vue3在vuex中添加动态路由无法寻找到addRoute方法
时间: 2024-02-27 18:57:44 浏览: 176
在Vue3中,使用Vue Router添加动态路由需要使用`router.addRoute`方法,而不是Vuex中的`addRoute`方法。如果你在Vuex中使用了`addRoute`方法,会导致找不到该方法的错误。
要在Vue3中添加动态路由,需要先获取Vue Router实例。可以使用`useRouter`函数获取Vue Router实例,然后再使用`addRoute`方法添加动态路由。示例代码如下:
```javascript
import { useRouter } from 'vue-router'
export default {
methods: {
addDynamicRoute() {
const router = useRouter()
router.addRoute({ path: '/dynamic', component: DynamicComponent })
}
}
}
```
这里的`DynamicComponent`是一个动态组件,需要提前定义好。注意,`addRoute`方法只能在路由初始化完成后调用,否则会出现找不到该方法的错误。
相关问题
vue2在vuex中动态添加路由
在Vuex中添加路由,需要先在router/index.js中定义一个空的路由,然后在Vuex中动态添加路由。
1. 在router/index.js中定义一个空的路由:
```
const router = new VueRouter({
routes: []
})
```
2. 在Vuex的actions中添加路由:
```
const actions = {
addRoute({ commit }, route) {
router.addRoutes([route])
commit('ADD_ROUTE', route)
}
}
```
3. 在Vuex的mutations中更新路由列表:
```
const mutations = {
ADD_ROUTE(state, route) {
state.routes.push(route)
}
}
```
4. 在组件中调用Vuex的action来动态添加路由:
```
this.$store.dispatch('addRoute', {
path: '/new-route',
component: NewRouteComponent
})
```
5. 最后,在router-view中渲染动态添加的路由:
```
<router-view v-if="$route.meta.dynamicRoute" />
```
用vue组件+vue路由+vuex+axios实现增删改查
好的,下面是一个简单的示例:
首先,安装需要的依赖:
```
npm install vue vue-router vuex axios
```
然后,在src目录下,创建以下文件:
1. `api.js`:定义与后端交互的API请求。
2. `store.js`:定义Vuex状态管理器。
3. `router.js`:定义Vue路由器。
4. `views`目录:定义Vue组件。
`api.js`文件内容如下:
```js
import axios from 'axios';
const baseUrl = 'http://localhost:3000'; // 后端API地址
const api = axios.create({
baseURL: baseUrl,
timeout: 10000,
});
export default {
async getTodos() {
const response = await api.get('/todos');
return response.data;
},
async addTodo(todo) {
const response = await api.post('/todos', todo);
return response.data;
},
async updateTodo(todo) {
const response = await api.put(`/todos/${todo.id}`, todo);
return response.data;
},
async deleteTodoById(id) {
const response = await api.delete(`/todos/${id}`);
return response.data;
},
};
```
`store.js`文件内容如下:
```js
import Vue from 'vue';
import Vuex from 'vuex';
import api from './api';
Vue.use(Vuex);
export default new Vuex.Store({
state: {
todos: [],
},
mutations: {
setTodos(state, todos) {
state.todos = todos;
},
addTodo: (state, todo) => {
state.todos.push(todo);
},
updateTodo: (state, todo) => {
const index = state.todos.findIndex((t) => t.id === todo.id);
Vue.set(state.todos, index, todo);
},
deleteTodoById: (state, id) => {
const index = state.todos.findIndex((t) => t.id === id);
state.todos.splice(index, 1);
},
},
actions: {
async fetchTodos({ commit }) {
const todos = await api.getTodos();
commit('setTodos', todos);
},
async addTodo({ commit }, todo) {
const newTodo = await api.addTodo(todo);
commit('addTodo', newTodo);
},
async updateTodoById({ commit }, todo) {
const updatedTodo = await api.updateTodo(todo);
commit('updateTodo', updatedTodo);
},
async deleteTodoById({ commit }, id) {
await api.deleteTodoById(id);
commit('deleteTodoById', id);
},
},
});
```
`router.js`文件内容如下:
```js
import Vue from 'vue';
import VueRouter from 'vue-router';
import TodoList from './views/TodoList.vue';
import AddTodo from './views/AddTodo.vue';
import EditTodo from './views/EditTodo.vue';
Vue.use(VueRouter);
export default new VueRouter({
routes: [
{
path: '/',
name: 'home',
component: TodoList,
},
{
path: '/add',
name: 'add',
component: AddTodo,
},
{
path: '/edit/:id',
name: 'edit',
component: EditTodo,
},
],
});
```
最后,`views`目录下的组件分别为:
1. `TodoList.vue`:展示所有待办事项。
2. `AddTodo.vue`:添加新待办事项。
3. `EditTodo.vue`:编辑已有待办事项。
`TodoList.vue`文件内容如下:
```vue
<template>
<div>
<h1>Todo List</h1>
<ul>
<li v-for="todo in todos" :key="todo.id">
{{ todo.title }} - {{ todo.completed ? 'Completed' : 'Not Completed' }}
<router-link :to="{ name: 'edit', params: { id: todo.id }}">Edit</router-link>
<button @click="deleteTodoById(todo.id)">Delete</button>
</li>
</ul>
<router-link :to="{ name: 'add' }">Add Todo</router-link>
</div>
</template>
<script>
export default {
data() {
return {
todos: [],
};
},
async mounted() {
await this.$store.dispatch('fetchTodos');
this.todos = this.$store.state.todos;
},
methods: {
async deleteTodoById(id) {
await this.$store.dispatch('deleteTodoById', id);
},
},
};
</script>
```
`AddTodo.vue`文件内容如下:
```vue
<template>
<div>
<h1>Add Todo</h1>
<form @submit.prevent="addTodo">
<label for="title">Title:</label>
<input type="text" id="title" v-model="title" required />
<br />
<label for="completed">Completed:</label>
<input type="checkbox" id="completed" v-model="completed" />
<br />
<button type="submit">Add</button>
</form>
</div>
</template>
<script>
export default {
data() {
return {
title: '',
completed: false,
};
},
methods: {
async addTodo() {
const todo = {
title: this.title,
completed: this.completed,
};
await this.$store.dispatch('addTodo', todo);
this.$router.push('/');
},
},
};
</script>
```
`EditTodo.vue`文件内容如下:
```vue
<template>
<div>
<h1>Edit Todo</h1>
<form @submit.prevent="updateTodo">
<label for="title">Title:</label>
<input type="text" id="title" v-model="todo.title" required />
<br />
<label for="completed">Completed:</label>
<input type="checkbox" id="completed" v-model="todo.completed" />
<br />
<button type="submit">Save</button>
</form>
</div>
</template>
<script>
export default {
data() {
return {
todo: {},
};
},
async mounted() {
const id = this.$route.params.id;
const todos = this.$store.state.todos;
this.todo = todos.find((t) => t.id === Number(id)) || {};
},
methods: {
async updateTodo() {
await this.$store.dispatch('updateTodoById', this.todo);
this.$router.push('/');
},
},
};
</script>
```
到此为止,使用Vue组件+Vue路由+Vuex+Axios实现增删改查的示例完成了。
阅读全文
相关推荐
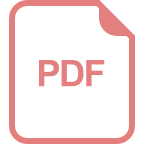
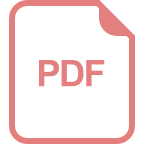
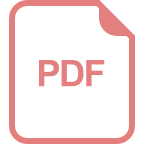
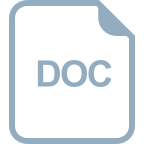
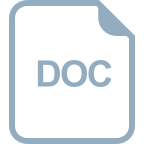


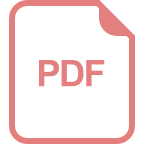
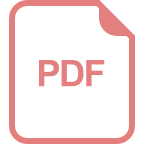
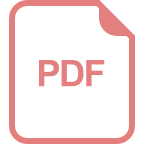
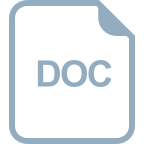
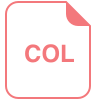



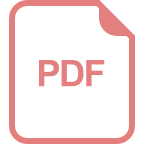