C++ iso8122读取 .dbf和.sbn、shx sho abx文件 加水印 输出到其他文件中
时间: 2024-02-05 21:10:28 浏览: 128
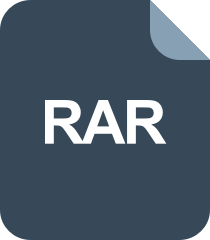
api.rar_ dbf_.shx_autocad_shapfile_shx 文件
可以使用以下库来读取和处理.dbf、.sbn、.shx和.abx文件:
1. libdbf - 用于读取和处理.dbf文件的C++库。
2. ShapeLib - 用于读取和处理.shp、.shx和.dbf文件的C++库。
3. libSHP - 用于读取和处理.shp、.shx和.dbf文件的C++库。
4. MapServer - 用于读取和处理.shp、.sbn、.shx和.abx文件的C++库。
要加水印并输出到其他文件,您可以使用以下步骤:
1. 从原始文件中读取数据。
2. 在内存中对数据进行处理,加入水印。
3. 将处理后的数据写入新文件中。
以下是代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include "libdbf/dbf_file.h"
#include "ShapeLib/shapefil.h"
int main()
{
// 读取.dbf文件
DBFHandle hDBF = DBFOpen("data.dbf", "rb");
if (hDBF == nullptr)
{
std::cerr << "Error: Unable to open data.dbf" << std::endl;
return 1;
}
int nRecords = DBFGetRecordCount(hDBF);
int nFields = DBFGetFieldCount(hDBF);
// 读取.shp、.shx和.dbf文件
SHPHandle hSHP = SHPOpen("data.shp", "rb");
if (hSHP == nullptr)
{
std::cerr << "Error: Unable to open data.shp" << std::endl;
return 1;
}
// 创建输出文件
DBFHandle hDBFOut = DBFCreate("data_out.dbf");
if (hDBFOut == nullptr)
{
std::cerr << "Error: Unable to create data_out.dbf" << std::endl;
return 1;
}
SHPHandle hSHPOut = SHPCreate("data_out.shp", SHPT_POINT);
if (hSHPOut == nullptr)
{
std::cerr << "Error: Unable to create data_out.shp" << std::endl;
return 1;
}
// 复制.dbf字段
for (int i = 0; i < nFields; i++)
{
const char* pszFieldName = DBFGetFieldName(hDBF, i);
DBFFieldType eType = DBFGetFieldInfo(hDBF, i, nullptr, nullptr);
int nWidth = DBFGetFieldWidth(hDBF, i);
int nDecimals = DBFGetFieldDecimals(hDBF, i);
if (DBFAddField(hDBFOut, pszFieldName, eType, nWidth, nDecimals) == -1)
{
std::cerr << "Error: Unable to add field " << pszFieldName << std::endl;
return 1;
}
}
// 处理.shp文件
for (int i = 0; i < nRecords; i++)
{
SHPObject* pSHP = SHPReadObject(hSHP, i);
if (pSHP == nullptr)
{
std::cerr << "Error: Unable to read object " << i << std::endl;
return 1;
}
// 在内存中添加水印
std::vector<double> adfX(pSHP->nVertices);
std::vector<double> adfY(pSHP->nVertices);
for (int j = 0; j < pSHP->nVertices; j++)
{
adfX[j] = pSHP->padfX[j];
adfY[j] = pSHP->padfY[j];
}
// TODO: 在坐标中添加水印
// 在新文件中写入.shp对象
SHPObject* pSHPOut = SHPCreateObject(pSHP->nSHPType, pSHP->nShapeId, pSHP->nParts, pSHP->panStart, pSHP->panLength, pSHP->nVertices, &adfX[0], &adfY[0], nullptr, nullptr);
if (pSHPOut == nullptr)
{
std::cerr << "Error: Unable to create output object " << i << std::endl;
return 1;
}
if (SHPWriteObject(hSHPOut, -1, pSHPOut) == -1)
{
std::cerr << "Error: Unable to write output object " << i << std::endl;
return 1;
}
SHPDestroyObject(pSHPOut);
// 在新文件中写入.dbf记录
for (int j = 0; j < nFields; j++)
{
const char* pszFieldName = DBFGetFieldName(hDBF, j);
DBFFieldType eType = DBFGetFieldInfo(hDBF, j, nullptr, nullptr);
int nWidth = DBFGetFieldWidth(hDBF, j);
int nDecimals = DBFGetFieldDecimals(hDBF, j);
switch (eType)
{
case FTString:
{
const char* pszValue = DBFReadStringAttribute(hDBF, i, j);
if (DBFWriteStringAttribute(hDBFOut, i, j, pszValue) == false)
{
std::cerr << "Error: Unable to write string attribute " << pszFieldName << std::endl;
return 1;
}
break;
}
case FTInteger:
{
int nValue = DBFReadIntegerAttribute(hDBF, i, j);
if (DBFWriteIntegerAttribute(hDBFOut, i, j, nValue) == false)
{
std::cerr << "Error: Unable to write integer attribute " << pszFieldName << std::endl;
return 1;
}
break;
}
case FTDouble:
{
double dfValue = DBFReadDoubleAttribute(hDBF, i, j);
if (DBFWriteDoubleAttribute(hDBFOut, i, j, dfValue) == false)
{
std::cerr << "Error: Unable to write double attribute " << pszFieldName << std::endl;
return 1;
}
break;
}
case FTLogical:
{
bool bValue = DBFReadLogicalAttribute(hDBF, i, j);
if (DBFWriteLogicalAttribute(hDBFOut, i, j, bValue) == false)
{
std::cerr << "Error: Unable to write logical attribute " << pszFieldName << std::endl;
return 1;
}
break;
}
default:
break;
}
}
}
// 关闭文件
DBFClose(hDBF);
SHPClose(hSHP);
DBFClose(hDBFOut);
SHPClose(hSHPOut);
return 0;
}
```
请注意,此示例仅用于演示如何读取和处理这些文件。要添加水印,您需要编写自己的代码来修改内存中的数据。
阅读全文
相关推荐
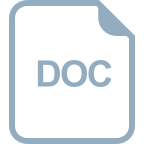
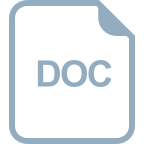


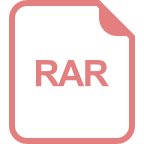
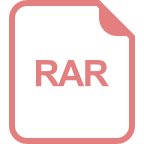
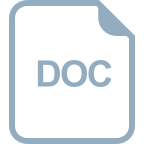
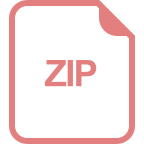
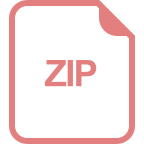
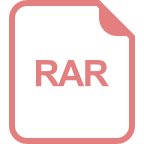
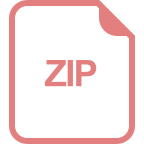
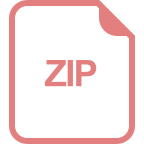
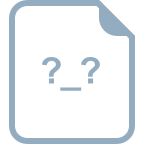
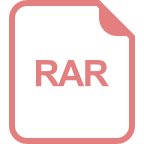
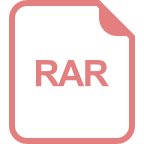
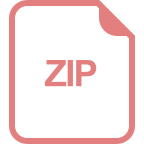