使用C++写出用信号量解决经典同步问题的生产者消费者问题
时间: 2024-02-21 21:57:28 浏览: 86
以下是使用 C++11 的 std::thread 和 std::condition_variable 类实现的生产者消费者问题的解决方案,其中使用了两个信号量 empty 和 full 用来控制缓冲区的空槽数量和已经填充的数据数量:
```cpp
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <queue>
const int N = 10;
std::queue<int> buffer;
std::mutex m;
std::condition_variable not_full, not_empty;
int count = 0;
void producer()
{
while (true)
{
int item = rand() % 100;
{
std::unique_lock<std::mutex> lock(m);
not_full.wait(lock, [] { return count != N; });
buffer.push(item);
count++;
std::cout << "Produced item: " << item << ", count: " << count << std::endl;
}
not_empty.notify_one();
}
}
void consumer()
{
while (true)
{
int item;
{
std::unique_lock<std::mutex> lock(m);
not_empty.wait(lock, [] { return count != 0; });
item = buffer.front();
buffer.pop();
count--;
std::cout << "Consumed item: " << item << ", count: " << count << std::endl;
}
not_full.notify_one();
}
}
int main()
{
std::thread t1(producer);
std::thread t2(consumer);
t1.join();
t2.join();
return 0;
}
```
在生产者线程中,首先生成一个随机数作为生产的数据项,然后获取互斥锁,检查空槽数量是否为 0,如果为 0 则等待非满条件变量,否则将数据项放入缓冲区中,并将计数器加 1。在释放互斥锁之前,通过非空条件变量通知消费者线程可以从缓冲区中取出数据了。
在消费者线程中,首先获取互斥锁,检查缓冲区中是否有数据,如果没有则等待非空条件变量,否则将数据项从缓冲区中取出,并将计数器减 1。在释放互斥锁之前,通过非满条件变量通知生产者线程可以往缓冲区中放入数据了。
阅读全文
相关推荐



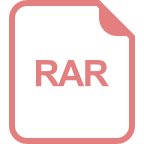
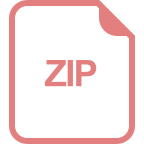












