resttemplate 请求post 发送json参数
时间: 2023-11-14 16:59:25 浏览: 90
使用 RestTemplate 发送 POST 请求并发送 JSON 参数的示例代码如下:
```
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
JSONObject requestBody = new JSONObject();
requestBody.put("key1", "value1");
requestBody.put("key2", "value2");
HttpEntity<String> request = new HttpEntity<String>(requestBody.toString(), headers);
String url = "http://example.com/api/endpoint";
ResponseEntity<String> response = restTemplate.postForEntity(url, request, String.class);
```
其中,`HttpHeaders` 设置请求头信息,`JSONObject` 构造请求体 JSON 对象,`HttpEntity` 将请求体和请求头组合成一个请求实体对象,`RestTemplate` 发送 POST 请求并返回响应结果。
相关问题
resttemplate post请求发送json数据
您可以使用RestTemplate的postForObject方法发送POST请求并发送JSON数据。下面是一个示例代码:
```java
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
public class RestClient {
public static void main(String[] args) {
// 创建RestTemplate实例
RestTemplate restTemplate = new RestTemplate();
// 设置请求头,指定Content-Type为application/json
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
// 设置请求体,即要发送的JSON数据
String jsonBody = "{\"key\": \"value\"}";
// 创建HttpEntity对象,包含请求头和请求体
HttpEntity<String> requestEntity = new HttpEntity<>(jsonBody, headers);
// 发送POST请求并获取响应
String url = "http://example.com/api/endpoint"; // 请求的URL
String response = restTemplate.postForObject(url, requestEntity, String.class);
// 处理响应
System.out.println(response);
}
}
```
在上面的代码中,您需要将`http://example.com/api/endpoint`替换为实际的目标URL。另外,您可以根据需要调整JSON请求体的内容。
resttemplate.postForEntity发送json数据
您可以使用RestTemplate的postForEntity方法来发送JSON数据。下面是示例代码:
```java
import org.springframework.http.*;
import org.springframework.web.client.*;
// 创建RestTemplate对象
RestTemplate restTemplate = new RestTemplate();
// 设置请求头,指定Content-Type为application/json
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
// 创建请求体,即要发送的JSON数据
String jsonBody = "{\"key1\": \"value1\", \"key2\": \"value2\"}";
// 创建HttpEntity对象,封装请求头和请求体
HttpEntity<String> requestEntity = new HttpEntity<>(jsonBody, headers);
// 发送POST请求,获取响应
String url = "http://example.com/api/endpoint";
ResponseEntity<String> response = restTemplate.postForEntity(url, requestEntity, String.class);
// 获取响应状态码和响应体
HttpStatus statusCode = response.getStatusCode();
String responseBody = response.getBody();
```
在上面的示例中,我们首先创建了一个RestTemplate对象,然后设置了请求头,指定Content-Type为application/json。接下来,我们创建了一个请求体,即要发送的JSON数据。然后,将请求头和请求体封装到HttpEntity对象中。最后,使用postForEntity方法发送POST请求,并获取响应的状态码和响应体。
请注意替换示例代码中的URL为您实际要发送请求的URL,并根据您的实际需求修改请求体和响应处理逻辑。
阅读全文
相关推荐
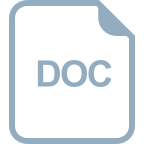
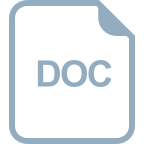
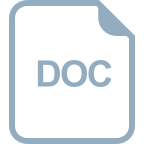

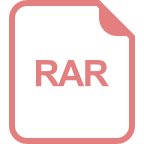










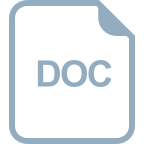
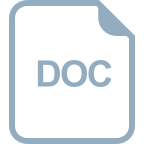