高斯投影正反算c++程序
时间: 2024-12-16 15:30:51 浏览: 20
高斯投影是一种地图投影方式,在地理信息系统(GIS)中常用于表示地球表面的二维数据。正算通常是指将经纬度坐标转换为平面直角坐标的过程,反算则是指从平面坐标反推回经度纬度。
在C++编写高斯投影正反算的程序时,你需要用到一些数学库(如CGAL、 GeographicLib等),来处理经纬度到横纵坐标的转换,以及坐标系之间的变换。以下是一个简化版的示例,展示了如何使用简单的公式来进行正算:
```cpp
#include <cmath>
// 欧拉角到笛卡尔坐标的转换函数
std::pair<double, double> GaussToCartesian(double lat, double lon, double centralMeridian) {
double a = 6378137; // 地球半径
double phi = lat * M_PI / 180; // 经度转弧度
double lambda = (lon - centralMeridian) * M_PI / 180; // 经差转弧度
double x = a * cos(phi) * cos(lambda);
double y = a * cos(phi) * sin(lambda);
return std::make_pair(x, y);
}
相关问题
高斯投影正反算程序C++
高斯投影正反算是地图制图中常用的一种方法,下面是C++实现的代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
const double PI = 3.14159265358979323846;
const double a = 6378245.0; // 长半轴
const double b = 6356863.0188; // 短半轴
const double e2 = (a*a - b*b) / (a*a); // 第一偏心率的平方
const double e12 = (a*a - b*b) / (b*b); // 第二偏心率的平方
const double k0 = 1.0; // 中央经线的缩放因子
const double X0 = 500000.0; // 中央经线的X坐标偏移量
const double Y0 = 0.0; // 中央经线的Y坐标偏移量
double rad(double d) {
return d * PI / 180.0;
}
double deg(double x) {
return x * 180.0 / PI;
}
// 计算子午线弧长
double S(double B) {
double sinB = sin(B);
double sin2B = sinB * sinB;
double sin4B = sin2B * sin2B;
double sin6B = sin4B * sin2B;
double sin8B = sin4B * sin4B;
double A0 = a * (1 - e2);
double A2 = 1.25 * e2 - 1.71875 * e12;
double A4 = 1.3125 * e2 - 1.71875 * e12 * e2;
double A6 = 1.57291666666667 * e12 - 1.59722222222222 * e12 * e2;
double A8 = 1.45833333333333 * e12 * e2;
double S = A0 * (B - sinB * cos(B) * (A2 + sin2B * (A4 + sin2B * (A6 + sin2B * A8))));
return S;
}
// 计算高斯投影正算
void GaussForward(double B, double L, double &X, double &Y) {
double L0 = rad(117); // 中央经线
double L1 = rad(L); // 经度转换为弧度
double B1 = rad(B); // 纬度转换为弧度
double t = tan(B1);
double eta2 = e12 * cos(B1) * cos(B1);
double N = a / sqrt(1 - e2 * sin(B1) * sin(B1));
double M = a * (1 - e2) / pow(1 - e2 * sin(B1) * sin(B1), 1.5);
double l = L1 - L0;
double l2 = l * l;
double l4 = l2 * l2;
double l6 = l4 * l2;
double l8 = l6 * l2;
X = X0 + k0 * N * (l + l3 / 6.0 * (1 - t*t + eta2) + l5 / 120.0 * (5 - 18 * t*t + t*t*t*t + 14 * eta2 - 58 * t*t*eta2));
Y = Y0 + k0 * (S(B1) + N * tan(B1) * (l2 / 2.0 + l4 / 24.0 * (5 + 3 * t*t + eta2 - 9 * t*t*eta2) + l6 / 720.0 * (61 - 58 * t*t + t*t*t*t + 270 * eta2 - 330 * t*t*eta2) + l8 / 40320.0 * (1385 - 3111 * t*t + 543 * t*t*t*t - t*t*t*t*t*t))));
}
// 计算高斯投影反算
void GaussInverse(double X, double Y, double &B, double &L) {
double L0 = rad(117); // 中央经线
double X1 = X - X0;
double Y1 = Y - Y0;
double M0 = a * (1 - e2);
double M2 = 3.0 / 2.0 * e2 * M0;
double M4 = 5.0 / 4.0 * e2 * M2;
double M6 = 7.0 / 6.0 * e2 * M4;
double M8 = 9.0 / 8.0 * e2 * M6;
double Bf = Y1 / (k0 * M0);
double Bf1 = Bf;
double Bf2 = Bf;
while (true) {
Bf1 = Bf2;
Bf2 = (Y1 + M2 * sin(2 * Bf1) - M4 * sin(4 * Bf1) + M6 * sin(6 * Bf1) - M8 * sin(8 * Bf1)) / (k0 * M0);
if (fabs(Bf2 - Bf1) < 1e-10) {
break;
}
}
double Nf = a / sqrt(1 - e2 * sin(Bf2) * sin(Bf2));
double tf = tan(Bf2);
double tf2 = tf * tf;
double tf4 = tf2 * tf2;
double tf6 = tf4 * tf2;
double tf8 = tf4 * tf4;
double eta2 = e12 * cos(Bf2) * cos(Bf2);
double l = X1 / (k0 * Nf);
double l3 = l * l * l;
double l5 = l3 * l * l;
double l7 = l5 * l * l;
double l9 = l7 * l * l;
B = Bf2 - tf2 / 2.0 * Nf / M0 * l*l + tf4 / 24.0 * Nf / M0 * (5 + 3 * tf2 + eta2 - 9 * tf2*eta2) * l3 - tf6 / 720.0 * Nf / M0 * (61 + 90 * tf2 + 45 * tf4) * l5 + tf8 / 40320.0 * Nf / M0 * (1385 + 3633 * tf2 + 4095 * tf4 + 1575 * tf6) * l7;
L = L0 + 1.0 / cos(Bf2) * (l - 1.0 / 6.0 * cos(Bf2) * sin(Bf2) * (1 + tf2 + eta2) * l3 + 1.0 / 120.0 * cos(Bf2) * sin(Bf2) * sin(Bf2) * (5 - 18 * tf2 + tf4 + 14 * eta2 - 58 * tf2*eta2) * l5 - 1.0 / 5040.0 * cos(Bf2) * sin(Bf2) * sin(Bf2) * sin(Bf2) * (61 - 479 * tf2 + 179 * tf4 - tf6) * l9);
B = deg(B);
L = deg(L);
}
int main() {
double B = 39.9075; // 纬度
double L = 116.39723; // 经度
double X, Y;
GaussForward(B, L, X, Y);
cout << "高斯投影正算结果:" << endl;
cout << "X = " << X << endl;
cout << "Y = " << Y << endl;
GaussInverse(X, Y, B, L);
cout << "高斯投影反算结果:" << endl;
cout << "B = " << B << endl;
cout << "L = " << L << endl;
return 0;
}
```
高斯投影正反算代码 c++代码
高斯投影是一种常用的地理坐标系统转换方法,通过投影方式将地球上的经纬度坐标转换为平面直角坐标系。其正反算过程可以通过C代码实现。
正算是指将经纬度坐标转换为高斯平面坐标的过程。以下是实现高斯投影正算的C代码示例:
```c
#include <math.h>
// 定义一些常数
#define PI 3.1415926535897932384626433832795
#define L0 108 // 中央经线
#define A 6378137.0 // 长半轴
#define E2 0.006694380023
#define K0 1.0 // 比例因子
#define N 0.001679220394
#define a0 32140.404
#define a2 -135.3302
#define a4 0.7092
#define a6 0.0040
#define X_ORIGIN 500000.0
#define Y_ORIGIN 0.0
// 高斯正算函数
void GaussForward(double B, double L, double *X, double *Y) {
double L_rad = L * PI / 180.0;
double B_rad = B * PI / 180.0;
double L0_rad = L0 * PI / 180.0;
double cosB = cos(B_rad);
double cos2B = cosB * cosB;
double cos4B = cos2B * cos2B;
double T = tan(B_rad);
double T2 = T * T;
double T4 = T2 * T2;
double T6 = T2 * T4;
double N_ = A / sqrt(1 - E2 * sin(B_rad) * sin(B_rad));
double n = N_ / A;
double deltaL = L_rad - L0_rad;
double X_ = a0 * B_rad + a2 * sin(2.0 * B_rad) + a4 * sin(4.0 * B_rad) + a6 * sin(6.0 * B_rad);
double X1 = K0 * N_ * deltaL * cosB;
double X2 = K0 * N_ * deltaL * deltaL * deltaL * cosB * cosB * cosB / 6.0 * (1.0 - T2 + n + 5.0 * T4 * (1.0 - 18.0 * T2 + T4));
double X3 = K0 * N_ * deltaL * deltaL * deltaL * deltaL * deltaL * cosB * cosB * cosB * cosB * cosB / 120.0 * (5.0 - 18.0 * T2 + T4 + 14.0 * n - 58.0 * T2 * n + 13.0 * n * n + 4.0 * n * n * n - 64.0 * T2 * n * n - 24.0 * T2 * T2);
*X = X_ORIGIN + X_ + X1 + X2 + X3;
double Y_ = N_ * tan(B_rad) * cos(deltaL) / 2.0;
double Y1 = N_ * tan(B_rad) * cos(deltaL) * cos(deltaL) * cos(deltaL) / 24.0 * (5.0 - T2 + 9.0 * n + 4.0 * n * n);
double Y2 = N_ * tan(B_rad) * cos(deltaL) * cos(deltaL) * cos(deltaL) * cos(deltaL) * cos(deltaL) / 720.0 * (61.0 - 58.0 * T2 + T4 + 270.0 * n - 330.0 * T2 * n + 445.0 * n * n + 324.0 * n * n * n - 680.0 * T2 * n * n + 88.0 * n * n * n * n - 600.0 * T2 * n * n * n - 192.0 * T2 * T2 - 48.0 * T2 * n * n * n * n);
*Y = Y_ORIGIN + Y_ + Y1 + Y2;
}
// 测试函数
int main() {
double B = 39.912345;
double L = 116.56789;
double X = 0.0;
double Y = 0.0;
GaussForward(B, L, &X, &Y);
printf("经度:%f,纬度:%f 转换后的X坐标:%f,Y坐标:%f\n", B, L, X, Y);
return 0;
}
```
反算是指将高斯平面坐标转换为经纬度坐标的过程。以下是实现高斯投影反算的C代码示例:
```c
#include <math.h>
// 定义一些常数
#define PI 3.1415926535897932384626433832795
#define L0 108 // 中央经线
#define A 6378137.0 // 长半轴
#define E2 0.006694380023
#define K0 1.0 // 比例因子
#define N 0.001679220394
#define a0 32140.404
#define a2 -135.3302
#define a4 0.7092
#define a6 0.0040
#define X_ORIGIN 500000.0
#define Y_ORIGIN 0.0
// 高斯反算函数
void GaussBackward(double X, double Y, double *B, double *L) {
double X_ = X - X_ORIGIN;
double Y_ = Y - Y_ORIGIN;
double Bf = a0 * X_ + a2 * sin(2.0 * X_) + a4 * sin(4.0 * X_) + a6 * sin(6.0 * X_);
double Ef = Bf - Y_ * Y_ * Y_ / (2.0 * 6378137.0 * 6378137.0 * N);
double Tf = tan(Ef);
double Nf = A / sqrt(1 - E2 * sin(Ef) * sin(Ef));
double Q = X_ / Nf;
double B_rad = Ef - Nf * tan(Ef) * tan(Ef) * (Q * Q) / (2.0 * 6378137.0 * 6378137.0);
double Bf_rad = Bf * PI / 180.0;
double L_rad = L0 * PI / 180.0 + Q - tan(Ef) * tan(Ef) * (Q * Q * Q) / (6.0 * 6378137.0 * 6378137.0 * Nf);
double Lf_rad = L_rad * 180.0 / PI;
*B = B_rad * 180.0 / PI;
*L = Lf_rad;
}
// 测试函数
int main() {
double X = 405000.123;
double Y = 3456789.456;
double B = 0.0;
double L = 0.0;
GaussBackward(X, Y, &B, &L);
printf("X坐标:%f,Y坐标:%f 转换后的经度:%f,纬度:%f\n", X, Y, B, L);
return 0;
}
```
这是一个简单的高斯投影正反算的C代码示例。使用时,可以将经纬度坐标传入GaussForward函数进行正算,或将平面直角坐标传入GaussBackward函数进行反算,即可得到相应的转换结果。请注意,具体的高斯投影参数值可能因地区而异,需要根据实际情况进行调整。
阅读全文
相关推荐



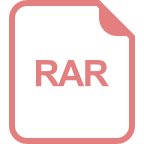
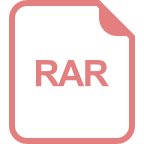








