c语言open用法
时间: 2023-12-03 07:04:42 浏览: 32
在 C 语言中,可以使用 `open` 函数打开文件。`open` 函数的原型如下:
```c
#include <fcntl.h>
int open(const char *pathname, int flags, mode_t mode);
```
其中,`pathname` 参数是要打开的文件路径;`flags` 参数是打开文件的方式和选项,例如 `O_RDONLY` 表示以只读方式打开文件,`O_WRONLY` 表示以只写方式打开文件,`O_RDWR` 表示以读写方式打开文件等;`mode` 参数是要创建文件时的权限,通常使用 `0644` 表示普通文件的读写权限。
`open` 函数返回一个文件描述符,如果出现错误则返回 `-1`。可以使用这个文件描述符对文件进行读写操作,例如使用 `read` 函数和 `write` 函数进行读写。
下面是一个例子,演示如何使用 `open` 函数打开文件并进行读写操作:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
// 打开文件
int fd = open("test.txt", O_RDWR | O_CREAT, 0644);
if (fd == -1) {
perror("open");
return -1;
}
// 写入数据
const char *msg = "Hello, world!";
if (write(fd, msg, sizeof(msg)) == -1) {
perror("write");
return -1;
}
// 读取数据
char buf[1024];
if (read(fd, buf, sizeof(buf)) == -1) {
perror("read");
return -1;
}
// 输出数据
printf("%s\n", buf);
// 关闭文件
if (close(fd) == -1) {
perror("close");
return -1;
}
return 0;
}
```
在上面的例子中,使用 `open` 函数打开了一个名为 `test.txt` 的文件,并以读写方式打开。然后,使用 `write` 函数向文件中写入了一串字符串,再使用 `read` 函数从文件中读取数据,并使用 `printf` 函数输出读取到的数据。最后,使用 `close` 函数关闭文件。
相关推荐
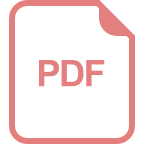
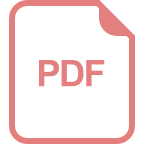
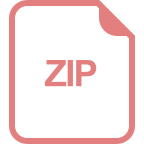
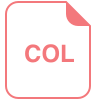
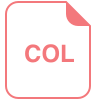
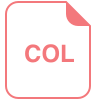
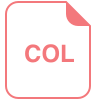
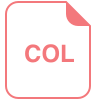









