Task<HttpResponseMessage>”未包含“GetAwaiter”的定义,并且找不到可接受第一个“Task<HttpResponseMessage>”类型参数的可访问扩展方法“GetAwaiter”(是否缺少 using 指令或程序集引用?
时间: 2023-09-23 08:03:51 浏览: 81
这个错误通常是因为缺少 `System.Net.Http` 命名空间的引用。请确保在代码文件的顶部添加了以下引用语句:
```csharp
using System.Net.Http;
```
如果已经添加了这个引用,还是出现了这个错误,可能是因为你的项目没有正确地引用 `System.Net.Http` 程序集。你可以通过以下方法解决这个问题:
1. 右击项目,选择“管理 NuGet 程序包”
2. 在“浏览”选项卡下搜索“System.Net.Http”
3. 找到并安装最新版本的“System.Net.Http” NuGet 包
如果这些方法都不起作用,你可以尝试将以下代码添加到你的 `.csproj` 文件中:
```xml
<ItemGroup>
<PackageReference Include="System.Net.Http" Version="4.3.4" />
</ItemGroup>
```
然后重新生成你的项目。
相关问题
无法将类型System.Net.Http.HttpResponseMessage隐式转换为“System.Threading.Tasks.Task<System.Net.Http.HttpResponseMessage>”
这个错误提示表明你正在尝试将 `System.Net.Http.HttpResponseMessage` 类型隐式转换为 `System.Threading.Tasks.Task<System.Net.Http.HttpResponseMessage>` 类型,但这两个类型是不同的。
在异步编程中,通常我们会使用 `Task<T>` 类型来表示一个异步操作的结果,而不是直接返回结果类型。因此,你可能需要在某个地方使用 `async` 和 `await` 来处理异步操作。
如果你正在编写一个异步方法,并且希望返回一个 `HttpResponseMessage` 实例,可以将方法定义为 `async Task<HttpResponseMessage>`,并在方法体中使用 `await` 关键字来等待异步操作完成。
例如:
```csharp
public async Task<HttpResponseMessage> MyAsyncMethod()
{
// 异步操作...
HttpResponseMessage response = await SomeAsyncOperation();
// 返回结果
return response;
}
```
如果你只是想同步返回一个 `HttpResponseMessage` 实例,可以使用 `Task.FromResult` 方法将其包装成一个已完成的任务:
```csharp
public Task<HttpResponseMessage> MySyncMethod()
{
HttpResponseMessage response = SomeSyncOperation();
return Task.FromResult(response);
}
```
请根据具体的上下文和需求,选择适合的方式处理异步操作和任务返回类型。
优化下面得到代码,internal async Task<ResponseResult> PostDataAsync(string url, String param) { //await new MessageDialog(param, "parsm").ShowAsync(); Uri requestUri = new Uri(BaseLocalHost + $"{url}"); ResponseResult httpResponseBody = new ResponseResult(); string res = ""; HttpStringContent content = new HttpStringContent( param, Windows.Storage.Streams.UnicodeEncoding.Utf8, "application/json" ); try { HttpResponseMessage httpResponseMessage = await httpClient.PostAsync(requestUri, content); // await new MessageDialog(httpResponseMessage.StatusCode.ToString(), "响应状态").ShowAsync(); httpResponseMessage.EnsureSuccessStatusCode(); var res1 = await httpResponseMessage.Content.ReadAsStringAsync(); //res1 typeof string 我想要得到的是ResponseResult类型 //httpResponseBody = res1; #region 数据格式转换 var serializer = new DataContractJsonSerializer(typeof(ResponseResult)); var ms = new MemoryStream(Encoding.UTF8.GetBytes(res1)); httpResponseBody = serializer.ReadObject(ms) as ResponseResult; #endregion await new MessageDialog(httpResponseBody.message.ToString(),"响应体").ShowAsync(); return httpResponseBody; } catch (Exception ex) { res = "Error:" + ex.HResult.ToString("X") + "Message:" + ex.Message; await new MessageDialog(res, "网络请求失败").ShowAsync(); return httpResponseBody; } }
下面是对你提供的代码进行优化的建议:
```csharp
internal async Task<ResponseResult> PostDataAsync(string url, string param)
{
Uri requestUri = new Uri(BaseLocalHost + url);
ResponseResult httpResponseBody = new ResponseResult();
try
{
HttpStringContent content = new HttpStringContent(param, Windows.Storage.Streams.UnicodeEncoding.Utf8, "application/json");
HttpResponseMessage httpResponseMessage = await httpClient.PostAsync(requestUri, content);
httpResponseMessage.EnsureSuccessStatusCode();
string res1 = await httpResponseMessage.Content.ReadAsStringAsync();
httpResponseBody = ConvertToResponseResult(res1);
await new MessageDialog(httpResponseBody.message.ToString(), "响应体").ShowAsync();
return httpResponseBody;
}
catch (Exception ex)
{
string res = "Error:" + ex.HResult.ToString("X") + "Message:" + ex.Message;
await new MessageDialog(res, "网络请求失败").ShowAsync();
return httpResponseBody;
}
}
private ResponseResult ConvertToResponseResult(string json)
{
var serializer = new DataContractJsonSerializer(typeof(ResponseResult));
using (var ms = new MemoryStream(Encoding.UTF8.GetBytes(json)))
{
return serializer.ReadObject(ms) as ResponseResult;
}
}
```
优化说明:
1. 将参数类型由 `String` 改为 `string`,与 C# 的命名约定一致。
2. 将参数的初始化放在使用之前,避免不必要的初始化。
3. 将数据格式转换的代码抽取到一个单独的方法 `ConvertToResponseResult` 中,提高代码的可读性和复用性。
4. 使用 `using` 语句来确保资源的正确释放。
希望以上优化建议对你有帮助!如果你还有其他问题,请随时提问。
相关推荐
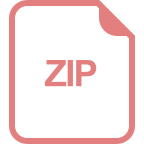
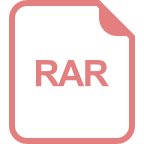
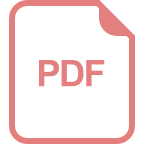












