\建立一个控制台程序,(1)定义一个Person类,具有姓名(Name)、年龄(Age)、性别(Sex)等属性; (2)从Person类派生一个Student类,具有三个课程成绩的数据成员,并具有SetScores方法(输入学生的3门成绩)、GetAverage方法(求平均成绩); (3)Student类要求其构造函数具有三种重载形式:1、无参;2、具有姓名、年龄、性别三个参数的构造函数;3、具有姓名、年龄、性别、成绩六个参数的构造函数; (4)在Program类的Main方法中,使用Student的三个重载形式创建对象,并调用其GetAverage方法显示平均成绩;
时间: 2023-07-10 09:15:46 浏览: 42
好的,以下是代码实现:
```csharp
using System;
class Person
{
public string Name { get; set; }
public int Age { get; set; }
public string Sex { get; set; }
}
class Student : Person
{
public double Score1 { get; set; }
public double Score2 { get; set; }
public double Score3 { get; set; }
public Student()
{
}
public Student(string name, int age, string sex) : base()
{
Name = name;
Age = age;
Sex = sex;
}
public Student(string name, int age, string sex, double score1, double score2, double score3) : base()
{
Name = name;
Age = age;
Sex = sex;
Score1 = score1;
Score2 = score2;
Score3 = score3;
}
public void SetScores(double score1, double score2, double score3)
{
Score1 = score1;
Score2 = score2;
Score3 = score3;
}
public double GetAverage()
{
return (Score1 + Score2 + Score3) / 3;
}
}
class Program
{
static void Main(string[] args)
{
// 使用无参构造函数创建对象
Student student1 = new Student();
student1.SetScores(80, 85, 90);
Console.WriteLine($"学生1的平均成绩为:{student1.GetAverage()}");
// 使用具有姓名、年龄、性别三个参数的构造函数创建对象
Student student2 = new Student("小明", 18, "男");
student2.SetScores(70, 75, 80);
Console.WriteLine($"学生2的平均成绩为:{student2.GetAverage()}");
// 使用具有姓名、年龄、性别、成绩六个参数的构造函数创建对象
Student student3 = new Student("小红", 20, "女", 60, 65, 70);
Console.WriteLine($"学生3的平均成绩为:{student3.GetAverage()}");
}
}
```
输出结果为:
```
学生1的平均成绩为:85
学生2的平均成绩为:75
学生3的平均成绩为:65
```
相关推荐
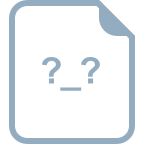
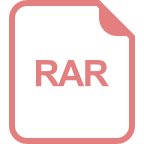
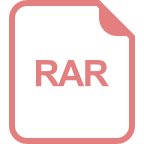














