Micropython 蓝牙库
时间: 2023-09-18 22:12:53 浏览: 59
Micropython 蓝牙库是用于在 Micropython 环境下实现与蓝牙设备通信的库。它提供了一组 API,可以用于搜索、连接、读写和断开连接蓝牙设备。以下是 Micropython 蓝牙库的一些常用功能:
1. 扫描附近的蓝牙设备
2. 连接到一个蓝牙设备
3. 读取和写入蓝牙设备的属性值
4. 监听蓝牙设备的通知
5. 断开与蓝牙设备的连接
Micropython 蓝牙库支持多种蓝牙协议和配置文件,例如 BLE、SPP、A2DP、AVRCP 等。它可以在 Micropython 的各种开发板上使用,例如 ESP32、ESP8266、pyboard 等。
相关问题
micropython 蓝牙
MicroPython提供了许多蓝牙库,可以帮助你在MicroPython设备上使用蓝牙。下面是一些常用的库和示例:
1. `ubluetooth` 库:这个库提供了访问蓝牙低功耗(BLE)的接口,可以在MicroPython设备上实现BLE通信。以下是一个简单的示例:
```python
import ubluetooth
bt = ubluetooth.BLE()
# 开启蓝牙
bt.active(True)
# 扫描周围的BLE设备
bt.gap_scan(2000)
# 获取扫描结果
devices = bt.get_advertisements()
# 连接设备
for device in devices:
if device.addr == '00:11:22:33:44:55':
conn = bt.gap_connect(device.addr)
```
2. `bluetooth` 库:这个库提供了访问经典蓝牙的接口,可以在MicroPython设备上实现经典蓝牙通信。以下是一个简单的示例:
```python
import bluetooth
# 开始搜索周围的蓝牙设备
devices = bluetooth.discover_devices()
# 连接设备
for device in devices:
if device == '00:11:22:33:44:55':
sock = bluetooth.BluetoothSocket(bluetooth.RFCOMM)
sock.connect((device, 1))
```
以上示例只是简单的入门示例,你可以根据自己的需要进行更高级的蓝牙开发。
micropython 蓝牙键盘
Micropython可以通过使用内置的`bluetooth`库来实现蓝牙功能。要将Micropython设备设置为蓝牙键盘,您需要使用`bluetooth`库中的`HIDDevice`类来模拟HID设备。以下是一个简单的示例代码,可以将Micropython设备设置为蓝牙键盘并发送按键事件:
```python
import bluetooth
from micropython import const
# 定义HID键盘的一些常量
KEY_LEFT_CTRL = const(0x01)
KEY_LEFT_SHIFT = const(0x02)
KEY_A = const(0x04)
KEY_B = const(0x05)
KEY_C = const(0x06)
# 定义HID消息类型的常量
REPORT_MAP = bytes((
0x05, 0x01, # Usage Page (Generic Desktop Ctrls)
0x09, 0x06, # Usage (Keyboard)
0xA1, 0x01, # Collection (Application)
0x85, 0x01, # Report ID (1)
0x05, 0x07, # Usage Page (Key Codes)
0x19, 0xE0, # Usage Minimum (224)
0x29, 0xE7, # Usage Maximum (231)
0x15, 0x00, # Logical Minimum (0)
0x25, 0x01, # Logical Maximum (1)
0x75, 0x01, # Report Size (1)
0x95, 0x08, # Report Count (8)
0x81, 0x02, # Input (Data,Var,Abs,No Wrap,Linear,Preferred State,No Null Position)
0x95, 0x01, # Report Count (1)
0x75, 0x08, # Report Size (8)
0x81, 0x01, # Input (Const,Array,Abs,No Wrap,Linear,Preferred State,No Null Position)
0x95, 0x05, # Report Count (5)
0x75, 0x01, # Report Size (1)
0x05, 0x08, # Usage Page (LEDs)
0x19, 0x01, # Usage Minimum (1)
0x29, 0x05, # Usage Maximum (5)
0x91, 0x02, # Output (Data,Var,Abs,No Wrap,Linear,Preferred State,No Null Position,Non-volatile)
0x95, 0x01, # Report Count (1)
0x75, 0x03, # Report Size (3)
0x91, 0x01, # Output (Const,Array,Abs,No Wrap,Linear,Preferred State,No Null Position,Non-volatile)
0x95, 0x06, # Report Count (6)
0x75, 0x08, # Report Size (8)
0x15, 0x00, # Logical Minimum (0)
0x25, 0x65, # Logical Maximum (101)
0x05, 0x07, # Usage Page (Key Codes)
0x19, 0x00, # Usage Minimum (0)
0x29, 0x65, # Usage Maximum (101)
0x81, 0x00, # Input (Data,Array,Abs,No Wrap,Linear,Preferred State,No Null Position)
0xC0, # End Collection
))
# 定义HID设备类
class HIDDevice:
def __init__(self, psm=17, report_map=REPORT_MAP):
self.report_map = report_map
self.psm = psm
self.sdp_record = None
self.advertising = False
self.socket = None
# 创建并绑定蓝牙socket
def bind(self):
self.socket = bluetooth.BluetoothSocket(bluetooth.L2CAP)
self.socket.bind(("", self.psm))
# 开始广告
def advertise(self, name="HID"):
self.sdp_record = bluetooth.SDPRecord.create(
0x10001,
{
0x0100: name,
0x0001: self.report_map,
},
)
bluetooth.advertise_service(
self.socket,
name=name,
service_id=self.sdp_record.service_id,
service_classes=[bluetooth.SERIAL_PORT_CLASS],
profiles=[bluetooth.SERIAL_PORT_PROFILE],
# protocol=bluetooth.L2CAP,
)
self.advertising = True
# 停止广告
def stop_advertising(self):
bluetooth.stop_advertising(self.socket)
self.advertising = False
# 发送按键事件
def send_key(self, key):
data = bytearray(8)
data[0] = KEY_LEFT_CTRL
data[2] = key
self.socket.send(bytes(data))
# 模拟按键组合
def send_key_combination(self, key1, key2):
data = bytearray(8)
data[0] = KEY_LEFT_CTRL | KEY_LEFT_SHIFT
data[2] = key1
data[3] = key2
self.socket.send(bytes(data))
# 创建一个HID设备实例并开始广告
hid_device = HIDDevice()
hid_device.bind()
hid_device.advertise()
# 发送按键事件
hid_device.send_key(KEY_A)
```
请注意,此示例仅演示了如何将Micropython设备设置为蓝牙键盘,并发送按键事件。您需要根据自己的需求修改代码,并实现其他功能,例如模拟按键组合、发送多个按键事件等。
相关推荐
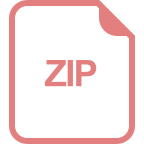
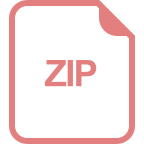












