linux mmap映射文件 文件中存放map类型数据,通过数组的方式修改数据文件,实现代码
时间: 2024-03-06 07:46:28 浏览: 40
下面是一个简单的 Linux C 语言程序,用于在内存中映射一个文件,然后通过数组的方式修改 map 类型的数据文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <sys/mman.h>
#include <unistd.h>
#include <string.h>
#include <errno.h>
#include <stdbool.h>
#include <stdint.h>
#include <map>
#define FILE_SIZE 1024
typedef struct {
int id;
char name[20];
} Person;
int main() {
int fd;
Person *p_map;
std::map<int, Person> *person_map;
if ((fd = open("file", O_RDWR | O_CREAT | O_TRUNC, 0666)) < 0) {
perror("open error");
exit(1);
}
lseek(fd, FILE_SIZE - 1, SEEK_SET);
write(fd, "", 1);
if ((p_map = (Person *) mmap(NULL, FILE_SIZE, PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0)) == MAP_FAILED) {
perror("mmap error");
exit(1);
}
person_map = new(std::nothrow) std::map<int, Person>;
// 将映射区域中的数据读入 map 中
for (int i = 0; i < FILE_SIZE / sizeof(Person); i++) {
if (p_map[i].id > 0) {
(*person_map)[p_map[i].id] = p_map[i];
}
}
// 在 map 中插入新的数据
Person new_person = {1001, "Alice"};
(*person_map)[new_person.id] = new_person;
// 将 map 中的数据写回到映射区域
int i = 0;
for (auto it = person_map->begin(); it != person_map->end(); it++) {
memcpy(&p_map[i++], &(it->second), sizeof(Person));
}
munmap(p_map, FILE_SIZE);
close(fd);
// 通过数组的方式修改 map 类型的数据文件
if ((fd = open("file", O_RDWR)) < 0) {
perror("open error");
exit(1);
}
Person persons[FILE_SIZE / sizeof(Person)];
memset(persons, 0, FILE_SIZE);
if (read(fd, persons, FILE_SIZE) < 0) {
perror("read error");
exit(1);
}
for (int i = 0; i < FILE_SIZE / sizeof(Person); i++) {
if (persons[i].id == 1001) {
strcpy(persons[i].name, "Bob");
break;
}
}
if (lseek(fd, 0, SEEK_SET) < 0) {
perror("lseek error");
exit(1);
}
if (write(fd, persons, FILE_SIZE) < 0) {
perror("write error");
exit(1);
}
close(fd);
return 0;
}
```
上面的代码中,我们首先创建了一个文件,然后使用 mmap 函数将该文件映射到内存中。接着,我们使用 C++ STL 中的 map 类型来存储数据,这个 map 类型的 key 是 int 类型,value 是 Person 类型的结构体。我们从映射的内存区域中读取数据,将其存储到 map 中,然后在 map 中插入新的数据,并将 map 中的数据写回到映射区域。最后,我们释放映射的内存区域,并关闭文件。
接着,我们通过数组的方式打开文件,读取数据到内存中,找到对应的数据进行修改,然后将修改后的数据写回到文件中。注意,这里我们使用了 lseek 函数将文件指针移到文件开头,然后使用 write 函数将数据写回到文件中。
相关推荐
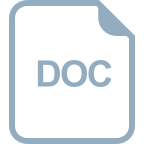














