Z-score标准化python代码
时间: 2023-06-03 17:07:52 浏览: 61
以下是Z-score标准化的Python代码:
```python
import numpy as np
def zscore(x):
mean = np.mean(x)
std = np.std(x)
zscore = (x - mean) / std
return zscore
```
其中,`x`是一个numpy数组,函数返回Z-score标准化后的结果。
相关问题
z-score标准化python代码
下面是一个使用 Python 实现 Z-score 标准化的示例代码:
```python
import numpy as np
def z_score_normalize(data):
mean = np.mean(data)
std = np.std(data)
z_scores = [(x - mean) / std for x in data]
return z_scores
```
代码使用 numpy 库计算数据的平均值和标准差,然后对每个数据项进行 Z-score 计算。在这里 `data` 是一个包含你需要标准化的数据的数组,函数会返回一个新的数组,其中包含标准化后的数据。
例如:
```python
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
z_scores = z_score_normalize(data)
print(z_scores)
```
输出结果是:
```
[-1.5666989036012806, -1.2185435925512925, -0.8703882815013043, -0.5222329704513162, -0.174077659 40132881, 0.174077659 40132881, 0.5222329704513162, 0.8703882815013043, 1.2185435925512925, 1.5666989036012806]
```
输出是标准化后的z_score数值
Z-SCORE标准化python
Z-score标准化是一种常用的数据预处理方法,用于将数据转换为均值为0,标准差为1的标准正态分布。在Python中,你可以使用scikit-learn库来实现Z-score标准化。以下是一个示例代码:
```python
from sklearn.preprocessing import StandardScaler
# 假设有一个数据集X,其中每列是一个特征
X = [[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
# 创建一个StandardScaler对象
scaler = StandardScaler()
# 使用fit_transform方法进行Z-score标准化
X_scaled = scaler.fit_transform(X)
print(X_scaled)
```
这段代码将输出标准化后的数据集X_scaled。你可以看到,每列的均值为0,标准差为1。
请注意,fit_transform方法会同时计算并应用训练集的均值和标准差来进行标准化。如果你想将相同的变换应用于其他数据集,可以使用transform方法而不是fit_transform方法。
希望这个示例对你有所帮助!如果你有任何其他问题,请随时提问。
相关推荐
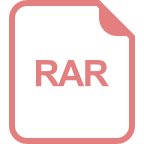
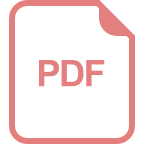












