asp.net使用C#基于cookie实现购物车
时间: 2024-06-08 10:11:54 浏览: 19
在 ASP.NET 中,可以使用 C# 代码基于 cookie 实现购物车功能,具体步骤如下:
1. 定义一个名为 "ShoppingCart" 的类,包含商品信息、数量、价格等属性。
```
public class ShoppingCartItem
{
public int ProductId { get; set; }
public string ProductName { get; set; }
public decimal Price { get; set; }
public int Quantity { get; set; }
}
```
2. 在添加商品到购物车时,将购物车信息保存到 cookie 中。
```
public void AddToCart(int productId, string productName, decimal price, int quantity)
{
// 读取购物车信息
List<ShoppingCartItem> cart = GetShoppingCart();
// 查找商品是否已经存在于购物车中
ShoppingCartItem item = cart.FirstOrDefault(i => i.ProductId == productId);
if (item == null)
{
// 商品不存在于购物车中,将其添加到购物车
item = new ShoppingCartItem
{
ProductId = productId,
ProductName = productName,
Price = price,
Quantity = quantity
};
cart.Add(item);
}
else
{
// 商品已经存在于购物车中,更新其数量
item.Quantity += quantity;
}
// 将购物车信息保存到 cookie 中
SaveShoppingCart(cart);
}
```
3. 从 cookie 中读取购物车信息。
```
public List<ShoppingCartItem> GetShoppingCart()
{
List<ShoppingCartItem> cart = new List<ShoppingCartItem>();
HttpCookie cookie = HttpContext.Current.Request.Cookies["ShoppingCart"];
if (cookie != null)
{
string json = HttpUtility.UrlDecode(cookie.Value);
cart = JsonConvert.DeserializeObject<List<ShoppingCartItem>>(json);
}
return cart;
}
```
4. 将购物车信息保存到 cookie 中。
```
public void SaveShoppingCart(List<ShoppingCartItem> cart)
{
string json = JsonConvert.SerializeObject(cart);
HttpCookie cookie = new HttpCookie("ShoppingCart", HttpUtility.UrlEncode(json));
HttpContext.Current.Response.Cookies.Add(cookie);
}
```
5. 在购物车页面中,读取购物车信息并显示出来。
```
List<ShoppingCartItem> cart = GetShoppingCart();
foreach (ShoppingCartItem item in cart)
{
// 显示商品信息、数量、价格等
}
```
注意,使用 cookie 实现购物车功能的缺点是,购物车信息存储在客户端,容易被篡改或删除。因此,对于重要的商业网站,建议使用服务器端的购物车实现方式。
相关推荐
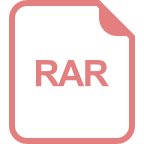














