用python写函数,返回一个扑克牌列表,里面有52项,每一项都是一个tuple。 例如:[(“♥”,“2”), (“♣”,“2”), (“♠”,”K”), (“♦”,“A”),………………….] poke_types = ["♥", "♠", "♣", "♦"] poke_nums = [2, 3, 4, 5, 6, 7, 8, 9, 10, "J", "Q", "K", "A"] 写一函数,判定三张扑克牌是否是同花? 写一函数,判定三张扑克牌是否是豹子? 写一函数,判定三张扑克牌是否是同花顺?
时间: 2023-08-01 18:11:28 浏览: 184
生成扑克牌列表的函数如下:
```python
import itertools
def generate_poke_list():
poke_types = ["♥", "♠", "♣", "♦"]
poke_nums = [2, 3, 4, 5, 6, 7, 8, 9, 10, "J", "Q", "K", "A"]
poke_list = list(itertools.product(poke_types, poke_nums))
return poke_list
```
判断三张扑克牌是否是同花的函数如下:
```python
def is_same_suit(poke1, poke2, poke3):
if poke1[0] == poke2[0] == poke3[0]:
return True
else:
return False
```
判断三张扑克牌是否是豹子的函数如下:
```python
def is_triplet(poke1, poke2, poke3):
if poke1[1] == poke2[1] == poke3[1]:
return True
else:
return False
```
判断三张扑克牌是否是同花顺的函数如下:
```python
def is_straight_flush(poke1, poke2, poke3):
poke_nums = ["A", 2, 3, 4, 5, 6, 7, 8, 9, 10, "J", "Q", "K", "A"]
poke_num_list = [poke1[1], poke2[1], poke3[1]]
poke_num_list.sort(key=lambda x: poke_nums.index(x))
if is_same_suit(poke1, poke2, poke3) and poke_num_list == poke_nums[poke_nums.index(poke_num_list[0]):poke_nums.index(poke_num_list[0])+3]:
return True
else:
return False
```
阅读全文
相关推荐
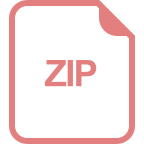
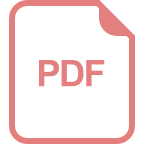

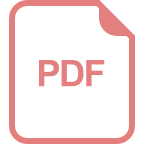
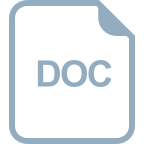
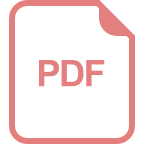
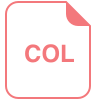
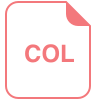
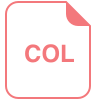
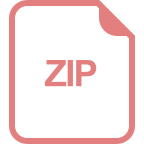
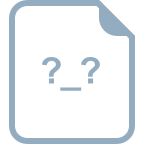
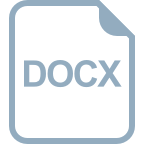
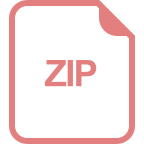
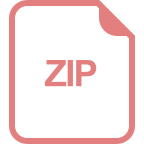
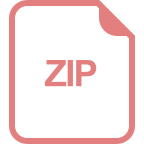