java中使用oo思想收银结算,商品(名称,数量,单价),有非打折商品,打折商品(8折,9折),将来可能会有对折,返现等不同优惠。 编写一个程序,对一组商品 (用户输入) 进行录入并统计总价
时间: 2024-06-10 15:05:30 浏览: 17
好的,根据您的要求,我来为您解答这个问题。
为了实现这个功能,我们可以先创建一个商品类,包含名称、数量和单价三个属性,并为类定义一个计算商品总价的方法。
```java
public class Product {
private String name; // 商品名称
private int quantity; // 商品数量
private double unitPrice; // 商品单价
private boolean discount; // 是否打折
public Product(String name, int quantity, double unitPrice) {
this.name = name;
this.quantity = quantity;
this.unitPrice = unitPrice;
this.discount = false; // 默认为非打折商品
}
public void setDiscount(boolean discount) {
this.discount = discount;
}
public boolean isDiscount() {
return discount;
}
public double getTotalPrice() {
double totalPrice = quantity * unitPrice;
if (discount) {
totalPrice *= 0.8; // 如果是打折商品,则总价减八折
}
return totalPrice;
}
// 为了方便程序调试,我们还可以重写toString方法
@Override
public String toString() {
return "Product{" +
"name='" + name + '\'' +
", quantity=" + quantity +
", unitPrice=" + unitPrice +
", discount=" + discount +
'}';
}
}
```
接下来,我们创建一个收银类,用来处理商品的录入和结算。在收银类中,我们可以使用一个动态数组来存储用户输入的商品,并且编写一个计算账单总价的方法。
```java
import java.util.ArrayList;
import java.util.Scanner;
public class Cashier {
private ArrayList<Product> products; // 存储商品的数组
public Cashier() {
products = new ArrayList<>();
}
public void addProduct(Product product) {
products.add(product);
}
public double getTotalPrice() {
double totalPrice = 0;
for (Product product : products) {
totalPrice += product.getTotalPrice();
}
return totalPrice;
}
public void checkout() {
System.out.println("----------------------------------");
System.out.println("商品清单:");
for (Product product : products) {
System.out.println(product.getName() + "\t" + product.getQuantity() + "个\t" +
"¥" + product.getUnitPrice() + "\t总价:¥" + product.getTotalPrice());
}
System.out.println("账单总价:¥" + getTotalPrice());
}
public static void main(String[] args) {
// 创建一个收银实例
Cashier cashier = new Cashier();
// 进行商品录入
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("请输入商品名称:");
String name = scanner.next();
if (name.equals("exit")) {
break;
}
System.out.print("请输入商品数量:");
int quantity = scanner.nextInt();
System.out.print("请输入商品单价:");
double unitPrice = scanner.nextDouble();
System.out.println("请输入打折信息(1表示打折,0表示不打折):");
boolean discount = scanner.nextInt() == 1 ? true : false;
Product product = new Product(name, quantity, unitPrice);
product.setDiscount(discount);
cashier.addProduct(product);
System.out.println("商品添加成功:" + product);
System.out.println();
}
// 进行账单结算
cashier.checkout();
}
}
```
这样,我们就完成了这个程序的编写。当用户输入商品名称、数量和单价时,程序会自动计算出商品总价,并根据是否打折来应用相应的折扣。最后,程序会输出账单总价和商品清单。
请注意:本程序仅供学习和参考,可能存在缺陷和不足之处,实际应用中请根据需要自行调整和完善。
相关推荐
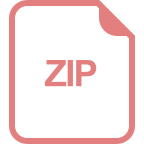














