【用Python讲算法故事】
发布时间: 2024-09-01 06:14:37 阅读量: 244 订阅数: 96 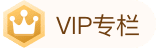
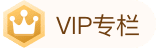
# 1. Python与算法的亲密关系
## 1.1 算法的定义与重要性
算法是一系列解决问题的指令集合,它们在计算机科学中占据核心地位。Python作为一门高级编程语言,以其简洁明了的语法和强大的库支持,在算法的实现和应用上具有独特的优势。算法对于Python而言,就像是烹饪的食谱对于厨师的重要性。无论是复杂的数据处理还是日常的开发任务,算法都是达成目标的必经之路。
## 1.2 Python在算法开发中的应用
在实际的算法开发中,Python凭借其简洁的语法和丰富的标准库,简化了算法的实现过程。它支持快速原型开发,使得开发者可以快速地验证算法想法,并转化为实际的程序代码。Python还广泛应用于数据科学、机器学习和网络开发等众多领域,这使得Python与算法之间的亲密关系更进一步。
## 1.3 算法优化与Python的性能
虽然Python被誉为“解释型”语言,运行效率不如编译型语言,但Python 3在性能上的提升已经足以满足大多数算法应用的需求。此外,Python通过C语言扩展、JIT技术(如PyPy)以及并行计算库(如multiprocessing和threading)等多种方式,实现了算法优化和性能提升。在追求算法效率的同时,Python始终保持了其代码的可读性和开发的敏捷性。
# 2.1 基本数据结构
### 2.1.1 列表和元组的操作
列表(List)和元组(Tuple)是Python中最基本的数据结构之一,它们都是序列数据类型,能够存储一系列元素。列表是可变的,意味着列表中的元素可以随时进行添加、删除和修改;而元组是不可变的,一旦创建后不能进行修改。
#### 列表操作
列表的创建非常简单,直接使用方括号`[]`或者`list()`函数进行创建:
```python
# 方括号创建列表
my_list = [1, 2, 3, 'Python']
# 使用list()函数转换序列到列表
another_list = list((4, 5, 6))
```
列表的操作包括但不限于添加、删除、排序、索引、切片等:
```python
# 添加元素
my_list.append(4)
my_list.extend([7, 8])
# 删除元素
my_list.remove(1)
del my_list[1]
# 排序列表
my_list.sort()
my_list.reverse()
# 访问元素
element = my_list[0]
# 列表切片
slice_of_list = my_list[1:3]
```
列表的一个典型应用场景是数组的扩展和修改。
#### 元组操作
元组的创建同样简单,使用圆括号`()`,或者直接使用逗号分隔值(不加括号):
```python
# 圆括号创建元组
my_tuple = (1, 2, 3, 'Python')
# 逗号分隔值创建元组
another_tuple = 4, 5, 6
```
元组的操作相对较少,因为它不可变,所以不能添加、删除或修改元素:
```python
# 访问元素
element = my_tuple[0]
# 元组切片
slice_of_tuple = my_tuple[1:3]
```
元组的典型应用场景是存储一组不可变的数据。
### 2.1.2 字典和集合的应用
#### 字典操作
字典(Dict)是一种可变容器模型,存储键值对。在Python中,字典被写为花括号`{}`内部的一组键值对,每个键和值用冒号`:`分隔,键必须是唯一的。
```python
# 创建字典
my_dict = {'name': 'Alice', 'age': 25, 'email': '***'}
```
字典操作包括但不限于添加、删除、访问、修改等:
```python
# 添加或修改键值对
my_dict['address'] = 'New York'
# 删除键值对
del my_dict['email']
# 访问键值对
value = my_dict['name']
# 获取所有键
keys = my_dict.keys()
# 获取所有值
values = my_dict.values()
# 获取所有键值对
items = my_dict.items()
```
字典的典型应用场景是实现哈希表和存储键值映射关系。
#### 集合操作
集合(Set)是一个无序的不重复元素序列。集合使用大括号`{}`创建,或者使用`set()`函数。
```python
# 创建集合
my_set = {1, 2, 3, 'Python'}
```
集合操作包括但不限于添加、删除、并集、交集等:
```python
# 添加元素
my_set.add(4)
# 删除元素
my_set.remove('Python')
# 并集
union_set = my_set.union({3, 4, 5})
# 交集
intersection_set = my_set.intersection({3, 4})
# 集合的成员检测
is_element = 1 in my_set
```
集合的典型应用场景是进行元素去重和集合运算。
#### 数据结构的对比
列表、元组、字典和集合各自有不同的特点和适用场景,它们在内存占用、操作速度和功能上各有利弊。如下表展示了这些基本数据结构的主要特性对比:
| 数据结构 | 可变性 | 有序性 | 元素唯一性 |
| -------- | ------ | ------ | ---------- |
| 列表 | 可变 | 有序 | 不唯一 |
| 元组 | 不可变 | 有序 | 不唯一 |
| 字典 | 可变 | 无序 | 不唯一 |
| 集合 | 可变 | 无序 | 唯一 |
通过理解列表、元组、字典和集合的基本操作和特性,开发者可以选择最合适的数据结构进行编程,以优化代码的效率和可读性。
# 3. Python中的经典算法
## 3.1 排序算法
排序算法是计算机科学中的基本问题之一,它们在数据处理、搜索优化和其他算法领域中有着广泛的应用。Python 作为一门高级编程语言,内置了多种排序机制,但理解不同排序算法的原理对于提升程序性能至关重要。
### 3.1.1 冒泡排序、选择排序与插入排序
冒泡排序(Bubble Sort)、选择排序(Selection Sort)和插入排序(Insertion Sort)是最基础的三种排序算法,它们虽然在实际应用中效率不高,但因为其实现简单,常作为教学示例。
#### 冒泡排序
冒泡排序的基本思想是通过重复遍历要排序的数列,一次比较两个元素,如果顺序错误就把它们交换过来。遍历数列的工作是重复进行直到没有再需要交换,也就是说该数列已经排序完成。
```python
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
```
这段代码通过双层循环完成冒泡排序。外层循环控制排序趟数,内层循环负责每趟的比较和交换。每完成一趟排序,最大的元素就“浮”到了数组的末尾,因此下一趟就不需要再比较这个元素。
#### 选择排序
选择排序的原理是首先在未排序序列中找到最小(或最大)元素,存放到排序序列的起始位置,然后,再从剩余未排序元素中继续寻找最小(或最大)元素,然后放到已排序序列的末尾。以此类推,直到所有元素均排序完毕。
```python
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_idx = i
for j in range(i+1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
```
选择排序的时间复杂度为 O(n^2),但不需要像冒泡排序那样进行多余的交换操作,因此在某些情况下效率更高。
#### 插入排序
插入排序的工作方式很像我们在打扑克牌时,把抓到的牌插入到已整理好牌序的一堆牌中。具体而言,它的工作原理是通过构建有序序列,对于未排序数据,在已排序序列中从后向前扫描,找到相应位置并插入。
```python
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i-1
```
0
0
相关推荐
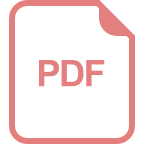
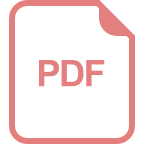
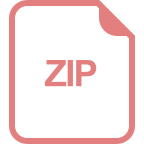





