bool compare_scores(const pair<string, string>& a, const pair<string, string>& b) { double score_a = stod(a.second, nullptr); double score_b = stod(b.second, nullptr); if (isnan(score_a)) { score_a = 0; } if (isnan(score_b)) { score_b = 0; } return score_a > score_b; } void management::print_scores_sorted(const vector<course>& courses_for_student) { vector<pair<string, string>> scores_vec; for (const auto& c : courses_for_student) { scores_vec.push_back(make_pair(c.m_coursename, c.m_score)); } sort(scores_vec.begin(), scores_vec.end(), compare_scores); scores_vec.erase(unique(scores_vec.begin(), scores_vec.end(), [](const pair<string, string>& a, const pair<string, string>& b) { return a.first == b.first && a.second == b.second; }), scores_vec.end()); for (const auto& s : scores_vec) { cout << s.first << "\t" << s.second << endl; } }能否帮我修改这段代码,让这两个函数完成对成绩由高到低排序并且去重输出
时间: 2024-03-07 10:50:48 浏览: 20
当对成绩进行排序时,我们需要将字符串转换为浮点数进行比较而不是直接使用字符串进行比较。在排序后,我们可以使用unique函数去除相邻重复的元素。以下是修改后的代码:
```
bool compare_scores(const pair<string, string>& a, const pair<string, string>& b) {
double score_a = stod(a.second, nullptr);
double score_b = stod(b.second, nullptr);
if (isnan(score_a)) {
score_a = 0;
}
if (isnan(score_b)) {
score_b = 0;
}
return score_a > score_b;
}
void management::print_scores_sorted(const vector<course>& courses_for_student) {
vector<pair<string, string>> scores_vec;
for (const auto& c : courses_for_student) {
scores_vec.push_back(make_pair(c.m_coursename, c.m_score));
}
sort(scores_vec.begin(), scores_vec.end(), compare_scores);
scores_vec.erase(unique(scores_vec.begin(), scores_vec.end()), scores_vec.end());
for (const auto& s : scores_vec) {
cout << s.first << "\t" << s.second << endl;
}
}
```
相关推荐
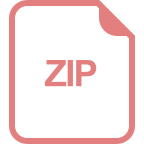
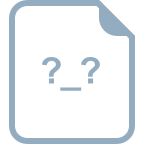











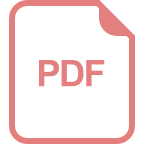
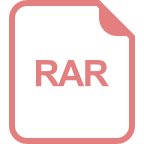
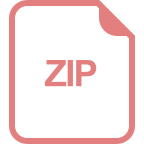