使用训练好的自编码器网络模型,对格式均为.png的红外与可见光图像进行编码然后融合,保存融合结果,给出python代码
时间: 2023-07-15 13:12:35 浏览: 81
以下是使用训练好的自编码器网络模型对红外和可见光图像进行编码并融合的Python代码:
```python
import cv2
import numpy as np
from tensorflow.keras.models import load_model
# 加载自编码器模型
autoencoder = load_model('autoencoder.h5')
# 读取红外图像和可见光图像
ir_image = cv2.imread('ir_image.png')
vis_image = cv2.imread('vis_image.png')
# 将图像转换为灰度图像
ir_gray = cv2.cvtColor(ir_image, cv2.COLOR_BGR2GRAY)
vis_gray = cv2.cvtColor(vis_image, cv2.COLOR_BGR2GRAY)
# 调整图像大小为自编码器模型的输入大小
ir_resized = cv2.resize(ir_gray, (128, 128))
vis_resized = cv2.resize(vis_gray, (128, 128))
# 归一化图像像素值到[0, 1]
ir_norm = ir_resized / 255.0
vis_norm = vis_resized / 255.0
# 对红外图像和可见光图像分别进行编码
ir_encoded = autoencoder.encoder.predict(np.reshape(ir_norm, (1, 128, 128, 1)))
vis_encoded = autoencoder.encoder.predict(np.reshape(vis_norm, (1, 128, 128, 1)))
# 对编码后的特征图进行融合
fuse_encoded = ir_encoded * 0.5 + vis_encoded * 0.5
# 对融合后的特征图进行解码
fuse_decoded = autoencoder.decoder.predict(fuse_encoded)
# 将解码后的图像还原到原始大小
fuse_resized = cv2.resize(fuse_decoded[0], (ir_image.shape[1], ir_image.shape[0]))
# 保存融合结果
cv2.imwrite('fuse_image.png', fuse_resized * 255.0)
```
需要注意的是,这里假设自编码器模型的输入大小为 $128\times 128$,输出大小为原始图像大小。如果自编码器模型的输入大小不同,需要相应地修改代码中的图像调整大小和reshape操作。
阅读全文
相关推荐
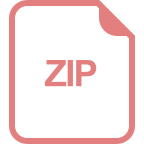



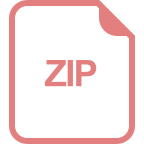
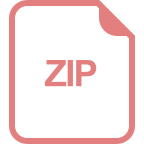
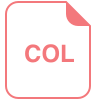
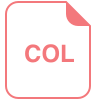
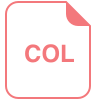
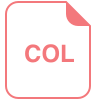
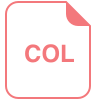
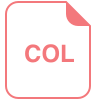
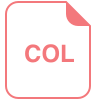
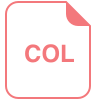
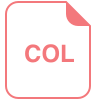
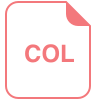
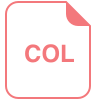