需求:网页上搜索word文档某一个章节,输出显示这一个章节的具体内容。使用python,flask,docx库的paragraphs,要求网页上显示的内容和word上样式排版一摸一样,同时内嵌图片包括位置也一样,图片可采用base64,请给出详细可用且比较新的代码
时间: 2024-02-23 19:01:52 浏览: 126
好的,以下是一个基于 Flask 和 docx 库的简单示例代码,可以实现你的需求。需要注意的是,这个示例代码只是一个简单的演示,实际应用中需要进行更多的优化和安全措施。
首先需要安装 Flask 和 docx 库,可以在命令行中运行以下命令:
```
pip install flask
pip install python-docx
```
接下来是代码实现:
```python
from flask import Flask, request, render_template, make_response
import base64
import io
from docx import Document
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/search', methods=['POST'])
def search():
# 获取上传的 Word 文件
file = request.files['file']
# 读取 Word 文件内容
doc = Document(file)
# 获取要搜索的章节标题
title = request.form['title']
# 遍历所有段落,查找匹配的章节
content = ""
for para in doc.paragraphs:
if para.style.name.startswith('Heading') and para.text == title:
# 找到了目标章节,将其内容和样式拼接到 content 变量中
content += para.text + '\n'
for run in para.runs:
if run.bold:
content += '<b>'
if run.italic:
content += '<i>'
content += run.text
if run.italic:
content += '</i>'
if run.bold:
content += '</b>'
content += '<br><br>'
for pic in para.inline_shapes:
# 将图片转换为 base64 编码,并嵌入到 HTML 中
img_stream = io.BytesIO()
pic.image.save(img_stream, format='png')
img_data = base64.b64encode(img_stream.getvalue()).decode('utf-8')
content += '<img src="data:image/png;base64,{}">'.format(img_data)
# 将 content 变量中的内容渲染到模板中,返回给用户
return make_response(render_template('search_result.html', content=content))
if __name__ == '__main__':
app.run(debug=True)
```
代码中用到了两个模板文件:`index.html` 和 `search_result.html`。`index.html` 是上传 Word 文件的页面,`search_result.html` 是搜索结果的页面。这两个模板文件的内容如下:
`index.html`:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>搜索 Word 章节</title>
</head>
<body>
<h1>搜索 Word 章节</h1>
<form method="post" action="{{ url_for('search') }}" enctype="multipart/form-data">
<label>上传 Word 文件:</label>
<input type="file" name="file">
<br><br>
<label>输入要搜索的章节标题:</label>
<input type="text" name="title">
<br><br>
<button type="submit">搜索</button>
</form>
</body>
</html>
```
`search_result.html`:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>搜索结果</title>
</head>
<body>
<h1>搜索结果</h1>
<div>
{{ content | safe }}
</div>
</body>
</html>
```
以上就是一个基于 Flask 和 docx 库的简单示例代码,可以实现网页上搜索 Word 文档某一个章节,输出显示这一个章节的具体内容,包括样式和图片。
阅读全文
相关推荐
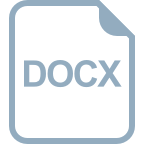
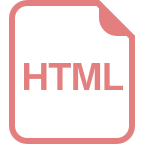




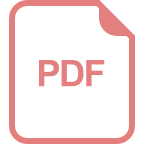
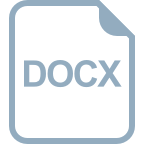
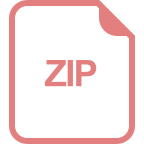
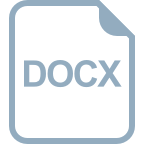
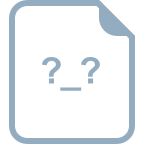
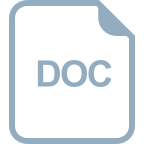
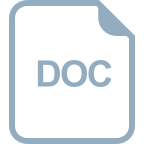


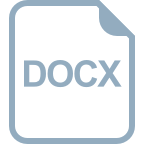
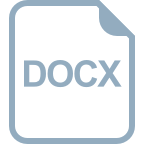
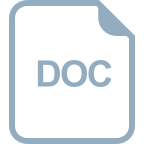