python 实现lstm神经网络代码
时间: 2023-08-29 07:07:05 浏览: 98
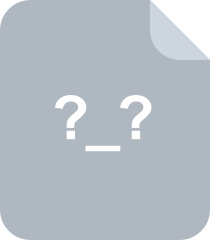
lstm 网络的资源,python实现
以下是使用Python实现LSTM神经网络的代码:
```
import numpy as np
class LSTM:
def __init__(self, input_size, output_size, hidden_size, learning_rate):
self.input_size = input_size
self.output_size = output_size
self.hidden_size = hidden_size
self.learning_rate = learning_rate
# Initialize weights and biases
self.Wf = np.random.randn(hidden_size, input_size + hidden_size)
self.Uf = np.random.randn(hidden_size, hidden_size)
self.bf = np.zeros((hidden_size, 1))
self.Wi = np.random.randn(hidden_size, input_size + hidden_size)
self.Ui = np.random.randn(hidden_size, hidden_size)
self.bi = np.zeros((hidden_size, 1))
self.Wo = np.random.randn(hidden_size, input_size + hidden_size)
self.Uo = np.random.randn(hidden_size, hidden_size)
self.bo = np.zeros((hidden_size, 1))
self.Wc = np.random.randn(hidden_size, input_size + hidden_size)
self.Uc = np.random.randn(hidden_size, hidden_size)
self.bc = np.zeros((hidden_size, 1))
self.Wy = np.random.randn(output_size, hidden_size)
self.by = np.zeros((output_size, 1))
def sigmoid(self, x):
return 1.0 / (1.0 + np.exp(-x))
def softmax(self, x):
exp_scores = np.exp(x)
return exp_scores / np.sum(exp_scores)
def forward(self, x, h_prev, c_prev):
# Concatenate input and previous hidden state
z = np.row_stack((h_prev, x))
# Forget gate
f = self.sigmoid(np.dot(self.Wf, z) + np.dot(self.Uf, h_prev) + self.bf)
# Input gate
i = self.sigmoid(np.dot(self.Wi, z) + np.dot(self.Ui, h_prev) + self.bi)
# Output gate
o = self.sigmoid(np.dot(self.Wo, z) + np.dot(self.Uo, h_prev) + self.bo)
# Candidate cell state
c_hat = np.tanh(np.dot(self.Wc, z) + np.dot(self.Uc, h_prev) + self.bc)
# Current cell state
c = f * c_prev + i * c_hat
# Current hidden state
h = o * np.tanh(c)
# Output
y = np.dot(self.Wy, h) + self.by
probs = self.softmax(y)
cache = (z, f, i, o, c_hat, c, h, y)
return probs, cache
def backward(self, dy, cache):
# Unpack cache
z, f, i, o, c_hat, c, h, y = cache
# Derivatives of output layer
dWy = np.dot(dy, h.T)
dby = np.sum(dy, axis=1, keepdims=True)
dh = np.dot(self.Wy.T, dy)
# Derivatives of hidden layer
do = dh * np.tanh(c)
do = do * o * (1 - o)
dUo = np.dot(do, h.T)
dWo = np.dot(do, z.T)
dbo = np.sum(do, axis=1, keepdims=True)
dc = dh * o * (1 - np.tanh(c) ** 2)
dc = dc + dcdy
di = dc * c_hat
di = di * i * (1 - i)
dUi = np.dot(di, h.T)
dWi = np.dot(di, z.T)
dbi = np.sum(di, axis=1, keepdims=True)
df = dc * c_prev
df = df * f * (1 - f)
dUf = np.dot(df, h.T)
dWf = np.dot(df, z.T)
dbf = np.sum(df, axis=1, keepdims=True)
# Derivatives of input vector
dz = None
dh_prev = None
dc_prev = None
return dWy, dby, dUo, dWo, dbo, dUi, dWi, dbi, dUf, dWf, dbf, dz, dh_prev, dc_prev
def optimize(self, gradients):
# Update weights and biases
self.Wy -= self.learning_rate * gradients[0]
self.by -= self.learning_rate * gradients[1]
self.Uo -= self.learning_rate * gradients[2]
self.Wo -= self.learning_rate * gradients[3]
self.bo -= self.learning_rate * gradients[4]
self.Ui -= self.learning_rate * gradients[5]
self.Wi -= self.learning_rate * gradients[6]
self.bi -= self.learning_rate * gradients[7]
self.Uf -= self.learning_rate * gradients[8]
self.Wf -= self.learning_rate * gradients[9]
self.bf -= self.learning_rate * gradients[10]
def train(self, X, y, num_epochs):
for epoch in range(num_epochs):
# Initialize hidden state and cell state
h_prev = np.zeros((self.hidden_size, 1))
c_prev = np.zeros((self.hidden_size, 1))
loss = 0
# Loop through sequence
for t in range(len(X)):
# Forward pass
x = X[t].reshape(-1, 1)
y_true = y[t].reshape(-1, 1)
probs, cache = self.forward(x, h_prev, c_prev)
loss += -np.log(probs[np.argmax(y_true)])
# Backward pass
dy = probs
dy[np.argmax(y_true)] -= 1
gradients = self.backward(dy, cache)
# Optimize
self.optimize(gradients)
# Update hidden state and cell state
h_prev = cache[6]
c_prev = cache[5]
avg_loss = loss / len(X)
print('Epoch: %d, Loss: %f' % (epoch + 1, avg_loss))
```
该代码使用numpy实现一个基本的LSTM神经网络,用于进行分类任务。在构造函数中,我们初始化权重和偏置,然后以一些特定的方式定义前向传递函数。然后,我们在backward函数中计算梯度,然后使用optimize函数更新参数。最后,在train函数中,我们一遍遍地将数据馈送到网络中,计算损失并更新权重和偏置。
阅读全文
相关推荐

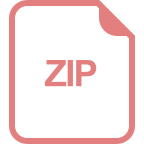
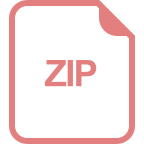
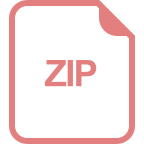
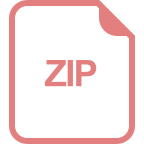
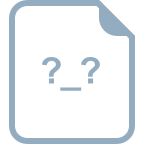
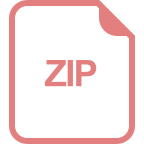
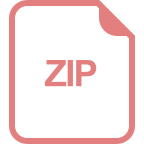
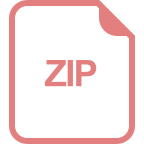
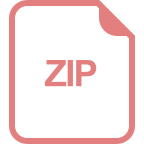
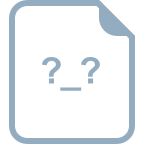
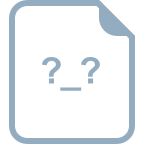
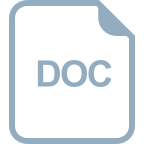

