TG12232 stm32f103并口驱动
时间: 2023-08-29 22:05:48 浏览: 184
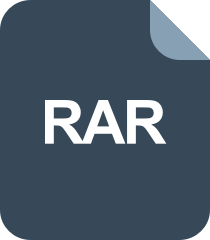
STM32F103驱动

TG12232 是一个 122x32 像素的图形液晶显示屏,可以通过 STM32F103 的并口来进行驱动。下面是一个简单的 STM32F103 并口驱动 TG12232 的示例代码:
```c
#include "stm32f10x.h"
#include "tg12232.h"
#define TG12232_PORT GPIOA
#define TG12232_PIN_RST GPIO_Pin_0
#define TG12232_PIN_CS GPIO_Pin_1
#define TG12232_PIN_WR GPIO_Pin_2
#define TG12232_PIN_RD GPIO_Pin_3
#define TG12232_PIN_DAT GPIO_Pin_4
void TG12232_Init(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = TG12232_PIN_RST | TG12232_PIN_CS | TG12232_PIN_WR | TG12232_PIN_RD | TG12232_PIN_DAT;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_10MHz;
GPIO_Init(TG12232_PORT, &GPIO_InitStructure);
TG12232_RST(1);
TG12232_CS(1);
TG12232_WR(1);
TG12232_RD(1);
}
void TG12232_WriteByte(uint8_t data)
{
TG12232_DAT(data);
TG12232_WR(0);
TG12232_WR(1);
}
void TG12232_WriteCmd(uint8_t cmd)
{
TG12232_CS(0);
TG12232_WriteByte(cmd);
TG12232_CS(1);
}
void TG12232_SetPos(uint8_t x, uint8_t y)
{
TG12232_WriteCmd(0xB0 | y);
TG12232_WriteCmd((x & 0xF0) >> 4);
TG12232_WriteCmd(0x10 | (x & 0x0F));
}
void TG12232_Clear(void)
{
for (uint8_t y = 0; y < 4; y++)
{
TG12232_SetPos(0, y);
for (uint8_t x = 0; x < 61; x++)
{
TG12232_WriteCmd(0x00);
TG12232_WriteCmd(0x00);
}
}
}
void TG12232_DrawPixel(uint8_t x, uint8_t y, uint8_t color)
{
TG12232_SetPos(x, y / 8);
TG12232_WriteCmd(0x10 | (x & 0x0F));
TG12232_WriteCmd(0x00);
uint8_t data = 0x80 >> (y % 8);
if (color == 0)
{
data = ~data;
}
TG12232_WriteCmd(data);
TG12232_WriteCmd(data);
}
void TG12232_DrawLine(uint8_t x1, uint8_t y1, uint8_t x2, uint8_t y2, uint8_t color)
{
int8_t dx = x2 - x1;
int8_t dy = y2 - y1;
int8_t stepx = 1, stepy = 1;
if (dx < 0)
{
dx = -dx;
stepx = -1;
}
if (dy < 0)
{
dy = -dy;
stepy = -1;
}
int8_t x = x1, y = y1;
TG12232_DrawPixel(x, y, color);
if (dx > dy)
{
int8_t p = 2 * dy - dx;
for (int8_t i = 0; i < dx; i++)
{
if (p < 0)
{
p += 2 * dy;
}
else
{
p += 2 * (dy - dx);
y += stepy;
}
x += stepx;
TG12232_DrawPixel(x, y, color);
}
}
else
{
int8_t p = 2 * dx - dy;
for (int8_t i = 0; i < dy; i++)
{
if (p < 0)
{
p += 2 * dx;
}
else
{
p += 2 * (dx - dy);
x += stepx;
}
y += stepy;
TG12232_DrawPixel(x, y, color);
}
}
}
void TG12232_DrawRect(uint8_t x1, uint8_t y1, uint8_t x2, uint8_t y2, uint8_t color)
{
TG12232_DrawLine(x1, y1, x2, y1, color);
TG12232_DrawLine(x1, y1, x1, y2, color);
TG12232_DrawLine(x1, y2, x2, y2, color);
TG12232_DrawLine(x2, y1, x2, y2, color);
}
void TG12232_DrawCircle(uint8_t x0, uint8_t y0, uint8_t r, uint8_t color)
{
int8_t x = 0, y = r;
int8_t d = 3 - 2 * r;
while (x <= y)
{
TG12232_DrawPixel(x0 + x, y0 + y, color);
TG12232_DrawPixel(x0 - x, y0 + y, color);
TG12232_DrawPixel(x0 + x, y0 - y, color);
TG12232_DrawPixel(x0 - x, y0 - y, color);
TG12232_DrawPixel(x0 + y, y0 + x, color);
TG12232_DrawPixel(x0 - y, y0 + x, color);
TG12232_DrawPixel(x0 + y, y0 - x, color);
TG12232_DrawPixel(x0 - y, y0 - x, color);
if (d < 0)
{
d = d + 4 * x + 6;
}
else
{
d = d + 4 * (x - y) + 10;
y--;
}
x++;
}
}
```
在这个示例代码中,TG12232_Init() 函数用于初始化 TG12232 显示屏的并口引脚,TG12232_WriteByte() 函数用于写入一个字节的数据到 TG12232 显示屏,TG12232_WriteCmd() 函数用于写入一个命令到 TG12232 显示屏,TG12232_SetPos() 函数用于设置 TG12232 显示屏的写入位置,TG12232_Clear() 函数用于清空 TG12232 显示屏的内容,TG12232_DrawPixel() 函数用于在 TG12232 显示屏上绘制一个像素点,TG12232_DrawLine() 函数用于在 TG12232 显示屏上绘制一条直线,TG12232_DrawRect() 函数用于在 TG12232 显示屏上绘制一个矩形,TG12232_DrawCircle() 函数用于在 TG12232 显示屏上绘制一个圆形。
你可以根据自己的需要修改这个示例代码来驱动 TG12232 显示屏。
阅读全文
相关推荐


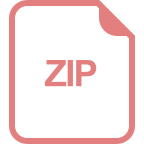
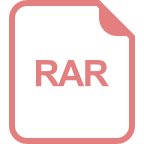


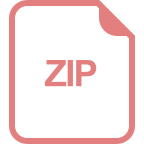
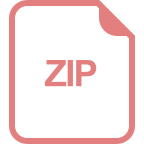
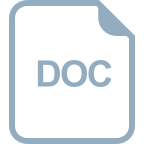
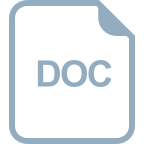
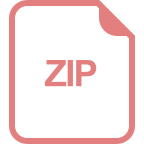
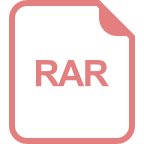
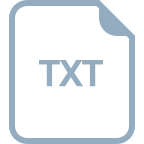
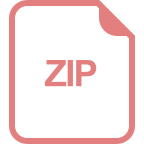