解释每一句heihei = butter(order, [low, high], btype='bandpass', analog=False, output='ba', fs=None) b=heihei[0] a=heihei[1] print('b=', b) print('a=', a)
时间: 2023-11-22 20:52:35 浏览: 23
这段代码是用于滤波的,解释如下:
1. `heihei = butter(order, [low, high], btype='bandpass', analog=False, output='ba', fs=None)`: 这一行代码调用了 `butter` 函数来生成一个巴特沃斯(Butterworth)滤波器。其中 `order` 是滤波器的阶数,`[low, high]` 是滤波器的截止频率,`btype='bandpass'` 表示滤波器是带通滤波器,`analog=False` 表示滤波器是数字滤波器,`output='ba'` 表示返回滤波器的分子和分母系数,`fs=None` 表示采样率未知。
2. `b=heihei[0]`:`heihei` 是一个长度为 2 的元组,分别存储了滤波器的分子和分母系数。这一行代码将分子系数赋值给变量 `b`。
3. `a=heihei[1]`:同上,这一行代码将分母系数赋值给变量 `a`。
4. `print('b=', b)` 和 `print('a=', a)`:这两行代码分别输出滤波器的分子和分母系数,以便查看滤波器的具体参数。
相关问题
写一个飞机大战的cocos代码
由于涉及到游戏逻辑和图形渲染等方面,编写一个完整的飞机大战游戏需要涉及大量的代码。以下是一个简单的示例,可以作为参考:
1. 创建一个场景
```cpp
auto scene = Scene::create();
auto layer = Layer::create();
scene->addChild(layer);
```
2. 加载游戏资源
```cpp
auto spriteCache = SpriteFrameCache::getInstance();
spriteCache->addSpriteFramesWithFile("resources.plist");
```
3. 创建玩家飞机
```cpp
auto player = Sprite::createWithSpriteFrameName("player.png");
player->setPosition(Vec2(visibleSize.width/2, visibleSize.height/4));
layer->addChild(player);
```
4. 创建敌机
```cpp
auto enemy = Sprite::createWithSpriteFrameName("enemy.png");
float randomX = RandomHelper::random_real(0, visibleSize.width);
enemy->setPosition(Vec2(randomX, visibleSize.height));
layer->addChild(enemy);
```
5. 处理玩家飞机移动
```cpp
auto move = MoveTo::create(0.5f, Vec2(touch->getLocation().x, player->getPosition().y));
player->runAction(move);
```
6. 处理敌机移动和碰撞检测
```cpp
auto move = MoveBy::create(2.0f, Vec2(0, -visibleSize.height));
enemy->runAction(move);
if (player->getBoundingBox().intersectsRect(enemy->getBoundingBox())) {
// 碰撞发生,处理游戏结束逻辑
}
```
7. 处理子弹发射和移动
```cpp
auto bullet = Sprite::createWithSpriteFrameName("bullet.png");
bullet->setPosition(player->getPosition());
layer->addChild(bullet);
auto move = MoveBy::create(1.0f, Vec2(0, visibleSize.height));
bullet->runAction(move);
```
8. 处理游戏结束
```cpp
auto gameOverLabel = Label::createWithTTF("Game Over", "fonts/Marker Felt.ttf", 48);
gameOverLabel->setPosition(Vec2(visibleSize.width/2, visibleSize.height/2));
layer->addChild(gameOverLabel);
```
以上只是一个简单的示例,实际上还需要处理音效、分数统计、关卡设定等方面,需要更加完整的代码实现。
make: *** No rule to make target 'main.c', needed by 'heihei'. Stop.是为什么
这个错误提示表示在当前目录下没有找到名为 "main.c" 的文件,但是您的编译命令却需要这个文件来进行编译,所以编译过程无法继续进行,最终导致了编译失败。
您需要确认以下几点:
1. 确认当前目录下是否存在名为 "main.c" 的文件。
2. 确认您的编译命令中是否正确指定了要编译的源文件名。
3. 确认您的编译环境是否正常,例如编译器是否正确安装等。
如果以上三个方面都没有问题,您还可以尝试重新生成 Makefile 文件,或者检查 Makefile 文件中是否正确指定了源文件名。
相关推荐
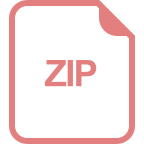











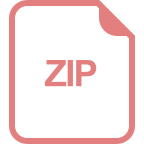