使用udp实现即时聊天C语言fork
时间: 2023-06-11 19:09:18 浏览: 101
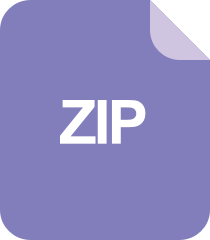
c语言实现的udp聊天室
以下是使用UDP实现即时聊天的C语言代码示例,其中包含了fork()创建子进程的部分:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#define PORT 8888
#define MAX_MSG_LEN 1024
int main() {
int sockfd;
struct sockaddr_in server_addr, client_addr;
char buf[MAX_MSG_LEN];
pid_t pid;
// 创建UDP套接字
if ((sockfd = socket(AF_INET, SOCK_DGRAM, 0)) < 0) {
perror("socket failed");
exit(EXIT_FAILURE);
}
// 设置服务器地址
memset(&server_addr, 0, sizeof(server_addr));
server_addr.sin_family = AF_INET;
server_addr.sin_addr.s_addr = htonl(INADDR_ANY);
server_addr.sin_port = htons(PORT);
// 绑定服务器地址
if (bind(sockfd, (struct sockaddr *)&server_addr, sizeof(server_addr)) < 0) {
perror("bind failed");
exit(EXIT_FAILURE);
}
printf("Server is listening on port %d...\n", PORT);
while (1) {
socklen_t client_len = sizeof(client_addr);
// 接收客户端消息
int recv_len = recvfrom(sockfd, buf, MAX_MSG_LEN, 0, (struct sockaddr *)&client_addr, &client_len);
if (recv_len < 0) {
perror("recvfrom failed");
exit(EXIT_FAILURE);
}
// 打印客户端消息
buf[recv_len] = '\0';
printf("Client %s:%d says: %s\n", inet_ntoa(client_addr.sin_addr), ntohs(client_addr.sin_port), buf);
// 创建子进程处理客户端消息
pid = fork();
if (pid == -1) {
perror("fork failed");
exit(EXIT_FAILURE);
} else if (pid == 0) { // 子进程
// 发送回复消息给客户端
char reply[MAX_MSG_LEN];
sprintf(reply, "Hello, %s:%d! I'm the server.", inet_ntoa(client_addr.sin_addr), ntohs(client_addr.sin_port));
int send_len = sendto(sockfd, reply, strlen(reply), 0, (struct sockaddr *)&client_addr, sizeof(client_addr));
if (send_len < 0) {
perror("sendto failed");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS); // 子进程结束
}
}
return 0;
}
```
在上述代码中,使用了fork()函数创建子进程处理客户端消息。当接收到客户端的UDP消息后,主进程创建一个子进程,子进程负责处理客户端的请求,主进程继续监听其他客户端的消息。
需要注意的是,使用fork()函数创建子进程时,需要对父进程和子进程分别进行不同的操作,因此需要使用pid_t类型的变量来保存fork()函数的返回值,根据返回值来判断当前进程是父进程还是子进程。在子进程中处理完客户端的请求后,需要调用exit()函数结束子进程。
阅读全文
相关推荐
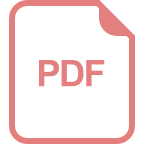
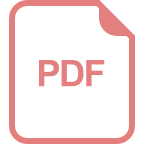
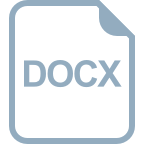
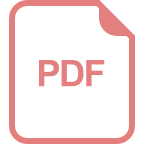
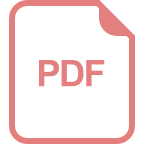
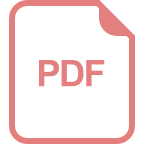
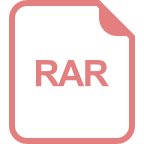
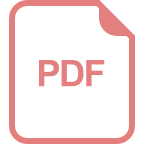
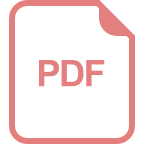
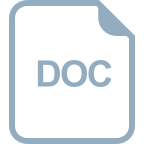
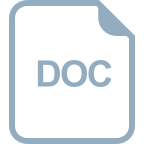
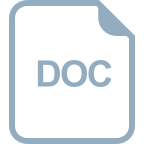
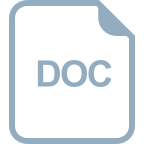
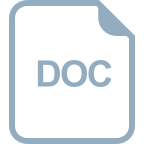
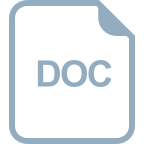
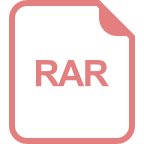