C语言fork两个子进程并读取父进程写入管道中的信息
时间: 2024-05-09 08:19:18 浏览: 13
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <string.h>
int main() {
int fd[2];
pid_t pid1, pid2;
if (pipe(fd) == -1) {
printf("Pipe failed\n");
return 1;
}
pid1 = fork();
if (pid1 < 0) {
printf("Fork 1 failed\n");
return 1;
} else if (pid1 == 0) { // 子进程1
close(fd[1]);
char buffer[100];
int n = read(fd[0], buffer, sizeof(buffer));
if (n > 0) {
buffer[n] = '\0';
printf("Child process 1 received message: %s", buffer);
}
close(fd[0]);
exit(0);
} else { // 父进程
pid2 = fork();
if (pid2 < 0) {
printf("Fork 2 failed\n");
return 1;
} else if (pid2 == 0) { // 子进程2
close(fd[1]);
char buffer[100];
int n = read(fd[0], buffer, sizeof(buffer));
if (n > 0) {
buffer[n] = '\0';
printf("Child process 2 received message: %s", buffer);
}
close(fd[0]);
exit(0);
} else { // 父进程
close(fd[0]);
char message[] = "Hello, child processes!\n";
int n = write(fd[1], message, strlen(message));
if (n == -1) {
printf("Write to pipe failed\n");
}
close(fd[1]);
wait(NULL);
wait(NULL);
}
}
return 0;
}
```
相关推荐
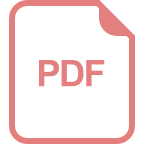
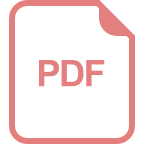
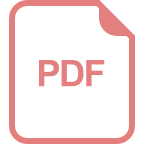














