_inverted_conv.is_zero_layer(): res = x elif self.shortcut is None or self.shortcut.is_zero_layer(): res = self.mobile_inverted_conv(x) else: conv_x = self.mobile_inverted_conv(x) skip_x = self.shortcut(x) res = skip_x + conv_x return res @property def unit_str(self): return '(%s, %s)' % (self.mobile_inverted_conv.unit_str, self.shortcut.unit_str if self.shortcut is not None else None) @property def config(self): return { 'name': MobileInvertedResidualBlock.__name__, 'mobile_inverted_conv': self.mobile_inverted_conv.config, 'shortcut': self.shortcut.config if self.shortcut is not None else None, } @staticmethod def build_from_config(config): mobile_inverted_conv = set_layer_from_config( config['mobile_inverted_conv']) shortcut = set_layer_from_config(config['shortcut']) return MobileInvertedResidualBlock(mobile_inverted_conv, shortcut) def get_flops(self, x): flops1, _ = self.mobile_inverted_conv.get_flops(x) if self.shortcut: flops2, _ = self.shortcut.get_flops(x) else: flops2 = 0 return flops1 + flops2, self.forward(x)
时间: 2024-02-14 18:04:50 浏览: 32
这段代码定义了MobileInvertedResidualBlock类,它表示ProxylessNAS中的一个Mobile Inverted Residual Block。Mobile Inverted Residual Block是一种基于MobileNetV2的轻量级神经网络模块,用于构建ProxylessNAS网络架构。
MobileInvertedResidualBlock类的构造函数接受两个参数:mobile_inverted_conv和shortcut。mobile_inverted_conv是Mobile Inverted Convolution的实例,用于实现卷积操作;shortcut是一个可选项,用于实现跳跃连接。MobileInvertedResidualBlock类的前向函数forward(x)接受输入张量x,并根据是否存在shortcut来计算输出张量res。
MobileInvertedResidualBlock类还有unit_str属性和config属性,用于获取表示该类的字符串和配置字典。build_from_config方法根据配置字典构造一个MobileInvertedResidualBlock对象。get_flops方法用于获取MobileInvertedResidualBlock的计算代价(FLOPS)。
相关问题
class MobileNetV2(nn.Module): def __init__(self, num_classes=1000, width_mult=1.0, inverted_residual_setting=None, round_nearest=8, block=None, norm_layer=None):如何修改参数
你可以通过实例化 `MobileNetV2` 类时传入不同的参数来修改模型的参数,例如:
```python
model = MobileNetV2(num_classes=10, width_mult=0.5)
```
其中,`num_classes` 参数表示模型的输出类别数,`width_mult` 参数表示模型的通道数缩放比例。你还可以根据需要调整其他参数,例如 `inverted_residual_setting` 表示模型的结构设置,`round_nearest` 表示通道数取整时的最小单位,`block` 表示模型基本块的类型,`norm_layer` 表示模型使用的归一化层类型等等。如果不传入这些参数,会使用默认值。
MAV_CMD_DO_INVERTED_FLIGHT
MAV_CMD_DO_INVERTED_FLIGHT是无人机飞行控制命令之一,用于指示无人机执行倒飞动作。根据提供的引用内容,我找到了相关的信息[^1]。
根据引用,MAV_CMD_DO_INVERTED_FLIGHT的参数如下:
- Param1:保留字段,设置为0。
- Param2:保留字段,设置为0。
- Param3:保留字段,设置为0。
- Param4:保留字段,设置为0。
- Param5:保留字段,设置为0。
- Param6:保留字段,设置为0。
- Param7:保留字段,设置为0。
这个命令的作用是让无人机执行倒飞动作。具体的实现方式可能因不同的无人机系统而有所不同。如果您需要更详细的信息,建议查阅相关的无人机飞行控制文档或咨询无人机制造商。
相关推荐
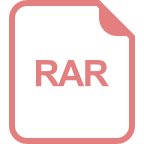













