module mppcs_block_dec #( parameter DW = 32, /// max. data width parameter HW = 4, /// max. header width parameter ND = 16 /// max. number of data per block ) ( /// ingress data interface input logic [DW-1:0] data_in, /// ingress data before header extraction input logic in_valid, /// ingress flow control output logic in_ready, /// ingress flow control /// egress data interface output logic block_start, /// block synchronization output logic [HW-1:0] header_out, /// block header output logic [DW-1:0] data_out, /// egress data after header extraction output logic out_valid, /// egress flow control input logic out_ready, /// egress flow control /// control options input [$clog2(DW)-1:0] msb_data, /// number of data bits - 1 input [$clog2(HW)-1:0] msb_header, /// number of header bits - 1 input [$clog2(ND)-1:0] msb_num_data, /// number of data per block - 1 output logic sync_track, /// block sync tracking signal input logic sync_mode, /// 0: use external sync directly, 1 : use internal sync after assertion of external sync input logic [3:0] sync_offset, /// offset between sync and block start signal input logic sync_start, /// external sync input logic enable, /// 0: clock-gated, 1: mission mode input logic bypass, /// 1: data pass-through without header insertion /// clock & reset input clk, input rst );
时间: 2024-04-26 22:25:28 浏览: 171
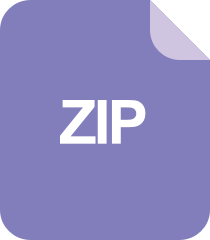
jiaminghi/data-view dataV for vite vue2 补丁 for npm/yarn

这是一个Verilog HDL代码模块,实现了一个带有数据和头部的块解码器。它的作用是从数据流中提取出块,并将数据和头部分别输出。模块中包含了许多参数,如最大数据宽度DW、最大头部宽度HW、每个块中的最大数据数目ND等。模块的输入包括数据流、数据有效信号、流控制信号等;输出包括块同步信号、头部、数据、数据有效信号等。此外,还包括一些控制选项,如数据位数、头部位数、每个块中数据的数量等。模块还包括时钟和复位信号。
阅读全文
相关推荐
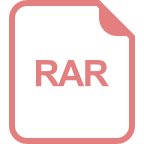
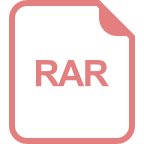


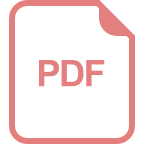
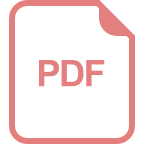
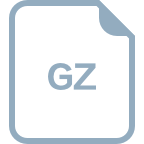
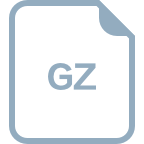
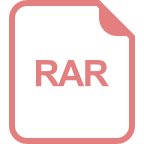
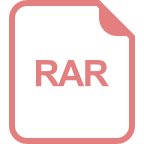
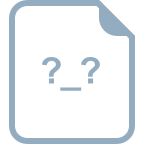
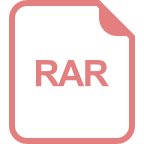
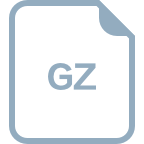
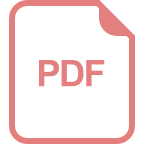
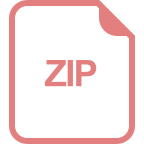
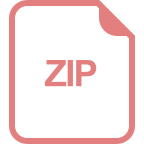
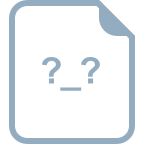