【Python算法可视化扩展应用】
发布时间: 2024-09-01 05:56:10 阅读量: 421 订阅数: 96 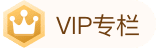
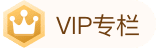
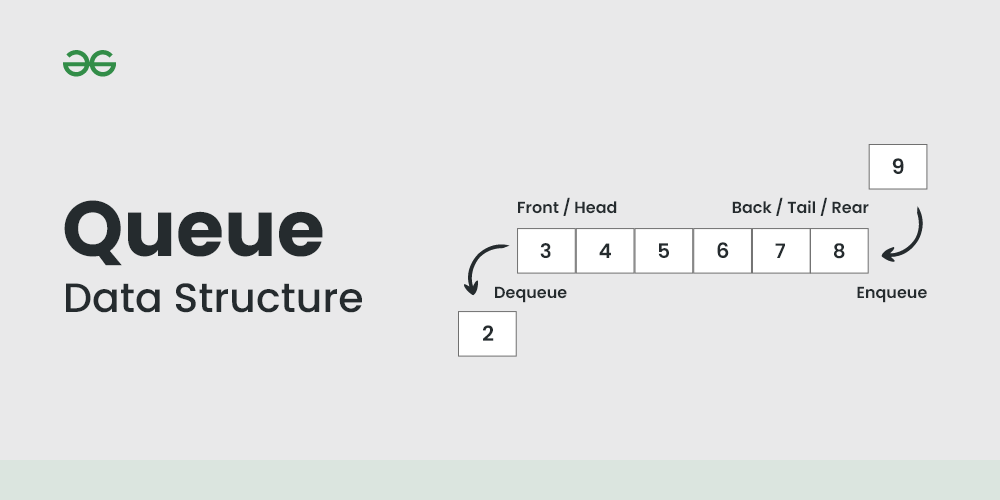
# 1. Python算法可视化的基础介绍
在信息技术飞速发展的当下,数据的可视化已成为解释复杂数据和模型的关键手段,尤其是在算法教学和研究中,它让抽象的概念具体化,帮助人们直观理解算法的运行机制。Python算法可视化是一个将算法执行过程通过图形的方式表现出来的过程,使得算法执行的每一步都可视化,便于观察其内部逻辑和数据变化,极大地增强了算法学习和调试的直观性。
可视化的过程涉及到数据处理、图形绘制和用户交互等多个环节,Python作为一门强大的编程语言,其丰富的库资源为算法可视化提供了强大的支持。使用Python进行算法可视化,不仅可以提升编程能力,还能增强对算法行为和性能的深入理解。
本章将介绍算法可视化的基础概念,概述其在数据分析、教育、研究等领域中的应用价值,并为后续章节的深入探讨打下基础。我们将从算法可视化的意义讲起,逐步过渡到可视化的关键技术要素,为读者提供一个全面的入门指导。
# 2. Python算法可视化的核心组件
## 2.1 可视化库的选择与使用
### 2.1.1 Matplotlib基础
Matplotlib是Python中最流行的2D绘图库之一,提供了丰富的API来创建各种静态、动态和交互式的图形。对于算法可视化而言,Matplotlib能以图形化的方式展现算法的执行过程,帮助我们理解和分析算法的运行状态。
Matplotlib由John Hunter在2003年创立,目的是为了模仿MATLAB的绘图功能。它的设计灵感来源于MATLAB,因此对于使用过MATLAB的用户来说,上手会非常容易。Matplotlib可以创建线图、直方图、条形图、散点图、饼图等各类统计图表。
创建一个基本的Matplotlib图表的代码示例如下:
```python
import matplotlib.pyplot as plt
# 假设我们有以下数据
x = [0, 1, 2, 3, 4, 5]
y = [0, 1, 4, 9, 16, 25]
# 使用plot函数绘制线图
plt.plot(x, y)
# 添加标题和标签
plt.title('Square numbers')
plt.xlabel('Value of x')
plt.ylabel('Square of x')
# 显示图表
plt.show()
```
以上代码段绘制了一个简单的一次函数图像。在算法可视化中,我们更关注动态效果,因此在实际应用中往往需要利用`FuncAnimation`或`animate`函数来实现图形的动态更新,从而达到可视化算法执行过程的目的。
```python
import numpy as np
from matplotlib import animation, pyplot as plt
# 初始化图表和轴
fig, ax = plt.subplots()
xdata, ydata = [], []
ln, = plt.plot([], [], 'ro')
def init():
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
return ln,
def update(frame):
xdata.append(frame)
ydata.append(np.sin(frame))
ln.set_data(xdata, ydata)
return ln,
# 创建动画
ani = animation.FuncAnimation(fig, update, frames=np.linspace(0, 2*np.pi, 128),
init_func=init, blit=True)
plt.show()
```
这段代码通过绘制正弦函数,模拟了动态的图形更新。在算法可视化中,通过类似的机制,我们可以实时展示算法操作数据的每一步。
### 2.1.2 Seaborn与其他可视化工具
虽然Matplotlib是一个功能强大的绘图库,但在处理复杂数据时,有时候我们需要更为高级的绘图工具。Seaborn是建立在Matplotlib基础上的一个统计绘图库,它提供了一系列高级绘图工具,可以轻松地创建更加美观的图表。
Seaborn内置了许多预设的主题和颜色方案,使图表美观且易于阅读。Seaborn还支持一些复杂的数据可视化任务,如绘制回归线、分类图、分组统计图等。通过Seaborn的“pairplot”、“heatmap”等函数,我们可以方便地实现数据的可视化分析。
此外,还有许多其他的Python可视化库,如Plotly、Bokeh等,它们提供了不同的功能和用途。Plotly支持创建交互式的图表,可以嵌入到网页中。Bokeh则擅长处理大规模数据集的实时可视化。选择合适的可视化库,可以帮助我们更有效地表达算法可视化的需求。
### 2.1.3 绘图工具的比较
我们可以通过下面的表格对Matplotlib、Seaborn、Plotly和Bokeh这四种常用的绘图工具进行比较,从而更好地选择适合我们需求的工具:
| 特性 | Matplotlib | Seaborn | Plotly | Bokeh |
|---|---|---|---|---|
| 功能丰富度 | 低 | 高 | 高 | 低 |
| 交互性 | 低 | 低 | 高 | 高 |
| 适用场景 | 静态图表 | 统计图表 | 交互式图表 | 实时数据可视化 |
| 性能 | 中 | 中 | 高 | 高 |
| Web集成 | 低 | 低 | 高 | 高 |
选择合适的可视化工具将依赖于项目的具体需求。例如,如果你需要创建一个复杂的统计图表,Seaborn可能是更好的选择。如果需要交互式的Web图表,Plotly和Bokeh将是更合适的选择。
## 2.2 数据结构在可视化中的应用
### 2.2.1 列表和字典的可视化
在Python中,列表(list)和字典(dict)是最常用的数据结构。列表是一种有序集合,而字典是一种映射类型。在可视化时,我们经常需要将这些数据结构以图形的方式展现出来,以便观察其内部的结构和关系。
#### 列表的可视化
列表可以被用来存储任何类型的数据,比如数字、字符串甚至是其他列表。列表的可视化通常涉及展示列表中的元素以及它们之间的位置关系。
```python
import matplotlib.pyplot as plt
# 示例列表
my_list = [3, 8, 1, 10, 15, 4]
# 绘制条形图
plt.bar(range(len(my_list)), my_list)
plt.xlabel('List Index')
plt.ylabel('Value')
plt.title('List Visualization')
plt.show()
```
以上代码将列表`my_list`的每个元素显示为条形图中的一个条形。图表的横轴表示列表索引,纵轴表示值。
#### 字典的可视化
字典由键值对组成,是Python中的一种映射类型。在可视化字典时,我们通常关注键和值的关系。比如,我们可以通过散点图来可视化字典中键和值之间的对应关系。
```python
import matplotlib.pyplot as plt
# 示例字典
my_dict = {'apple': 3, 'banana': 8, 'cherry': 1, 'date': 10}
# 提取键和值
keys = list(my_dict.keys())
values = list(my_dict.values())
# 绘制散点图
plt.scatter(keys, values)
plt.xlabel('Key')
plt.ylabel('Value')
plt.title('Dictionary Visualization')
plt.show()
```
在这段代码中,我们将字典的键值对绘制为散点图。横轴代表字典的键,纵轴代表对应的值。这使得我们能够快速地可视化字典中存储的数据的关系。
### 2.2.2 高维数据的降维与可视化技巧
在算法可视化中,高维数据的处理是一个挑战。高维数据包含了多个属性或特征,人类的直观理解能力在面对高维数据时会显得力不从心。因此,降维技术在数据可视化中显得尤为重要。
#### 主成分分析(PCA)
PCA是一种常用的数据降维方法,它通过正交变换将一组可能相关的变量转换成一组线性不相关的变量,转换后的变量称为主成分。主成分是原始数据的线性组合,且第一主成分具有最大的方差,第二主成分具有次大的方差,依此类推。
我们可以使用Python中的`sklearn.decomposition`模块进行PCA操作,并可视化结果。
```python
from sklearn.decomposition import PCA
import matplotlib.pyplot as plt
# 示例三维数据
data = [[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]]
# 创建PCA实例,降维到2维
pca = PCA(n_components=2)
reduced_data = pca.fit_transform(data)
# 绘制降维后的数据
plt.scatter(reduced_data[:, 0], reduced_data[:, 1])
plt.xlabel('Principal Component 1')
plt.ylabel('Principal Component 2')
plt.title('PCA Visualization of High-dimensional Data')
plt.show()
```
通过PCA降维,我们可以将三维数据降维到二维,然后通过散点图的方式展示降维后的数据点。这有助于我们从视觉上理解数据的分布和结构。
#### t-SNE
t-SNE是一种非常流行的降维技术,它在处理高维数据的可视化方面尤为出色。t-SNE是一种非线性降维方法,特别适合于将高维数据降至二维或三维以便可视化。
使用Python中的`sklearn.manifold`模块中的t-SNE进行数据降维,代码示例如下:
```python
from sklearn.manifold import TSNE
import matplotlib.pyplot as plt
# 示例数据
X = [[0, 0, 0], [0, 1, 1], [1, 0, 1], [1, 1, 1]]
# 创建t-SNE实例
tsne = TSNE(n_components=2, random_state=0)
reduced_data = tsne.fit_transform(X)
# 绘制降维后的数据
plt.scatter(reduced_data[:, 0], reduced_data[:, 1])
plt.xlabel('t-SNE Feature 1')
plt.ylabel('t-SNE Feature 2')
plt.title('t-SNE Visualization of High-dimensional Data')
plt.show()
```
t-SNE的可视化结果通常更符合人类的直观理解,尤其是在数据聚类方面表现突出。它能够发现并保持原始高维数据中的结构,使得降维后的数据分布依然有实际意义。
降维技术的运用,使得原本不可视的高维数据能够被简化到二维或三维空间进行展示,这是算法可视化中不可或缺的一个步骤。
## 2.3 算法可视化的交互性提升
### 2.3.1 交互式图表的创建与管理
交互式图表增加了用户与图表之间的互动,用户可以通过点击、拖动和缩放等操作来探索数据。Matplotlib和Seaborn这些静态图表库虽然提供了绘图功能,但交互性较弱。因此,为了实现强大的交互式图表,我们会使用如Plotly或Bokeh这样的库。
#### Plotly的交互式图表
Plotly是一个用于创建交互式图表的Python库。它不仅支持在浏览器中显示交互式图表,还可以嵌入到Jupyter Notebook中。Plotly图表支持多种交互功能,例如:缩放、拖动、悬停提示等。
```python
import plotly.graph_objs as go
from plotly.offline import plot
# 创建数据
trace0 = go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name='trace 0')
trace1 = go.Scatter(x=[20, 30, 40], y=[50, 60, 70], name='trace 1')
# 组合数据为图集
data = [trace0, trace1]
# 创建布局
layout = go.Layout(
title='Interactive Plot with Plotly'
)
# 创建图形对象
fig = go.Figure(data=data, layout=layout)
# 显示图形
plot(fig, filen
```
0
0
相关推荐
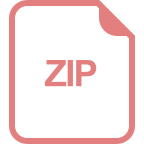
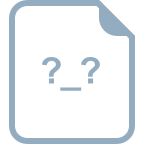
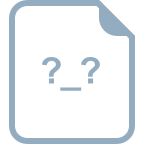
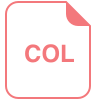
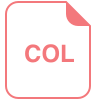
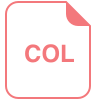
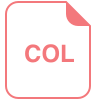

