C#遍历循环列表
时间: 2023-07-25 13:07:28 浏览: 50
在 C# 中,你可以使用 foreach 循环来遍历 List<T> 类型的列表。以下是一个示例代码:
```
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
foreach (int number in numbers)
{
Console.WriteLine(number);
}
```
上述代码中,我们首先创建了一个包含整数的 List 对象 numbers。然后,我们使用 foreach 循环来遍历该列表,并将列表中的每个元素赋值给变量 number。在循环中,我们将每个元素打印到控制台上。
请注意,我们可以使用 foreach 循环遍历任何实现 IEnumerable<T> 接口的对象,因此上述示例代码也适用于其他类型的集合、数组或自定义对象。
相关问题
C#遍历循环列表排序
在 C# 中,可以使用 `List<T>` 类型来表示列表,并使用 `foreach` 循环遍历列表中的元素。要对列表中的元素进行排序,可以使用 `List<T>` 类型的 `Sort()` 方法。
下面是一个示例代码,该代码创建一个包含整数的列表,然后使用 `foreach` 循环遍历该列表并输出其内容,最后使用 `Sort()` 方法对列表进行排序并再次输出其内容:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main(string[] args)
{
List<int> numbers = new List<int>() { 4, 2, 6, 3, 1, 5 };
Console.WriteLine("Original list:");
foreach (int number in numbers)
{
Console.Write(number + " ");
}
Console.WriteLine();
numbers.Sort();
Console.WriteLine("Sorted list:");
foreach (int number in numbers)
{
Console.Write(number + " ");
}
Console.WriteLine();
}
}
```
输出结果:
```
Original list:
4 2 6 3 1 5
Sorted list:
1 2 3 4 5 6
```
C#遍历循环列表冒泡排序
以下是使用冒泡排序算法对 C# 中的循环列表进行排序的示例代码:
```csharp
public class Node<T>
{
public T Value { get; set; }
public Node<T> Next { get; set; }
public Node(T value)
{
Value = value;
}
}
public class LinkedList<T> : IEnumerable<T>
{
private Node<T> _head;
private Node<T> _tail;
private int _count;
public int Count => _count;
public void Add(T value)
{
var node = new Node<T>(value);
if (_head == null)
{
_head = node;
}
else
{
_tail.Next = node;
}
_tail = node;
_count++;
}
public IEnumerator<T> GetEnumerator()
{
var current = _head;
while (current != null)
{
yield return current.Value;
current = current.Next;
}
}
IEnumerator IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
public void BubbleSort()
{
if (_head == null || _head.Next == null)
{
return;
}
var swapped = true;
while (swapped)
{
swapped = false;
var current = _head;
while (current.Next != null)
{
if (Comparer<T>.Default.Compare(current.Value, current.Next.Value) > 0)
{
SwapNodes(current, current.Next);
swapped = true;
}
current = current.Next;
}
}
}
private void SwapNodes(Node<T> node1, Node<T> node2)
{
var temp = node1.Value;
node1.Value = node2.Value;
node2.Value = temp;
}
}
```
在上面的代码中,我们首先定义了一个 `Node<T>` 类来表示列表中的节点,以及一个 `LinkedList<T>` 类来表示循环列表。其中, `Add` 方法用于向列表中添加新元素, `GetEnumerator` 方法用于实现 `IEnumerable<T>` 接口,以便我们可以使用 `foreach` 循环遍历列表中的元素。`BubbleSort` 方法则使用冒泡排序算法对列表中的元素进行排序,最后的 `SwapNodes` 方法用于交换两个节点的值。
要使用上面的代码对循环列表进行冒泡排序,可以按照以下方式操作:
```csharp
var list = new LinkedList<int>();
list.Add(3);
list.Add(1);
list.Add(4);
list.Add(2);
Console.WriteLine("Before sorting:");
foreach (var item in list)
{
Console.Write($"{item} ");
}
list.BubbleSort();
Console.WriteLine("\nAfter sorting:");
foreach (var item in list)
{
Console.Write($"{item} ");
}
```
输出结果如下:
```
Before sorting:
3 1 4 2
After sorting:
1 2 3 4
```
相关推荐
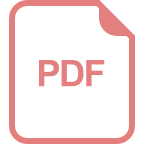
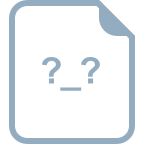
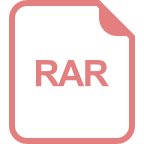












