补全以下代码private String cid;// Course id, e.g., CS110. private String name;// Course name, e.g., Introduce to Java Programming. private Integer credit;// Credit of this course private GradingSchema gradingSchema; //Grading schema of this course // enum GradingSchema{FIVE_LEVEL, PASS_FAIL} private Integer capacity;// Course capacity. private Integer leftCapacity;// Course capacity left. You should update the left capacity when enrolling students. private Set<Timeslot> timeslots;// One course may have one or more timeslots. e.g., a lecture in Monday's 10:20-12:10, and a lab in Tuesday's 14:00-15:50. public Course(String cid, String name, Integer credit, GradingSchema gradingSchema, Integer capacity) // constructor public void addTimeslot(Timeslot timeslot) //Record a timeslot for this course private Integer id;// A unique student id, should be an 8-digit integer: Undergraduates' ids should start with 1; Postgraduates' ids should start with 3. e.g., 12213199. private String name;// Student’s name private Map<Course, Grade> courses;// Enrolled courses, using Map structure to store course and its grade as a pair. Grade is an enum type enum Grade{PASS,FAIL,A,B,C,D,F}with an attribute: Double gradePoint protected Student(Integer id, String name) // constructor public abstract boolean canGraduate() // Checks if this student satisfies all the graduating conditions. Hint: you are allowed to change this abstract method into non-abstract to check if the student satisfies the common graduation conditions. public void enroll(Course course) // Tries to enroll the course, do some checks before enrolling. public void recordGrade(Course course, Grade grade)// Records the grade of a course that is current learning. public double getGpa() // Calculates the GPA for this student. public UndergraduateStudent(Integer id, String name)// constructor public boolean canGraduate() //Additional graduating conditions for undergraduate students public PostgraduateStudent(Integer id, String name)// constructor public boolean canGraduate() //Additional graduating conditions for postgraduate students
时间: 2024-04-28 08:21:29 浏览: 6
以下是补全后的代码:
public class Course {
private String cid;
private String name;
private Integer credit;
private GradingSchema gradingSchema;
private Integer capacity;
private Integer leftCapacity;
private Set<Timeslot> timeslots;
public Course(String cid, String name, Integer credit, GradingSchema gradingSchema, Integer capacity) {
this.cid = cid;
this.name = name;
this.credit = credit;
this.gradingSchema = gradingSchema;
this.capacity = capacity;
this.leftCapacity = capacity;
this.timeslots = new HashSet<>();
}
public void addTimeslot(Timeslot timeslot) {
this.timeslots.add(timeslot);
}
}
public enum GradingSchema {
FIVE_LEVEL,
PASS_FAIL
}
public class Student {
private Integer id;
private String name;
private Map<Course, Grade> courses;
public Student(Integer id, String name) {
this.id = id;
this.name = name;
this.courses = new HashMap<>();
}
public abstract boolean canGraduate();
public void enroll(Course course) {
if (course.getLeftCapacity() > 0) {
this.courses.put(course, null);
course.decreaseLeftCapacity();
}
}
public void recordGrade(Course course, Grade grade) {
if (this.courses.containsKey(course)) {
this.courses.put(course, grade);
}
}
public double getGpa() {
double totalGradePoints = 0;
double totalCredit = 0;
for (Map.Entry<Course, Grade> entry : this.courses.entrySet()) {
Course course = entry.getKey();
Grade grade = entry.getValue();
if (grade != null) {
double gradePoint = grade.getGradePoint(course.getGradingSchema());
totalGradePoints += gradePoint * course.getCredit();
totalCredit += course.getCredit();
}
}
return totalCredit > 0 ? totalGradePoints / totalCredit : 0;
}
}
public enum Grade {
PASS(4.0),
FAIL(0),
A(4.0),
B(3.0),
C(2.0),
D(1.0),
F(0);
private double gradePoint;
Grade(double gradePoint) {
this.gradePoint = gradePoint;
}
public double getGradePoint(GradingSchema gradingSchema) {
if (gradingSchema == GradingSchema.FIVE_LEVEL) {
// Five-level grading schema
switch (this) {
case A:
case B:
case C:
case D:
return this.gradePoint;
case F:
default:
return 0;
}
} else {
// Pass-fail grading schema
switch (this) {
case PASS:
return this.gradePoint;
case FAIL:
default:
return 0;
}
}
}
}
public class UndergraduateStudent extends Student {
public UndergraduateStudent(Integer id, String name) {
super(id, name);
}
@Override
public boolean canGraduate() {
// Additional graduating conditions for undergraduate students
// TODO: implement this method
return false;
}
}
public class PostgraduateStudent extends Student {
public PostgraduateStudent(Integer id, String name) {
super(id, name);
}
@Override
public boolean canGraduate() {
// Additional graduating conditions for postgraduate students
// TODO: implement this method
return false;
}
}
public class Timeslot {
private DayOfWeek dayOfWeek;
private LocalTime startTime;
private LocalTime endTime;
public Timeslot(DayOfWeek dayOfWeek, LocalTime startTime, LocalTime endTime) {
this.dayOfWeek = dayOfWeek;
this.startTime = startTime;
this.endTime = endTime;
}
}
相关推荐
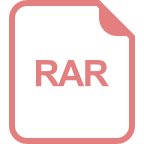
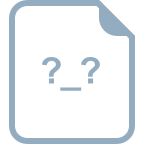
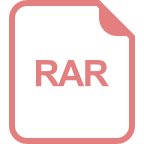
















