#include <stdio.h>#include <string.h>void fsort(char *color[], int n) { for (int i = 0; i < n - 1; i++) { for (int j = 0; j < n - i - 1; j++) { if (strcmp(color[j], color[j + 1]) > 0) { // 交换指针 char *temp = color[j]; color[j] = color[j + 1]; color[j + 1] = temp; } } }}int main() { char *colors[5] = {"red", "blue", "green", "yellow", "black"}; fsort(colors, 5); for (int i = 0; i < 5; i++) { printf("%s ", colors[i]); } printf("\n"); return 0;}
时间: 2024-01-11 13:03:31 浏览: 182
这段代码和我之前给出的答案是一样的,它实现了一个按照字符串字典序排序的函数 `fsort()`,并在 `main()` 函数中使用了这个函数对一个字符串数组进行排序,最后输出排序后的结果。
代码中的 `fsort()` 函数使用了冒泡排序算法进行排序,它的参数包括一个指向指针数组的指针和数组中元素的个数。在函数内部,我们使用两重循环遍历数组,并通过 `strcmp()` 函数比较字符串的大小关系,如果需要交换,则交换指针即可。
在 `main()` 函数中,我们定义了一个指针数组 `colors`,其中每个元素指向一个字符串常量。然后我们调用 `fsort()` 函数对它进行排序,并输出结果。
这段代码的输出结果是:
```
black blue green red yellow
```
这正是我们希望得到的按照字典序排序后的字符串数组。
相关问题
#include <stdio.h> #include <string.h> void fsort(char *color[ ], int n); int main(void ) { int i; char *pcolor[ ] = {"red", "blue", "yellow", "green", "black"}; void fsort(char *color[ ], int n); fsort(pcolor, 5); /* 调用函数 */ for(i = 0; i < 5; i++) printf("%s ", pcolor[i]); printf("\n"); return 0; } /* 请在这里填写答案 */
这段代码实现了一个字符串数组按照字典序进行排序的功能。函数fsort的定义应该如下:
```c
void fsort(char *color[], int n) {
int i, j;
char *temp;
for (i = 0; i < n - 1; i++) {
for (j = i + 1; j < n; j++) {
if (strcmp(color[i], color[j]) > 0) {
temp = color[i];
color[i] = color[j];
color[j] = temp;
}
}
}
}
```
其中,color是一个字符串指针数组,n是数组元素个数。在函数中,我们使用了双重循环对所有元素进行比较,并且使用了strcmp函数进行字符串比较。如果前一个元素大于后一个元素,则进行交换。最后,函数会将排好序的字符串数组返回给调用者。
#include <stdio.h> #include <malloc.h> #include <string.h> #define N 10 void GetMemory(char **ptr, i
你好,这个代码片段是在C语言中定义的,它包含了三个常用的头文件`stdio.h`, `malloc.h`, 和 `string.h`。特别地,`#define N 10` 定义了一个名为 N 的宏,其值为10,这通常用于表示数组或字符串的最大长度。
`void GetMemory(char **ptr, int n)` 函数声明了一个名为 GetMemory 的函数,接受两个参数:一个指向字符型指针的指针 `char **ptr` 和一个整数 `int n`。这种双星号是指向指针的指针,意味着 `ptr` 可以改变其指向的内容,可能是动态分配的内存。函数的作用很可能是为了动态分配一个大小为 n 的字符数组,并将其地址赋给 `*ptr`。
举个例子,你可能会这么调用这个函数:
```c
char *str;
GetMemory(&str, N); // 分配一个长度为 N 的字符数组并将其地址赋给 str
```
然后你可以通过 `str` 来操作这块动态分配的内存。然而,由于代码未提供完整实现,这部分细节并未给出。完整的函数实现应包括对内存的分配(如 `malloc(n * sizeof(char))`),以及释放内存的操作(如 `free(ptr)`)。
阅读全文
相关推荐
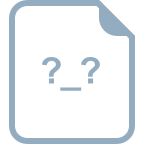
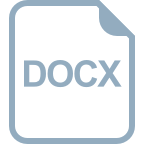
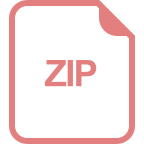












