population, objectives = moea(100, 100)、parent1 = population[random.randint(0, pop_size-1)]报错“list index out of range”
时间: 2024-05-26 15:14:39 浏览: 13
这个错误通常出现在尝试访问一个列表中不存在的索引时。可能是在使用 `population` 列表或 `parent1` 变量时,索引参数超出了列表的范围,导致出现了该错误。
请检查一下你的代码,确保你的 `population` 列表至少有 `pop_size` 个元素,并且 `random.randint(0, pop_size-1)` 的范围不超出 `population` 列表的索引范围。如果 `population` 列表中没有足够的元素,你可以尝试增加种群大小或更改随机数范围。
此外,如果你的代码中使用了其他列表或索引,也请检查它们是否出现了类似的错误。
相关问题
objectives = np.random.rand(10, 2)
objectives = np.random.rand(10, 2) 是一个使用NumPy库生成的一个10行2列的二维数组。np.random.rand()函数用于生成指定形状的随机数组,其中的元素是在[0, 1)范围内均匀分布的随机数。
这个二维数组可以表示为一个包含10个数据点的数据集,每个数据点有两个特征。每个特征的值都是在0到1之间的随机数。
import numpy as np from platypus import NSGAII, Problem, Real, Integer # 定义问题 class JobShopProblem(Problem): def __init__(self, jobs, machines, processing_times): num_jobs = len(jobs) num_machines = len(machines[0]) super().__init__(num_jobs, 1, 1) self.jobs = jobs self.machines = machines self.processing_times = processing_times self.types[:] = Integer(0, num_jobs - 1) self.constraints[:] = [lambda x: x[0] == 1] def evaluate(self, solution): job_order = np.argsort(np.array(solution.variables[:], dtype=int)) machine_available_time = np.zeros(len(self.machines)) job_completion_time = np.zeros(len(self.jobs)) for job_idx in job_order: job = self.jobs[job_idx] for machine_idx, processing_time in zip(job, self.processing_times[job_idx]): machine_available_time[machine_idx] = max(machine_available_time[machine_idx], job_completion_time[job_idx]) job_completion_time[job_idx] = machine_available_time[machine_idx] + processing_time solution.objectives[:] = [np.max(job_completion_time)] # 定义问题参数 jobs = [[0, 1], [2, 0], [1, 2]] machines = [[0, 1, 2], [1, 2, 0], [2, 0, 1]] processing_times = [[5, 4], [3, 5], [1, 3]] # 创建算法实例 problem = JobShopProblem(jobs, machines, processing_times) algorithm = NSGAII(problem) algorithm.population_size = 100 # 设置优化目标 problem.directions[:] = Problem.MINIMIZE # 定义算法参数 algorithm.population_size = 100 max_generations = 100 mutation_probability = 0.1 # 设置算法参数 algorithm.max_iterations = max_generations algorithm.mutation_probability = mutation_probability # 运行算法 algorithm.run(max_generations) # 输出结果 print("最小化的最大完工时间:", algorithm.result[0].objectives[0]) print("工件加工顺序和机器安排方案:", algorithm.result[0].variables[:]) 请检查上述代码
代码的语法没有问题,但是在定义问题时,约束条件中的 lambda 函数有误。应该将 x[0] == 1 改为 x[0] == 0,因为变量的范围是 0 到 num_jobs - 1。另外,应该在 lambda 函数前加上 @staticmethod,表示这是一个静态方法。修改后的代码如下:
```
import numpy as np
from platypus import NSGAII, Problem, Real, Integer
# 定义问题
class JobShopProblem(Problem):
def __init__(self, jobs, machines, processing_times):
num_jobs = len(jobs)
num_machines = len(machines[0])
super().__init__(num_jobs, 1, 1)
self.jobs = jobs
self.machines = machines
self.processing_times = processing_times
self.types[:] = Integer(0, num_jobs - 1)
self.constraints[:] = [self.check_order]
@staticmethod
def check_order(x):
return x[0] == 0
def evaluate(self, solution):
job_order = np.argsort(np.array(solution.variables[:], dtype=int))
machine_available_time = np.zeros(len(self.machines))
job_completion_time = np.zeros(len(self.jobs))
for job_idx in job_order:
job = self.jobs[job_idx]
for machine_idx, processing_time in zip(job, self.processing_times[job_idx]):
machine_available_time[machine_idx] = max(machine_available_time[machine_idx], job_completion_time[job_idx])
job_completion_time[job_idx] = machine_available_time[machine_idx] + processing_time
solution.objectives[:] = [np.max(job_completion_time)]
# 定义问题参数
jobs = [[0, 1], [2, 0], [1, 2]]
machines = [[0, 1, 2], [1, 2, 0], [2, 0, 1]]
processing_times = [[5, 4], [3, 5], [1, 3]]
# 创建算法实例
problem = JobShopProblem(jobs, machines, processing_times)
algorithm = NSGAII(problem)
algorithm.population_size = 100
# 设置优化目标
problem.directions[:] = Problem.MINIMIZE
# 定义算法参数
algorithm.population_size = 100
max_generations = 100
mutation_probability = 0.1
# 设置算法参数
algorithm.max_iterations = max_generations
algorithm.mutation_probability = mutation_probability
# 运行算法
algorithm.run(max_generations)
# 输出结果
print("最小化的最大完工时间:", algorithm.result[0].objectives[0])
print("工件加工顺序和机器安排方案:", algorithm.result[0].variables[:])
```
相关推荐
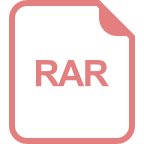












