sql中如何追加语句
时间: 2024-09-22 20:02:46 浏览: 25
在MyBatis中,要实现SQL的动态追加条件,你可以利用Interceptor(拦截器)和自定义注解来完成。以下是如何操作的一个示例:
首先,创建一个自定义注解`@DataScope`,这个注解将在特定的方法或接口上应用,指示需要添加额外的SQL条件:
```java
// 自定义注解
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface DataScope {
String condition() default "";
}
```
接着,在Interceptor中,我们拦截Mapper接口的方法调用,并在执行SQL之前检查是否存在`@DataScope`注解。如果存在,就在原始的SQL中追加条件:
```java
// 拦截器实现
public class ScopeInterceptor implements Interceptor {
@Override
public Object intercept(Invocation invocation) throws Throwable {
Method method = invocation.getMethod();
if (method.isAnnotationPresent(DataScope.class)) {
DataScope annotation = method.getAnnotation(DataScope.class);
String sql = (String) ((SqlSource) invocation.getArgs()[0]).getSql();
String appendedCondition = annotation.condition(); // 获取自定义条件
sql += " AND " + appendedCondition;
// 设置修改后的SQL
SqlSource newSqlSource = new SqlCommandSource(sql, null);
return invocation.proceed(newSqlSource);
}
return invocation.proceed();
}
// 其他必要的Interceptor接口方法...
}
```
最后,要在Mapper接口上使用拦截器,配置Spring或MyBatis的Interceptor设置:
```java
@Configuration
public class MyBatisConfig {
@Bean
public ScopeInterceptor scopeInterceptor() {
return new ScopeInterceptor();
}
@Bean
@ConfigurationProperties(prefix = "mybatis")
public SqlSessionFactory sqlSessionFactory(ScopeInterceptor scopeInterceptor) {
SqlSessionFactoryBuilder builder = new SqlSessionFactoryBuilder();
Map<String, Object> configMap = new HashMap<>();
configMap.put("plugins", Arrays.asList(scopeInterceptor));
return builder.build(configMap);
}
}
```
现在,当你在一个Mapper接口的方法上使用`@DataScope`注解时,执行的SQL会自动包含你在注解中指定的条件。
阅读全文
相关推荐
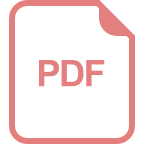
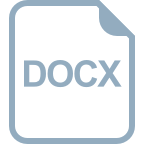
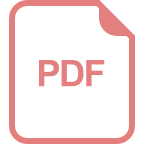















