public static ICollection<IGrouping<long, HullInfo>> GetAllHullInfoByTimes(long startTime, long endTime) { using (var context = new DataBaseContext()) { var Hulls = context.Hulls.Where(t => t.MeasureTime >= startTime && t.MeasureTime <= endTime) .GroupBy(s => s.MeasureTime).ToList(); return Hulls; } }如何调用结果
时间: 2024-01-15 16:03:02 浏览: 29
你可以在你的代码中调用这个方法,并传入起始时间和结束时间作为参数,如下所示:
```
long startTime = 1614556800; // 2021/3/1 00:00:00 的 Unix 时间戳
long endTime = 1617235199; // 2021/3/31 23:59:59 的 Unix 时间戳
var hullInfoByTimes = GetAllHullInfoByTimes(startTime, endTime);
foreach (var hullGroup in hullInfoByTimes)
{
Console.WriteLine($"Measure Time: {hullGroup.Key}");
foreach (var hullInfo in hullGroup)
{
Console.WriteLine($"Hull Info: {hullInfo}");
}
}
```
这个方法将返回一个 `ICollection<IGrouping<long, HullInfo>>` 类型的集合,其中每个元素都是一个 `IGrouping<long, HullInfo>` 对象,该对象表示按测量时间分组的船体信息。你可以使用 `foreach` 循环遍历这个集合,并获取每个分组的测量时间和相应的船体信息。
相关问题
如何将 ICollection<IGrouping<long, HullInfo>>绑定到datagridview
要将 `ICollection<IGrouping<long, HullInfo>>` 绑定到 `DataGridView`,需要先将其转换为可绑定的数据源。以下是一种方法:
1.创建一个新的 `DataTable` 实例。
2.循环遍历 `ICollection<IGrouping<long, HullInfo>>`,将其中的数据添加到 `DataTable` 中。可以使用 `foreach` 循环遍历 `ICollection<IGrouping<long, HullInfo>>` 中的每个 `IGrouping`,然后使用嵌套的 `foreach` 循环遍历 `IGrouping` 中的每个 `HullInfo`,并将其添加到 `DataTable` 中。
3.将 `DataTable` 绑定到 `DataGridView` 的 `DataSource` 属性上。
以下是示例代码:
```
ICollection<IGrouping<long, HullInfo>> data = GetData(); // 从数据源获取数据
// 创建 DataTable
DataTable table = new DataTable();
table.Columns.Add("GroupId", typeof(long));
table.Columns.Add("HullInfoId", typeof(long));
table.Columns.Add("Name", typeof(string));
table.Columns.Add("Length", typeof(double));
table.Columns.Add("Width", typeof(double));
// 将数据添加到 DataTable
foreach (var group in data)
{
foreach (var hullInfo in group)
{
DataRow row = table.NewRow();
row["GroupId"] = group.Key;
row["HullInfoId"] = hullInfo.Id;
row["Name"] = hullInfo.Name;
row["Length"] = hullInfo.Length;
row["Width"] = hullInfo.Width;
table.Rows.Add(row);
}
}
// 将 DataTable 绑定到 DataGridView
dataGridView.DataSource = table;
```
ICollection<IGrouping<long, HullInfo>>如何绑定到dataGrieView的一列中
要将 `ICollection<IGrouping<long, HullInfo>>` 绑定到 `DataGridView` 的一列中,可以使用自定义列的方式。以下是一种方法:
1.创建一个继承自 `DataGridViewColumn` 的自定义列类,例如 `GroupingColumn`,并重写其中的 `CellTemplate` 属性,指定单元格模板为自定义的 `GroupingCell` 类。
```csharp
public class GroupingColumn : DataGridViewColumn
{
public GroupingColumn()
{
// 设置列的属性
HeaderText = "Grouping";
CellTemplate = new GroupingCell();
}
}
public class GroupingCell : DataGridViewTextBoxCell
{
public override object FormattedValue
{
get
{
// 获取单元格的值,并将其转换为 IGrouping<long, HullInfo>
var grouping = (IGrouping<long, HullInfo>)Value;
// 返回 IGrouping<long, HullInfo> 的 Key 值
return grouping.Key;
}
}
}
```
2.将自定义列添加到 `DataGridView` 的 `Columns` 集合中。
```csharp
// 创建 DataGridView
var dataGridView = new DataGridView();
// 添加自定义列
var groupingColumn = new GroupingColumn();
dataGridView.Columns.Add(groupingColumn);
// 绑定数据源
var data = GetData(); // 从数据源获取数据
dataGridView.DataSource = data;
```
这样,`ICollection<IGrouping<long, HullInfo>>` 中的每个 `IGrouping<long, HullInfo>` 就会显示在 `DataGridView` 的 `GroupingColumn` 列中,并且单元格的值为 `IGrouping<long, HullInfo>` 的 Key 值。
相关推荐
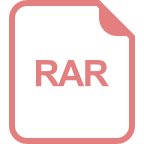
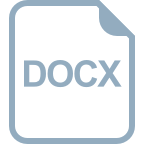
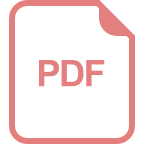












