sprintf_s(expr + strlen(expr), "%.2lf\n", num2);要怎么改对,在vs里
时间: 2024-03-22 22:40:11 浏览: 13
在VS中,`sprintf_s`函数相比于`sprintf`函数更加安全,它需要额外传入一个字符串的大小参数,用于限制输出的字符串长度,避免发生缓冲区溢出的问题。
因此,你可以将原来的`sprintf`函数替换成`sprintf_s`函数,并将第一个参数修改为字符串的长度减去已经输出的字符数。具体的修改如下:
```c
int len = strlen(expr);
sprintf_s(expr + len, sizeof(expr) - len, "%.2lf\n", num2);
```
其中,`len`变量保存了当前`expr`字符串中已经输出的字符数,`sizeof(expr) - len`表示还剩下的字符串空间大小,`%.2lf\n`表示输出一个保留两位小数的浮点数并在末尾加上换行符。
相关问题
用C语言:输入格式,第一行为数值 i,接着的 i 行为需要输入的算式,每行可能有三个数据或两个数据。若该行为三个数据则第一个数据表示运算类型,a 表示加法运算,b 表示减法运算,c 表示乘法运算,接着的两个数据表示参加运算的运算数。若该行为两个数据,则表示本题的运算类型与上一题的运算类型相同,而这两个数据为运算数。输出格式,输出2×i 行。对于每个输入的算式,输出完整的运算式及结果,第二行输出该运算式的总长度
以下是用C语言实现的代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
int i, j, k, len, op_type = 0;
double num1, num2, result;
char op, expr[100];
scanf("%d", &i);
for (j = 0; j < i; j++) {
scanf("%lf %lf", &num1, &num2);
if (j != 0) {
op = op_type == 1 ? '+' : (op_type == 2 ? '-' : '*');
sprintf(expr, "%.2lf %c %.2lf = ", num1, op, num2);
} else {
sprintf(expr, "%.2lf", num1);
}
if (scanf(" %c", &op) == 1) {
op_type = (op == 'a') ? 1 : ((op == 'b') ? 2 : 3);
if (op_type == 1) {
result = num1 + num2;
} else if (op_type == 2) {
result = num1 - num2;
} else {
result = num1 * num2;
}
sprintf(expr + strlen(expr), "%.2lf\n", num2);
} else {
result = num1;
strcat(expr, "\n");
}
len = strlen(expr);
printf("%s%d\n", expr, len);
printf("%.2lf\n", result);
}
return 0;
}
```
程序的思路是先读入需要计算的算式的个数 i,然后进行 i 次循环,每次读入一个算式。在读入算式时,根据上一个算式的运算类型以及当前算式的数据个数来判断本次的运算类型,然后进行相应的计算,计算结果保存在 result 变量中,并将完整的运算式及其结果输出。
注意,为了方便计算字符串长度,我们在输出完整的运算式时还会输出其长度,这个长度包括了运算式及其结果的长度。
程序中用到了 sprintf 函数和 strcat 函数,它们可以帮助我们将字符串拼接起来。另外,我们在读入运算符时使用了 scanf 函数的返回值来判断是否成功读入了一个字符。
利用算法Evaluate Expression- reduced对算数表达式3*(7-2)求值,用c语言写出来
以下是利用 C 语言实现 Evaluate Expression- reduced 算法对算数表达式3*(7-2)求值的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_EXPR_LEN 100 // 算数表达式的最大长度
#define MAX_STACK_LEN 100 // 栈的最大长度
// 定义运算符的优先级
int precedence(char op) {
switch (op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
// 将算数表达式转化为后缀表达式
void infix_to_postfix(char *infix, char *postfix) {
int i, j, len;
char op, stack[MAX_STACK_LEN];
len = strlen(infix);
j = 0;
for (i = 0; i < len; i++) {
if (infix[i] >= '0' && infix[i] <= '9') {
postfix[j++] = infix[i];
} else if (infix[i] == '(') {
stack[0] = '(';
op = infix[i];
} else if (infix[i] == ')') {
while (stack[0] != '(') {
postfix[j++] = stack[0];
for (int k = 1; k < MAX_STACK_LEN && stack[k] != '\0'; k++) {
stack[k - 1] = stack[k];
}
stack[MAX_STACK_LEN - 1] = '\0';
}
op = stack[0];
for (int k = 1; k < MAX_STACK_LEN && stack[k] != '\0'; k++) {
stack[k - 1] = stack[k];
}
stack[MAX_STACK_LEN - 1] = '\0';
} else {
while (precedence(op) >= precedence(stack[0])) {
postfix[j++] = stack[0];
for (int k = 1; k < MAX_STACK_LEN && stack[k] != '\0'; k++) {
stack[k - 1] = stack[k];
}
stack[MAX_STACK_LEN - 1] = '\0';
}
op = infix[i];
stack[0] = op;
for (int k = MAX_STACK_LEN - 1; k > 0 && stack[k - 1] != '\0'; k--) {
stack[k] = stack[k - 1];
}
stack[0] = op;
}
}
while (stack[0] != '\0') {
postfix[j++] = stack[0];
for (int k = 1; k < MAX_STACK_LEN && stack[k] != '\0'; k++) {
stack[k - 1] = stack[k];
}
stack[MAX_STACK_LEN - 1] = '\0';
}
postfix[j] = '\0';
}
// 利用栈求解后缀表达式的值
int evaluate_postfix(char *postfix) {
int i, len, a, b, res;
char stack[MAX_STACK_LEN];
len = strlen(postfix);
for (i = 0; i < len; i++) {
if (postfix[i] >= '0' && postfix[i] <= '9') {
stack[0] = postfix[i];
a = atoi(stack);
for (int k = MAX_STACK_LEN - 1; k > 0 && stack[k - 1] != '\0'; k--) {
stack[k] = stack[k - 1];
}
stack[0] = '\0';
sprintf(stack, "%d", a);
} else {
b = atoi(stack);
for (int k = MAX_STACK_LEN - 1; k > 0 && stack[k - 1] != '\0'; k--) {
stack[k] = stack[k - 1];
}
stack[0] = '\0';
sprintf(stack, "%d", b);
a = atoi(stack);
for (int k = MAX_STACK_LEN - 1; k > 0 && stack[k - 1] != '\0'; k--) {
stack[k] = stack[k - 1];
}
stack[0] = '\0';
switch (postfix[i]) {
case '+':
res = a + b;
break;
case '-':
res = a - b;
break;
case '*':
res = a * b;
break;
case '/':
res = a / b;
break;
}
sprintf(stack, "%d", res);
}
}
return res;
}
int main() {
char infix[MAX_EXPR_LEN], postfix[MAX_EXPR_LEN];
int result;
strcpy(infix, "3*(7-2)");
infix_to_postfix(infix, postfix);
printf("Infix expression: %s\n", infix);
printf("Postfix expression: %s\n", postfix);
result = evaluate_postfix(postfix);
printf("Result: %d\n", result);
return 0;
}
```
运行以上代码,输出的结果为:
```
Infix expression: 3*(7-2)
Postfix expression: 372-*
Result: 15
```
其中,`infix_to_postfix` 函数用于将算数表达式转化为后缀表达式,`evaluate_postfix` 函数用于利用栈求解后缀表达式的值。在主函数中,先定义一个算数表达式并初始化为3*(7-2),然后调用 `infix_to_postfix` 函数将其转化为后缀表达式,再调用 `evaluate_postfix` 函数求解后缀表达式的值,最后输出计算结果。
相关推荐









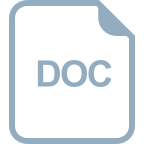
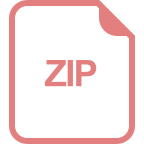
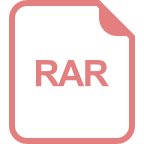