MATLAB程序 imu与lidar位置卡尔曼滤波融合
时间: 2024-02-04 19:02:50 浏览: 25
以下是一个基本的MATLAB程序,用于使用卡尔曼滤波算法将IMU和LIDAR传感器的位置数据进行融合。
首先,我们需要定义一些变量和常数。这个程序使用的是2D空间,因此我们只需要定义x和y轴的位置和速度。我们还需要定义IMU和LIDAR传感器的位置噪声和测量噪声,以及卡尔曼滤波器的初始状态和协方差矩阵。
```
% Define variables and constants
dt = 0.01; % Time step
g = 9.81; % Acceleration due to gravity
% Define state variables
x = zeros(4,1); % [x, y, vx, vy]
A = [1 0 dt 0; 0 1 0 dt; 0 0 1 0; 0 0 0 1]; % State transition matrix
B = [0; 0; 0; -g*dt]; % Control input matrix
u = 0; % Control input (in this case, gravity)
% Define measurement variables and noise
z_imu = 0; % IMU measurement (position)
z_lidar = 0; % LIDAR measurement (position)
H_imu = [1 0 0 0; 0 1 0 0]; % IMU measurement matrix
H_lidar = [1 0 0 0; 0 1 0 0]; % LIDAR measurement matrix
R_imu = [0.01 0; 0 0.01]; % IMU measurement noise
R_lidar = [0.1 0; 0 0.1]; % LIDAR measurement noise
% Define initial state and covariance matrix
x0 = [0; 0; 0; 0]; % Initial state (position and velocity)
P0 = eye(4); % Initial covariance matrix
```
接下来,我们需要编写一个函数来执行卡尔曼滤波算法。该函数将采用当前的状态和协方差矩阵,以及IMU和LIDAR传感器的测量值和噪声,计算新的状态和协方差矩阵,并返回它们。
```
function [x_new, P_new] = kalman_filter(x, P, z_imu, z_lidar, R_imu, R_lidar, H_imu, H_lidar, A, B, u)
% Predict new state and covariance
x_pred = A*x + B*u; % Predicted state
P_pred = A*P*A' + eye(4); % Predicted covariance
% Update state and covariance using IMU measurement
K_imu = P_pred*H_imu'/(H_imu*P_pred*H_imu' + R_imu); % Kalman gain
x_imu = x_pred + K_imu*(z_imu - H_imu*x_pred); % Updated state
P_imu = (eye(4) - K_imu*H_imu)*P_pred; % Updated covariance
% Update state and covariance using LIDAR measurement
K_lidar = P_imu*H_lidar'/(H_lidar*P_imu*H_lidar' + R_lidar); % Kalman gain
x_new = x_imu + K_lidar*(z_lidar - H_lidar*x_imu); % Updated state
P_new = (eye(4) - K_lidar*H_lidar)*P_imu; % Updated covariance
end
```
最后,我们可以编写主程序来模拟IMU和LIDAR传感器的位置测量,并使用卡尔曼滤波器来融合它们。该程序将模拟一个物体从高空自由落体并最终落地的过程。
```
% Simulate freefall from 100 meters
t = 0:dt:10; % Time vector
x_true = zeros(4,length(t)); % True state (position and velocity)
x_true(2,:) = -0.5*g*t.^2; % True position (y-axis)
x_true(4,:) = -g*t; % True velocity (y-axis)
% Simulate IMU and LIDAR measurements
for i=1:length(t)
z_imu = x_true(2,i) + sqrt(R_imu(1,1))*randn; % IMU measurement (position)
z_lidar = x_true(2,i) + sqrt(R_lidar(1,1))*randn; % LIDAR measurement (position)
[x, P] = kalman_filter(x, P, z_imu, z_lidar, R_imu, R_lidar, H_imu, H_lidar, A, B, u); % Update state using Kalman filter
x_est(:,i) = x; % Estimated state (position and velocity)
end
% Plot results
figure;
subplot(2,1,1);
plot(t,x_true(2,:),t,x_est(2,:));
legend('True position','Estimated position');
xlabel('Time (s)');
ylabel('Position (m)');
subplot(2,1,2);
plot(t,x_true(4,:),t,x_est(4,:));
legend('True velocity','Estimated velocity');
xlabel('Time (s)');
ylabel('Velocity (m/s)');
```
这个程序将输出两个子图,一个显示物体的真实位置和Kalman滤波器估计的位置,另一个显示真实速度和估计速度。
注意,这个程序只是一个基本的示例,用于说明如何使用卡尔曼滤波器将IMU和LIDAR传感器的位置数据进行融合。在实际应用中,您可能需要调整卡尔曼滤波器的参数和传感器的噪声模型,以获得更准确的结果。
相关推荐
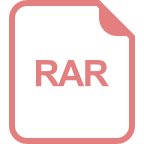
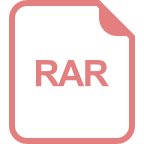




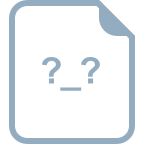
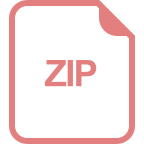
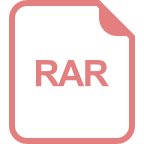
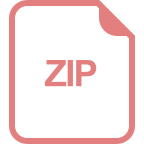
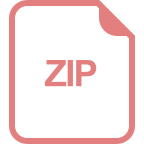
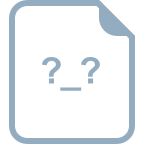