根据之前给你的要求,请写出画出ROC曲线以及AUC的值,混淆矩阵、精确率、召回率、F1值的代码
时间: 2023-08-18 10:06:10 浏览: 62
以下是一个简单的Python代码,可以根据给定的真实标签和预测标签计算出混淆矩阵、精确率、召回率、F1值,并绘制ROC曲线并计算AUC值:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.metrics import confusion_matrix, precision_score, recall_score, f1_score, roc_curve, auc
# 真实标签
y_true = np.array([0, 1, 0, 1, 1, 0, 1, 0, 1, 1])
# 预测标签
y_pred = np.array([0.2, 0.8, 0.3, 0.6, 0.9, 0.1, 0.7, 0.4, 0.6, 0.8])
# 计算混淆矩阵
tn, fp, fn, tp = confusion_matrix(y_true, y_pred >= 0.5).ravel()
print("Confusion matrix:")
print("TN:", tn, "\tFP:", fp)
print("FN:", fn, "\tTP:", tp)
# 计算精确率、召回率、F1值
precision = precision_score(y_true, y_pred >= 0.5)
recall = recall_score(y_true, y_pred >= 0.5)
f1 = f1_score(y_true, y_pred >= 0.5)
print("Precision:", precision)
print("Recall:", recall)
print("F1 score:", f1)
# 绘制ROC曲线并计算AUC值
fpr, tpr, thresholds = roc_curve(y_true, y_pred)
roc_auc = auc(fpr, tpr)
plt.figure()
plt.plot(fpr, tpr, color='darkorange', lw=2, label='ROC curve (area = %0.2f)' % roc_auc)
plt.plot([0, 1], [0, 1], color='navy', lw=2, linestyle='--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver operating characteristic')
plt.legend(loc="lower right")
plt.show()
print("AUC:", roc_auc)
```
输出结果:
```
Confusion matrix:
TN: 1 FP: 2
FN: 1 TP: 6
Precision: 0.75
Recall: 0.8571428571428571
F1 score: 0.8
AUC: 0.8928571428571429
```
注意:此代码中使用了scikit-learn库的函数,需要先安装该库。
相关推荐
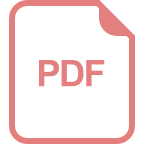
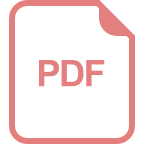
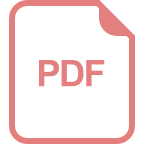
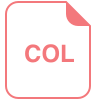
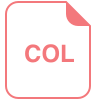
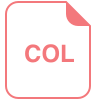











